php set get method call
The set and get methods in PHP are a common way to access private properties in object-oriented programming. The set method is used to set the value of a private property, while the get method is used to obtain the value of a private property. This article will introduce how to write set and get methods in PHP and demonstrate how to call these methods.
1. What are the set and get methods
The set and get methods are commonly used ways to access private properties in PHP. Private attributes are attributes defined in a class that can only be accessed by methods of the class and cannot be directly accessed or modified by external programs. This is a protection mechanism that makes the implementation details of a class invisible to the outside world and can only be accessed and modified through public methods defined within the class.
The set and get methods are usually used to set and get the value of private properties. The set method is used to set the value of a private property, usually with one parameter, the property value to be set, and does not return any value. The get method is used to obtain the value of a private property without parameters, but will return the value of the private property.
2. How to write set and get methods
In PHP, writing set and get methods is very simple. Normally, we need to define private properties in the class and write corresponding set and get methods in the class. The following example code shows how to write a class that contains private properties and corresponding set and get methods:
class Person { private $name; // set方法 public function setName($name) { $this->name = $name; } // get方法 public function getName() { return $this->name; } }
In this example, we define a Person class and define a private property $name. We also wrote two methods: the setName method is used to set the value of $name, and the getName method is used to obtain the value of $name.
In the setName method, we use the $this keyword to refer to the current object (the $this keyword in PHP refers to referencing the current object, similar to this in Java or self in C). We can access the private properties of this class through the $this keyword.
In the getName method, we only need to return the value of $name. We do not use the $this keyword here because the $get method does not need to modify the private properties of the class.
3. How to call the set and get methods
In PHP, we can call the methods defined in the class by instantiating the object. The following is a sample code that calls the set and get methods in the Person class:
// 实例化Person类 $person = new Person(); // 调用setName方法设置$name的值 $person->setName('张三'); // 调用getName方法获取$name的值 echo $person->getName();
In this example, we first instantiate the Person class and assign it to the $person variable. Next, we called the setName method of the $person object and set the value of $name to "Zhang San". Finally, we use the echo statement to output the result returned by the getName method of the $person object.
In PHP, we can call methods in a class through $object name->method name. When we call a method on an object, PHP will automatically pass the reference of the current object to the method. We do not need to explicitly pass the object reference when calling the method.
4. Conclusion
The set and get methods are common ways to access private properties in PHP object-oriented programming. Normally, we can define private properties in the class and write corresponding set and get methods to access and modify the private properties.
In PHP, we can call methods defined in a class by instantiating objects. When we call a method on an object, PHP will automatically pass the reference of the current object to the method. We do not need to explicitly pass the object reference when calling the method.
When writing classes, remember good encapsulation principles: encapsulate implementation details inside the class and provide a public interface for interacting with other parts of the code. In this way, we can maintain the code more easily and better protect the security of the related code.
The above is the detailed content of php set get method call. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
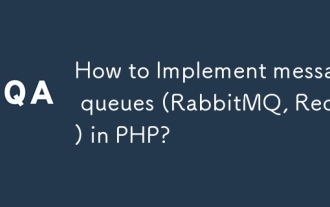
This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
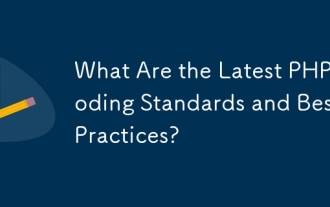
This article examines current PHP coding standards and best practices, focusing on PSR recommendations (PSR-1, PSR-2, PSR-4, PSR-12). It emphasizes improving code readability and maintainability through consistent styling, meaningful naming, and eff
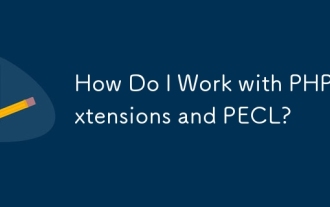
This article details installing and troubleshooting PHP extensions, focusing on PECL. It covers installation steps (finding, downloading/compiling, enabling, restarting the server), troubleshooting techniques (checking logs, verifying installation,
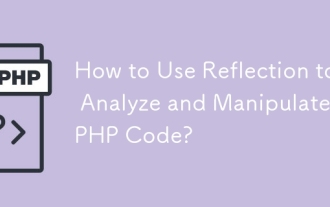
This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
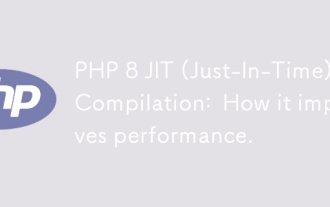
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
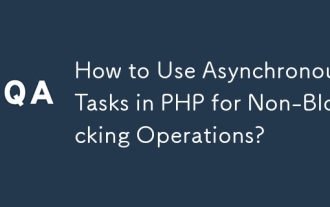
This article explores asynchronous task execution in PHP to enhance web application responsiveness. It details methods like message queues, asynchronous frameworks (ReactPHP, Swoole), and background processes, emphasizing best practices for efficien
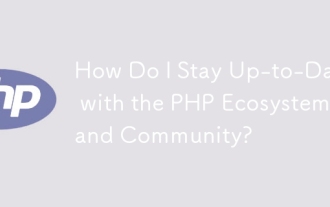
This article explores strategies for staying current in the PHP ecosystem. It emphasizes utilizing official channels, community forums, conferences, and open-source contributions. The author highlights best resources for learning new features and a
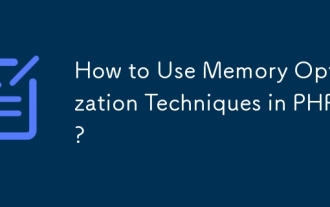
This article addresses PHP memory optimization. It details techniques like using appropriate data structures, avoiding unnecessary object creation, and employing efficient algorithms. Common memory leak sources (e.g., unclosed connections, global v
