Nodejs implements short links
With the development of the Internet, short links have become a very popular form of links. Short links can not only shorten the link length, but also beautify the link and increase user click-through rate. This article will introduce how to implement short links with Node.js.
- What is a short link
A short link is actually a string of characters, corresponding to a long link. The advantage of short links is that they can reduce link length, beautify links and increase user click-through rates.
Usually, short link services are provided by a third party, which generates a short link based on the user's long link and then redirects to the original long link address. There are many such services, such as Bitly, TinyURL, etc.
- Why use Node.js to implement short links
Node.js is an open source, cross-platform JavaScript running environment that allows JavaScript to run on the server side. Compared with other server-side languages, the biggest advantage of Node.js is its high concurrency and lightweight, which can quickly respond to user requests and handle very large concurrent requests.
Another major advantage of Node.js is the NPM package manager, which has a large number of lightweight modules available, which greatly reduces development complexity and improves development efficiency.
Therefore, using Node.js to implement short links can not only respond to requests quickly, but also easily use the NPM package manager to manage dependencies.
- Implementation steps
This article will implement the short link according to the following steps:
- Generate short code
- Storage short link Mapping relationship between code and long link
- Parse short link and redirect
3.1 Generate short code
The core of generating short code is to use a unique long link Takes an integer value as input and converts it into a string. Obviously, the input long integer needs to be large enough, otherwise the number of short codes will be very limited.
In the specific implementation, you can use alphabet and numbers to encode, and convert the long integer value into an arbitrary string, such as 32-base or 62-base.
The following is a sample code to generate a short code:
const alphabet = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'; const base = alphabet.length; function encode(num) { let encoded = ''; while (num) { const remainder = num % base; num = Math.floor(num / base); encoded = alphabet[remainder].toString() + encoded; } return encoded; }
The above code generates a function that can represent a 62-digit string. For an input integer value, this function converts it into a 62-digit string through continuous remainder method, and finally returns the generated string.
3.2 Store the mapping relationship between short code and long link
In the above, we have realized the generation of short code, and the next step is to store the mapping relationship between short code and long link. Here we choose to use Redis as the storage database.
Redis is an efficient key-value storage database written in C language. It can quickly read and write data. It also supports cluster construction and is very suitable for use as data storage for high-concurrency applications.
The following is a sample code for using Redis to store mapping relationships in Node.js:
const redis = require('redis'); const client = redis.createClient({ host: 'localhost', port: 6379 }); function saveUrl(url, callback) { client.incr('short:id', function(err, id) { if (err || !id) { return callback(err); } const shortUrl = encode(id); client.set(`short:${shortUrl}`, url, (err) => { if (err) { return callback(err); } callback(null, shortUrl); }); }); }
In the above code, we first use the incr
command to obtain a unique value from Redis Auto-incremented id. Then, encode that id into a shortcode and use the set
command to store the shortcode and long link mapping to Redis.
3.3 Parse short links and redirect
Finally, we implement a route that handles requests to parse short links and redirect users to the long link address.
The following is a sample code to implement the redirection function in Node.js:
const http = require('http'); const url = require('url'); http.createServer(function (req, res) { const shortUrl = url.parse(req.url).pathname.slice(1); client.get(`short:${shortUrl}`, function (err, url) { if (err) { return console.error(err); } if (!url) { res.writeHead(404); return res.end('404 Not Found'); } res.writeHead(301, { Location: url }); res.end(); }); }).listen(8080, () => console.log('Listening on port 8080...'));
Through the above code, we use http.createServer
to create an HttpServer instance, and The url
module is used in the callback function to resolve the requested connection.
Then, we obtain the original link corresponding to the short link from Redis, and use res.writeHead
to redirect to the original link address.
Of course, we must also consider the validity exception of the short link. For example, if the short link is not found in the database, a 404 error should be returned.
- Summary
This article focuses on how to use Node.js to implement short links, starting from generating short links, storing the mapping relationship between short codes and long links, and parsing short links. The three aspects of redirection elaborate on its implementation steps.
Here we use Redis to implement data storage, implement redirection through simple routing and http interaction, and use nodejs to work with Redis to create an efficient and pleasant development experience, allowing us to quickly Implement short link service.
The above is the detailed content of Nodejs implements short links. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


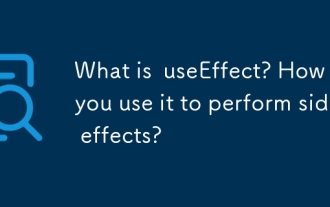
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
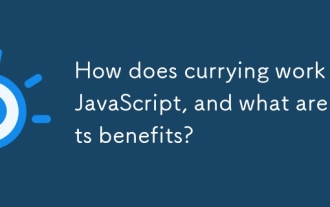
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
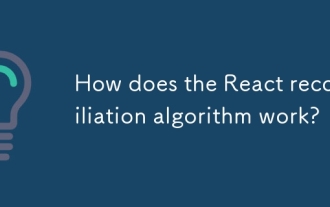
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
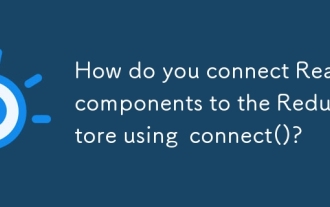
Article discusses connecting React components to Redux store using connect(), explaining mapStateToProps, mapDispatchToProps, and performance impacts.
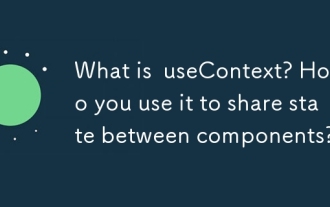
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
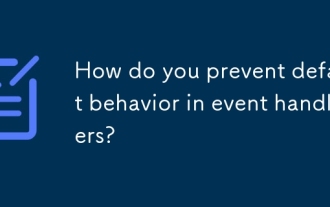
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
