laravel framework login registration process
The Laravel framework is an excellent and popular web application development framework based on the PHP language. The Laravel framework has a very complete user login and registration system. This registration and login system can implement basic authentication functions for users, and can perform a series of authorization operations on users, such as: user rights management, password reset, email verification, etc. . In the following article, we will elaborate on the login and registration process of the Laravel framework.
- User registration process
First, we need to create a user table to save the user's basic information. We can create this model using the "make:model" command provided by Laravel. Enter the following command in the terminal:
php artisan make:model User -m
This command will generate a User
model in the app
directory and a users# in the database. ##surface. In the
User model, we need to specify the authentication method used by the user. The Laravel framework provides a variety of user authentication methods, which we can set in the
guard attribute in the model:
protected $guard = 'web';
php artisan make:controller AuthRegisterController --resource
in the appHttpControllersAuth
directory. RegisterController.php file. In this controller, we need to implement the following method:
use AppUser; use IlluminateFoundationAuthRegistersUsers; public function register(Request $request) { $this->validator($request->all())->validate(); event(new Registered($user = $this->create($request->all()))); $this->guard()->login($user); return redirect($this->redirectTo); } // 验证用户输入的数据 protected function validator(array $data) { return Validator::make($data, [ 'name' => ['required', 'string', 'max:255'], 'email' => ['required', 'string', 'email', 'max:255', 'unique:users'], 'password' => ['required', 'string', 'min:8', 'confirmed'], ]); } // 保存注册用户信息到数据库 protected function create(array $data) { return User::create([ 'name' => $data['name'], 'email' => $data['email'], 'password' => Hash::make($data['password']), ]); }
routes/web.php:
Route::get('register', 'AuthRegisterController@showRegistrationForm')->name('register'); Route::post('register', 'AuthRegisterController@register');
<form method="POST" action="{{ route('register') }}"> @csrf <div> <label for="name">用户名</label> <input id="name" type="text" name="name" value="{{ old('name') }}" required autofocus> </div> <div> <label for="email">邮箱</label> <input id="email" type="email" name="email" value="{{ old('email') }}" required> </div> <div> <label for="password">密码</label> <input id="password" type="password" name="password" required> </div> <div> <label for="password-confirm">确认密码</label> <input id="password-confirm" type="password" name="password_confirmation" required> </div> <div> <button type="submit">注册</button> </div> </form>
- User login process
routes/web.php:
Route::get('login', 'AuthLoginController@showLoginForm')->name('login'); Route::post('login', 'AuthLoginController@login'); Route::post('logout', 'AuthLoginController@logout')->name('logout');
LoginController controller corresponding to the
User model. In the controller, we need to implement the following method:
use IlluminateFoundationAuthAuthenticatesUsers; public function login(Request $request) { $this->validateLogin($request); if ($this->attemptLogin($request)) { return $this->sendLoginResponse($request); } return $this->sendFailedLoginResponse($request); } // 认证用户名和密码 protected function attemptLogin(Request $request) { return $this->guard()->attempt( $this->credentials($request), $request->filled('remember') ); } // 获取用户名和密码 protected function credentials(Request $request) { return $request->only($this->username(), 'password'); } public function username() { return 'email'; } // 用户退出登录方法 public function logout(Request $request) { $this->guard()->logout(); $request->session()->invalidate(); return redirect('/'); }
<form method="POST" action="{{ route('login') }}"> @csrf <div> <label for="email">邮箱</label> <input id="email" type="email" name="email" value="{{ old('email') }}" required autofocus> </div> <div> <label for="password">密码</label> <input id="password" type="password" name="password" required> </div> <div> <input type="checkbox" name="remember" id="remember" {{ old('remember') ? 'checked' : '' }}> <label for="remember">记住我</label> </div> <div> <button type="submit">登录</button> </div> </form>
attemptLogin method provided by the Laravel framework to verify the user's login information. If the verification is passed, the user information will be stored in the session and the user will be redirected to the child URL.
The above is the detailed content of laravel framework login registration process. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


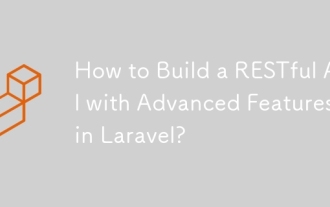
This article guides building robust Laravel RESTful APIs. It covers project setup, resource management, database interactions, serialization, authentication, authorization, testing, and crucial security best practices. Addressing scalability chall
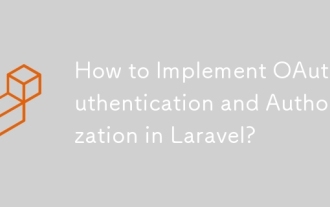
This article details implementing OAuth 2.0 authentication and authorization in Laravel. It covers using packages like league/oauth2-server or provider-specific solutions, emphasizing database setup, client registration, authorization server configu
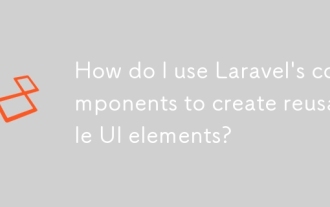
The article discusses creating and customizing reusable UI elements in Laravel using components, offering best practices for organization and suggesting enhancing packages.
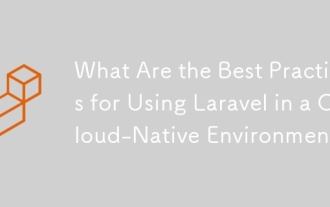
The article discusses best practices for deploying Laravel in cloud-native environments, focusing on scalability, reliability, and security. Key issues include containerization, microservices, stateless design, and optimization strategies.
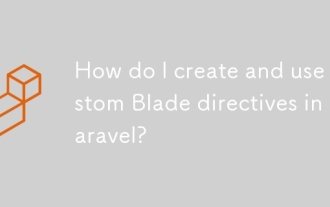
The article discusses creating and using custom Blade directives in Laravel to enhance templating. It covers defining directives, using them in templates, and managing them in large projects, highlighting benefits like improved code reusability and r
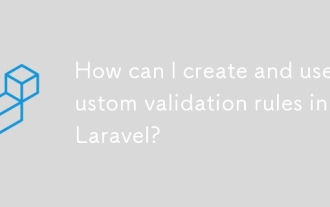
The article discusses creating and using custom validation rules in Laravel, offering steps to define and implement them. It highlights benefits like reusability and specificity, and provides methods to extend Laravel's validation system.
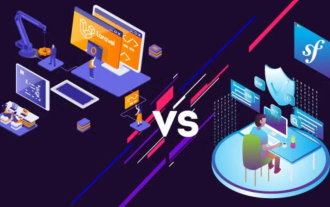
When it comes to choosing a PHP framework, Laravel and Symfony are among the most popular and widely used options. Each framework brings its own philosophy, features, and strengths to the table, making them suited for different projects and use cases. Understanding their differences and similarities is critical to selecting the right framework for your development needs.
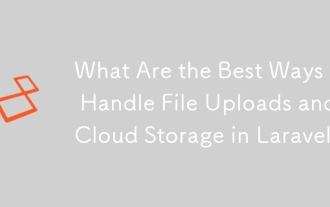
This article explores optimal file upload and cloud storage strategies in Laravel. It examines local storage vs. cloud providers (AWS S3, Google Cloud, Azure, DigitalOcean), emphasizing security (validation, sanitization, HTTPS) and performance opti
