Common TCP/IP error analysis in Go language
The Go language is a growing programming language that is designed to be very suitable for implementing network applications with high performance, reliability and concurrency. When using Go to write network programs related to the TCP/IP protocol, we are prone to encounter various errors, and some common TCP/IP errors will also bring certain difficulties to the debugging of the program. This article will focus on the topic of how to solve common TCP/IP errors in the Go language.
1. EOF error
EOF (End Of File) error usually occurs when reading or writing certain data (such as files, sockets, etc.). In the Go language, a common place to encounter EOF errors is when reading socket streaming data, especially data in the TCP/IP protocol.
First of all, EOF is not a real error, but a special signal indicating that the data flow has ended and the program needs to handle it accordingly. Therefore, in Go language, we can determine whether we encounter an EOF error in the following way:
if err == io.EOF { // 处理结束信号 }
If you are using functions that read streaming data (such as net.Conn.Read(), bufio. Reader.ReadBytes(), etc.), then the EOF error indicates that the end of the input stream has been read. To solve this error, the simplest way is to determine whether EOF is read every time it is read. If it is read, it means that the data flow has ended and the loop can be exited.
2. Connection reset error
In Go language, connection reset error (Connection reset) is also a common TCP/IP error. It usually occurs when the connection between the client and the server is unstable, or when the server suddenly closes the connection.
In this case, the client will try to reconnect to the server, but if this error is encountered frequently, the performance of the program will be affected or even crash directly. Therefore, in order to better solve this problem, we can adopt some "backoff"-like strategies to handle connection interruptions.
Specifically, we can let the program pause for a period of time when encountering a connection reset error, and then try to reconnect. This can be achieved through the following code:
for { conn, err := net.Dial("tcp", "127.0.0.1:8080") if err != nil { log.Println("连接失败:", err) time.Sleep(time.Second) continue } // 处理连接成功后的逻辑 }
In this code, the program will pause for one second after the connection fails, and then reconnect. This "backoff" strategy can effectively mitigate the impact of connection reset errors and improve application robustness.
3. Port occupation error
If you forget to determine whether a port is occupied when starting the Go program, you are likely to encounter "bind: address already in use". mistake.
There are many ways to solve this problem. One of the simplest methods is to use the net.Listen function to detect whether the port is occupied, and if it is occupied, exit the program.
Specifically, this can be achieved through the following code:
func checkPort(port string) error { ln, err := net.Listen("tcp", fmt.Sprintf(":%s", port)) if err != nil { return err } ln.Close() return nil } // 在启动程序之前检查端口是否被占用 if err := checkPort("8080"); err !=nil { log.Fatalf("端口被占用:%s", err) }
In this code, we use net.Listen to detect whether the port is occupied, and if it is occupied, an error is returned. This method is simple and easy to understand, and can effectively help us avoid port occupation errors.
Summary:
The Go language is a language specifically designed for network programming, so when using it to write network programs related to the TCP/IP protocol, we still have to pay attention to some common issues TCP/IP error. This article introduces three common TCP/IP errors: EOF, connection reset, and port occupation, and provides methods to solve these problems. We hope it can help readers better debug and maintain their own Go programs.
The above is the detailed content of Common TCP/IP error analysis in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


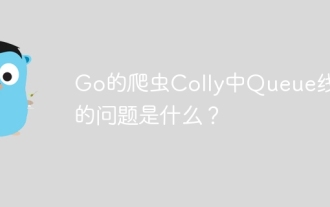
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
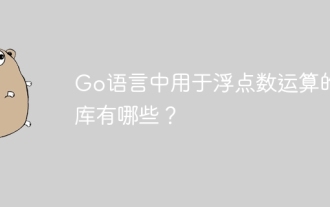
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
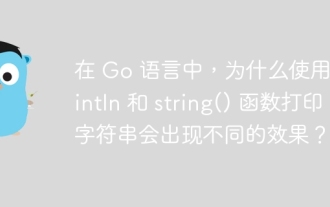
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
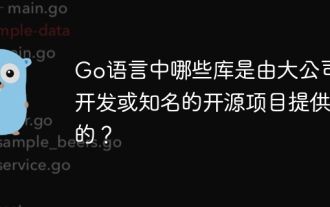
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
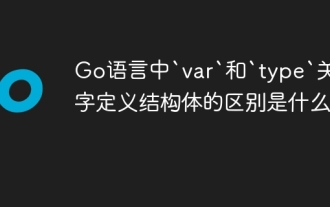
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
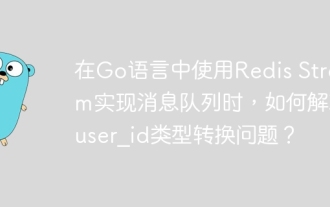
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
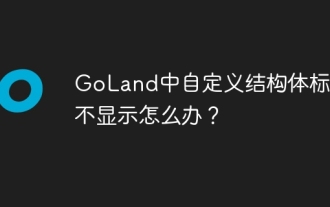
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
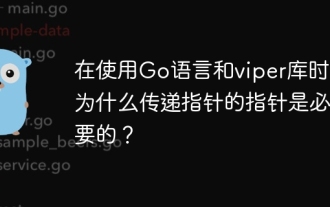
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
