JavaScript implements ping command
The Ping command is a tool for testing whether a connection can be established between two computers on the network. When developing web applications, it is often necessary to test the availability of the backend server, so being able to implement the Ping command in JavaScript is very useful for developers. Let's take a look at how to use JavaScript to implement the Ping command.
Introduction
The Ping command is a tool that detects whether the target host is reachable by sending network data packets. Its principle is to send an ICMP protocol data packet to the target host and then wait for its response. If the target host responds to this packet, it means that the target host exists and can be connected.
The process of implementing the Ping command in JavaScript mainly requires the use of XMLHttpRequest objects and WebSocket objects.
The XMLHttpRequest object is an object used to send and receive data through the HTTP protocol. You can send a Ping request to the server through the XMLHttpRequest object and read the Ping response returned by the server.
The WebSocket object is a full-duplex communication protocol based on the TCP protocol, which can achieve real-time two-way communication. Through the WebSocket object, you can directly send Ping requests to the server and receive Ping responses in the browser.
Implementation
The process of implementing the Ping command in JavaScript is mainly divided into two parts:
- Use the XMLHttpRequest object to send the Ping request, and read the response returned by the server Ping response.
- Use WebSocket object to implement real-time communication of Ping command.
Let’s introduce the implementation of these two parts in detail.
Use the XMLHttpRequest object to implement the Ping command
The first step to implement the Ping command is to use the XMLHttpRequest object to send a Ping request and read the Ping response returned by the server. The specific implementation steps are as follows:
- Create an XMLHttpRequest object.
var xhr = new XMLHttpRequest();
- Set the request method and request address.
var url = "http://www.example.com/ping"; // 这里应该是后端服务器实现ping的接口 xhr.open("POST", url, true);
- Set request header information.
xhr.setRequestHeader("Content-Type", "application/json");
- Send request data.
xhr.send(data);
- Processing response results.
xhr.onreadystatechange = function() { if (xhr.readyState === 4 && xhr.status === 200) { var response = xhr.responseText; // 处理返回的Ping响应数据 } };
Use WebSocket object to implement real-time communication of Ping command
The second step to implement Ping command is to use WebSocket object to implement real-time communication of Ping command. The specific implementation steps are as follows:
- Create a WebSocket object.
var ws = new WebSocket("ws://www.example.com/ping");
- Set the message processing method of the WebSocket object.
ws.onmessage = function(event) { var response = event.data; // 处理返回的Ping响应数据 };
- Send a Ping request to the server.
var request = { action: "ping", data: "hello world" }; ws.send(JSON.stringify(request));
- Close the WebSocket connection.
ws.onclose = function(event) { // WebSocket连接关闭 }; ws.close();
Summary
In developing web applications, how to test the availability of the back-end server is a very important issue. By using JavaScript to implement the Ping command, developers can more easily test the availability of the backend server. In the specific implementation process, XMLHttpRequest objects and WebSocket objects are mainly used to send Ping requests and process Ping responses.
The above is the detailed content of JavaScript implements ping command. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
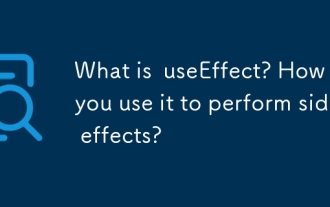
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.

Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.

Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
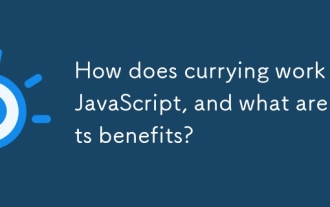
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
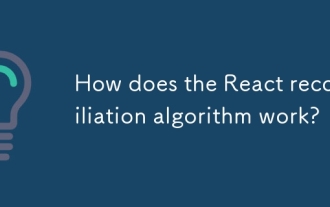
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
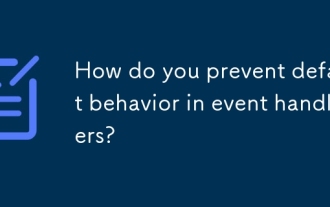
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
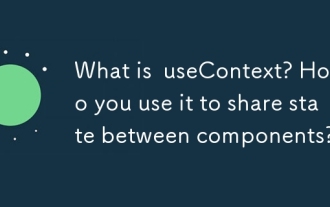
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
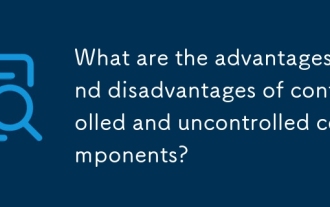
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.
