nodejs login jump page jump page jump
nodejs login jump page jump page jump
Node.js is a JavaScript running environment based on the Chrome V8 engine. It allows JavaScript to run on the server side, realizing the concept of a unified front-end and back-end development language. Node.js is widely used in web development, especially playing an important role in login functions and page jumps. This article will combine code implementation to introduce in detail the knowledge related to Node.js login and page jump.
1. Login function implementation
When using Node.js to implement the login function, we need to pay attention to the following points:
1) When using the HTTP protocol to transmit data, in order to To prevent the password from being transmitted in clear text, the HTTPS protocol needs to be used.
2) The front end sends the user name and password entered by the user to the back end through an AJAX request.
3) The backend needs to pass verification to determine whether the username and password are correct. If the verification is passed, set session to save the user login status.
The following is a sample code to implement the login function:
Back-end code:
const express = require('express') const bodyParser = require('body-parser') const session = require('express-session') const app = express() // 配置 body-parser,用于解析 post 请求体 app.use(bodyParser.urlencoded({ extended: false })) // 配置 express-session,用于保存 session app.use(session({ secret: 'keyboard cat', cookie: { maxAge: 60000 } })) // 登录接口 app.post('/login', (req, res) => { const username = req.body.username const password = req.body.password // TODO: 验证用户名和密码是否正确,这里省略代码... // 设置 session req.session.username = username // 返回登录成功信息 res.status(200).send('登录成功!') }) app.listen(3000, () => { console.log('Server is running on port 3000') })
Front-end code:
<form> <input type="text" name="username"> <input type="password" name="password"> <button type="button" onclick="login()">登录</button> </form> <script> function login() { // 获取用户名和密码 const username = document.getElementsByName('username')[0].value const password = document.getElementsByName('password')[0].value // 发送登录请求 const xhr = new XMLHttpRequest() xhr.open('POST', '/login', true) xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded') xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { alert(xhr.responseText) } } xhr.send(`username=${username}&password=${password}`) } </script>
2. Page jump implementation
After the user successfully logs in, we need to jump the user to the page after the successful login. The key to achieving page jump is to redirect the user's request to the specified URL. Node.js provides the res.redirect() method to implement page jump. The following is a sample code to implement page jump:
// 需要登录才能访问的页面 app.get('/secret', (req, res) => { // 如果用户未登录,重定向到登录页 if (!req.session.username) { res.redirect('/login.html') return } // 如果用户已登录,返回页面内容 res.send('这是一篇需要登录才能访问的文章。') })
In the above code, we use req.session to determine whether the user is logged in. If the user is not logged in, use res.redirect() to redirect to the login page.
In addition, in Node.js, access to static files (such as HTML, CSS, JS and other files) requires the use of express.static() middleware. Place the static files in the public directory, and then use the following code to start the application:
app.use(express.static('public')) app.listen(3000, () => { console.log('Server is running on port 3000') })
So users can access http://localhost:3000/login.html and http://localhost:3000/secret Wait for the address to access static files and dynamic pages.
Summary
Implementing login and page jump functions in Node.js requires verification, session, redirection and other technologies. Among them, verifying whether the user's username and password are correct is the key to realizing the login function; using session to save the user's login status to determine whether the user has logged in in subsequent requests; using the res.redirect() method to implement page jump. In actual development, appropriate improvements and improvements need to be made according to specific needs.
The above is the detailed content of nodejs login jump page jump page jump. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
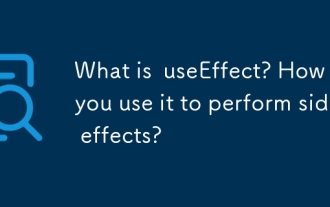
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
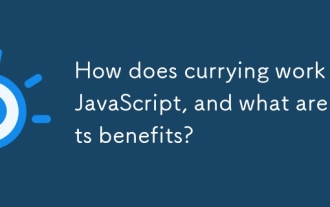
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
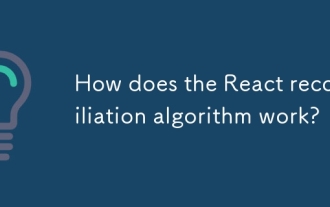
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
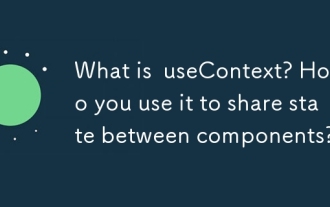
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
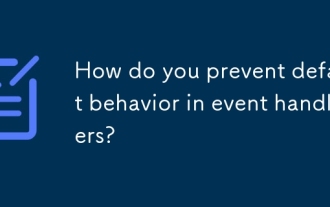
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
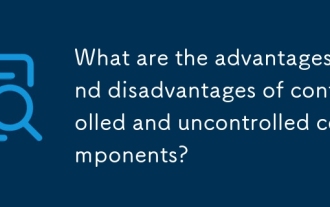
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
