jquery sets box size
In front-end web development, page layout design is a very important task. Among them, the box is the basis of page layout, and its size and style are crucial to the overall effect and user experience of the page. This article will introduce how to use jQuery to set the box size to achieve adaptive and fixed-size effects.
1. jQuery gets the box size
Before setting the box size, you first need to get the box size. jQuery provides multiple methods to obtain the size of the box, including width(), height(), outerWidth(), outerHeight(), innerWidth(), innerHeight(), etc.
- width() and height() methods
The width() and height() methods are used to obtain the width and height of the element respectively. These two methods return the width and height of the element's content area, excluding padding, border and margin.
Sample code:
$(document).ready(function() { var boxWidth = $('#box').width(); var boxHeight = $('#box').height(); console.log('boxWidth:' + boxWidth + ', boxHeight:' + boxHeight); });
- outerWidth() and outerHeight() methods
outerWidth() and outerHeight() methods are used to obtain the elements respectively outer width and outer height. These two methods return the total width and height of the element's content area plus padding, border, and margin.
Sample code:
$(document).ready(function() { var boxOuterWidth = $('#box').outerWidth(); var boxOuterHeight = $('#box').outerHeight(); console.log('boxOuterWidth:' + boxOuterWidth + ', boxOuterHeight:' + boxOuterHeight); });
- innerWidth() and innerHeight() methods
innerWidth() and innerHeight() methods are used to obtain the elements respectively inner width and inner height. These two methods return the total width and height of the element's content area plus padding, excluding border and margin.
Sample code:
$(document).ready(function() { var boxInnerWidth = $('#box').innerWidth(); var boxInnerHeight = $('#box').innerHeight(); console.log('boxInnerWidth:' + boxInnerWidth + ', boxInnerHeight:' + boxInnerHeight); });
2. Set the box size with jQuery
After getting the box size, you can use jQuery to dynamically set the box size. jQuery provides a variety of methods to set the box size, including width(), height(), outerWidth(), outerHeight(), innerWidth(), innerHeight(), etc.
- width() and height() methods
The width() and height() methods are used to set the width and height of elements respectively. Both methods accept a numeric parameter that specifies the width and height of the element. You can also use percentages as parameters to achieve adaptive effects.
Sample code:
$(document).ready(function() { // 设置固定宽度和高度 $('#box').width(200).height(100); // 设置自适应宽度和高度 $('#box').width('50%').height('50%'); });
- outerWidth() and outerHeight() methods
outerWidth() and outerHeight() methods are used to set the elements respectively outer width and outer height. These two methods accept a Boolean parameter to specify whether to include padding, border, and margin. You can also use percentages as parameters to achieve adaptive effects.
Sample code:
$(document).ready(function() { // 设置固定外宽度和外高度 $('#box').outerWidth(300, true).outerHeight(150, true); // 设置自适应外宽度和外高度 $('#box').outerWidth('50%', true).outerHeight('50%', true); });
- innerWidth() and innerHeight() methods
innerWidth() and innerHeight() methods are used to set the element respectively inner width and inner height. Both methods accept a numeric parameter that specifies the inner width and height of the element. You can also use percentages as parameters to achieve adaptive effects.
Sample code:
$(document).ready(function() { // 设置固定内宽度和内高度 $('#box').innerWidth(260).innerHeight(110); // 设置自适应内宽度和内高度 $('#box').innerWidth('50%').innerHeight('50%'); });
3. Implementation of adaptive and fixed sizes
In actual development, different page layouts require different box size setting methods. Generally speaking, adaptive sizes are suitable for responsive layouts, while fixed sizes are suitable for static layouts. The following describes how to use jQuery to achieve adaptive and fixed size effects.
- Implementation of adaptive size
Adaptive size usually uses percentages as parameters to implement responsive layout based on the browser window size.
Sample code:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>自适应大小的实现</title> <style> body { margin: 0; padding: 0; } #box { background-color: #ccc; width: 50%; height: 50%; margin: 0 auto; } </style> </head> <body> <div id="box"></div> <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script> $(document).ready(function() { $(window).resize(function() { $('#box').innerWidth('50%').innerHeight('50%'); }); }); </script> </body> </html>
In the above code, the css style is used to set the size of the box, and the innerWidth() and innerHeight() methods are used in jQuery to achieve adaptive size. When the browser window size changes, jQuery will detect the change in window size and recalculate the size of the box.
- Implementation of fixed size
Fixed size usually uses pixel values as parameters to implement static layout based on the design draft.
Sample code:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>固定大小的实现</title> <style> body { margin: 0; padding: 0; } #box { background-color: #ccc; width: 300px; height: 150px; margin: 0 auto; } </style> </head> <body> <div id="box"></div> <script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script> $(document).ready(function() { $('#box').width(200).height(100); }); </script> </body> </html>
In the above code, CSS styles are used to set the size of the box, and the width() and height() methods are used in jQuery to achieve a fixed size. In jQuery's ready event, set the size of the box to 200px×100px.
To sum up, this article introduces how to use jQuery to set the box size, including getting the box size and setting the box size, and how to achieve adaptive and fixed-size effects. I hope this article will be helpful to beginners in front-end web development.
The above is the detailed content of jquery sets box size. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


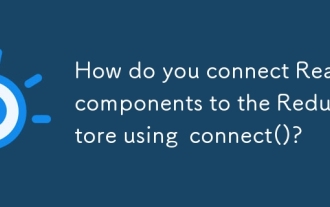
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
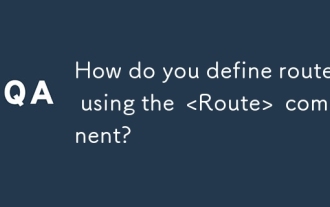
The article discusses defining routes in React Router using the <Route> component, covering props like path, component, render, children, exact, and nested routing.
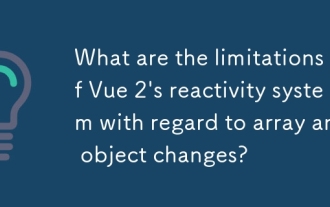
Vue 2's reactivity system struggles with direct array index setting, length modification, and object property addition/deletion. Developers can use Vue's mutation methods and Vue.set() to ensure reactivity.
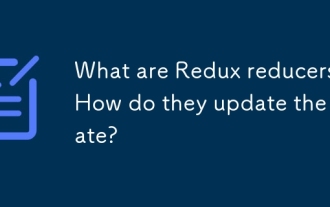
Redux reducers are pure functions that update the application's state based on actions, ensuring predictability and immutability.
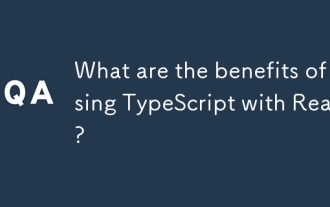
TypeScript enhances React development by providing type safety, improving code quality, and offering better IDE support, thus reducing errors and improving maintainability.
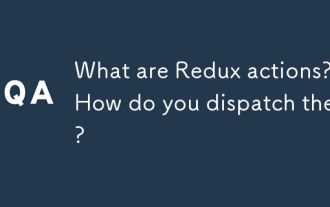
The article discusses Redux actions, their structure, and dispatching methods, including asynchronous actions using Redux Thunk. It emphasizes best practices for managing action types to maintain scalable and maintainable applications.
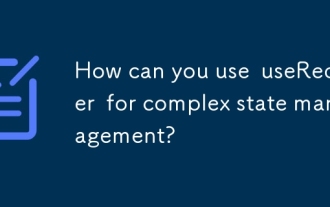
The article explains using useReducer for complex state management in React, detailing its benefits over useState and how to integrate it with useEffect for side effects.
