jquery event implementation sequence
In front-end development, we often use jQuery to operate DOM elements and add various interactive effects to them, which is inseparable from the use of jQuery events. When using jQuery events, the order in which events are fired is very important, because the firing of one event may affect the execution of subsequent events. Therefore, this article will introduce the implementation sequence of jQuery events, hoping to help everyone better use jQuery events.
1. Event Binding
Before using jQuery events, you first need to bind events. There are two common methods of event binding:
- Binding events through selectors
Code example:
$(selector).on(event,function)
This method can be done by selecting Use the handler to select DOM elements and bind events to the selected elements. For example:
$("button").on("click",function(){ alert("您点击了按钮"); })
This code will select all
- Bind events directly
Code example:
$(dom).on(event,function)
This method can directly bind events to DOM elements without a selector. For example:
var btn = document.getElementById("btn"); $(btn).on("click",function(){ alert("您点击了按钮"); })
This code binds a click event to a button with the ID "btn".
2. Event triggering sequence
After binding the event, when the event is triggered, jQuery will execute the event in a certain order. The specific event triggering sequence is as follows:
- Capture phase
Before the event bubbles up to the target element, jQuery will first traverse down the top of the DOM tree, looking for The event target is related to the node and executes the event handler associated with it. This process is called the "capture phase".
- Target event execution
When the event target is found, jQuery will execute the event processing function related to it.
- Bubble stage
After executing the event handler of the target element, jQuery will bubble up along the DOM tree and execute the parent elements related to the event one by one. event handling function. This process is called the "bubbling phase".
It should be noted that jQuery events can use the event.stopPropagation() method to prevent the event from bubbling, and the event.preventDefault() method can also be used to cancel the default behavior of the event.
3. Event delegation
Event delegation is also a very common technique when using jQuery events. Through event delegation, you can bind event handlers to a parent element, thereby avoiding binding the same event handler to each child element. For example:
$("#parent").on("click","button",function(){ alert("您点击了按钮"); })
This code binds a click event to a parent element with the ID "parent". When a
In event delegation, the event will be triggered on the parent element, and then jQuery will traverse the child elements under the parent element to find the node related to the event target and execute the event handling function related to it. Therefore, when using event delegation, the order in which events are fired also follows the order described above.
4. Summary
This article introduces the implementation sequence of jQuery events, including event binding, event triggering sequence and event delegation. When using jQuery events, we need to pay attention to the order in which events are triggered to ensure correct execution of events. I hope this article can be helpful to everyone in their work in front-end development.
The above is the detailed content of jquery event implementation sequence. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
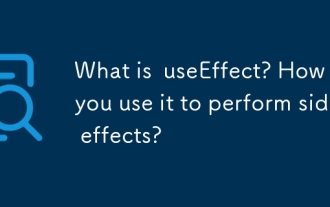
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
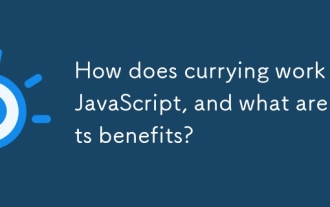
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
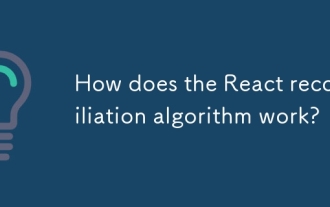
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
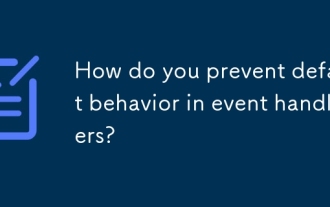
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
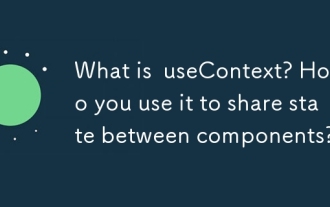
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
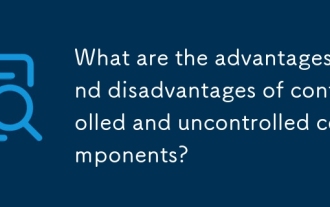
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
