php query stored procedure
In PHP, sometimes it is necessary to call stored procedures in the database to complete specific operations. In this case, you need to use PHP's database extension integration to interact with the database. This article will introduce how to query stored procedures in PHP.
Step 1: Connect to the database
First you need to make sure that you have correctly connected to the database. Connecting to the database can be done using the mysqli extension in PHP. After the connection is successful, you need to select a specific database. This can be achieved by calling the mysqli_select_db() function. The following is an example:
$username = "yourusername"; $password = "yourpassword"; $hostname = "localhost"; $database = "yourdatabase"; $connection = mysqli_connect($hostname, $username, $password); mysqli_select_db($connection, $database);
In the above code, the $username and $password variables store the username and password of the database respectively. The $hostname variable stores the hostname of the database. The $database variable stores the name of the database. Use the mysqli_connect() function when connecting to the database and store the result in the $connection variable. Then use the mysqli_select_db() function to select the database.
Step 2: Query the stored procedure
When querying the stored procedure in PHP, you need to use the mysqli_prepare() function to prepare the query and store the result in a variable. Use the CALL command in the query statement to call the stored procedure. For example:
$userid = 1; $stmt = mysqli_prepare($connection, "CALL get_user_by_id(?)"); mysqli_stmt_bind_param($stmt, "i", $userid); mysqli_stmt_execute($stmt);
In the above sample code, the $stmt variable stores the results of the query. The mysqli_prepare function is used to prepare query statements, where? is a placeholder representing the parameter value specified later. The actual parameter value can be specified through the mysqli_stmt_bind_param() function. The first parameter "i" is a format specifier, indicating an integer type, and the subsequent parameter $userid is the parameter value to be passed. Finally, use the mysqli_stmt_execute() function to execute the query.
Step 3: Obtain the query results
To obtain the query results of the stored procedure, you need to use the mysqli_stmt_store_result() function to save the results in the cache area. Then use the mysqli_stmt_bind_result() function to bind the result into a variable for use in your code. The following is an example:
mysqli_stmt_store_result($stmt); mysqli_stmt_bind_result($stmt, $col1, $col2, $col3); while (mysqli_stmt_fetch($stmt)) { printf("%s %s %s ", $col1, $col2, $col3); } mysqli_stmt_close($stmt); mysqli_close($connection);
In the above sample code, the mysqli_stmt_store_result() function is used to save the result in the cache area. Then use the mysqli_stmt_bind_result() function to bind the results to variables such as $col1, $col2, $col3, etc. for use in the loop. Use the mysqli_stmt_fetch() function in a loop to traverse the result set and output the query results. Finally, use the mysqli_stmt_close() function to close the query statement and use the mysqli_close() function to close the database connection.
Summary
Querying stored procedures is a way to implement specific database operations in PHP. When making a query, you first need to make sure you are connected to the database. Then, use the mysqli_prepare() function to prepare the query and the mysqli_stmt_bind_param() function to specify the parameters. Use the mysqli_stmt_execute() function to execute the query. Then, use the mysqli_stmt_store_result() function to save the result in the buffer area, and use the mysqli_stmt_bind_result() function to bind the result to a variable. Finally, use a while loop to traverse the result set and use the mysqli_stmt_fetch() function to output the query results. After completing the query, you need to use the mysqli_stmt_close() function to close the query statement and the mysqli_close() function to close the database connection.
The above is the detailed content of php query stored procedure. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


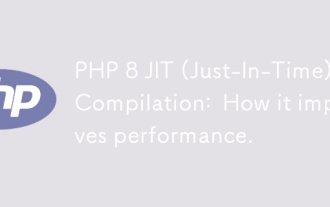
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
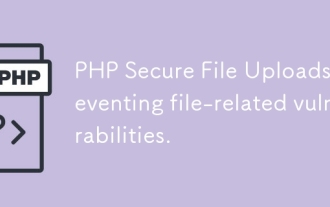
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
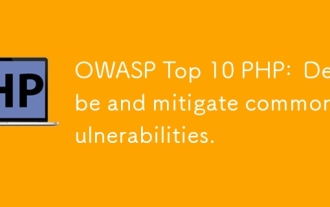
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
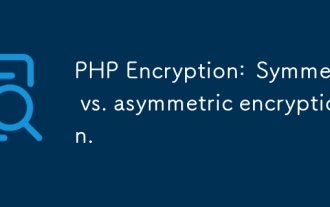
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
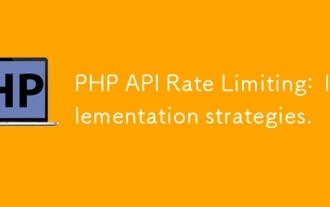
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
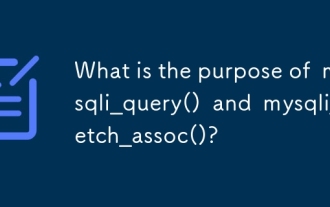
The article discusses the mysqli_query() and mysqli_fetch_assoc() functions in PHP for MySQL database interactions. It explains their roles, differences, and provides a practical example of their use. The main argument focuses on the benefits of usin
