


Benchmarking and performance analysis of concurrent programming in Go
With the continuous improvement of computer hardware technology, single-core CPUs can no longer meet the performance needs of computers. Therefore, how to fully utilize the performance of multi-core CPUs has become an important issue in the field of computer science. Concurrent programming is precisely to take advantage of the performance of multi-core CPUs and improve the efficiency and response speed of computer programs. As an efficient concurrent programming language, Go language's default concurrency model is widely accepted. However, in actual development, we need to evaluate and test the concurrency performance of the program in order to identify potential performance bottlenecks and optimization highlights. This article will introduce techniques and methods for benchmarking and performance analysis of concurrent programming in the Go language.
1. Basic knowledge of concurrent programming
In the Go language, concurrent programming is performed by using Goroutine and Channel. Goroutine is a lightweight thread that can realize automated multi-threaded concurrent processing by the Go language runtime scheduler (Goroutine Scheduler), avoiding the cumbersome and complicated operations of manually creating threads for developers. Channel is a type used to transfer data and can communicate between goroutines, avoiding the complex operations of using locks and condition variables.
2. Benchmark testing
Benchmark testing is a method that can test certain code fragments and evaluate their performance. In Go language, you can use the benchmark function in the test package for benchmark testing. Benchmark testing can repeatedly execute a function and return the average speed of its execution (the time taken to call the function each time).
The following shows a simple Benchmark test:
func BenchmarkExampleFunction(b *testing.B) { for n := 0; n < b.N; n++ { ExampleFunction() } }
In the above function, we repeatedly execute the ExampleFunction function by using a for loop. During the test, the test package will repeatedly call the ExampleFunction function and record its execution time. After the test is completed, the test results will display "BenchmarkExampleFunction X ns/op", where X represents the average number of nanoseconds for each function execution.
3. Performance Analysis
Performance analysis is a method to find out the performance bottlenecks and optimization highlights in the program. In Go language, you can use the pprof toolkit for performance analysis. pprof can generate a visual performance profile and mark the bottleneck points in the program on the profile, thereby helping developers find out where the program needs to be optimized.
When using pprof for performance analysis, you need to add a command line parameter "-cpuprofile" to generate a CPU profile and save it in a file:
go test -cpuprofile=profile.out
When the test is completed , the pprof toolbox will display the performance data discovered during the execution of the test. We can use the pprof profiler to open the generated CPU profile file as follows:
go tool pprof -web profile.out
pprof will start a web server locally and open the performance profiler in the browser. By using the performance analyzer, we can view all function calls in the program, as well as the time and CPU resources consumed by each function call. By looking at the performance analyzer, we can find out the bottleneck points in the program and optimize accordingly.
4. Summary
In order to make full use of the performance of multi-core CPUs, the Go language provides mechanisms such as Goroutine and Channel to achieve efficient concurrent programming. In actual development, concurrency performance needs to be evaluated and tested to identify potential performance bottlenecks and optimization highlights in the program. We can use the benchmark testing function of the testing package and the performance analysis function of the pprof tool package to evaluate the concurrency performance of the program, quickly find out the performance bottlenecks in the program, and optimize accordingly.
The above is the detailed content of Benchmarking and performance analysis of concurrent programming in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


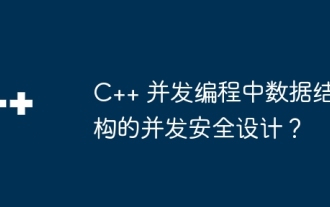
In C++ concurrent programming, the concurrency-safe design of data structures is crucial: Critical section: Use a mutex lock to create a code block that allows only one thread to execute at the same time. Read-write lock: allows multiple threads to read at the same time, but only one thread to write at the same time. Lock-free data structures: Use atomic operations to achieve concurrency safety without locks. Practical case: Thread-safe queue: Use critical sections to protect queue operations and achieve thread safety.
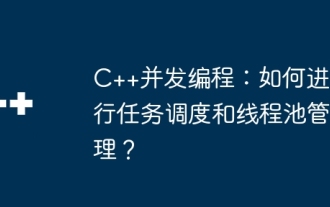
Task scheduling and thread pool management are the keys to improving efficiency and scalability in C++ concurrent programming. Task scheduling: Use std::thread to create new threads. Use the join() method to join the thread. Thread pool management: Create a ThreadPool object and specify the number of threads. Use the add_task() method to add tasks. Call the join() or stop() method to close the thread pool.
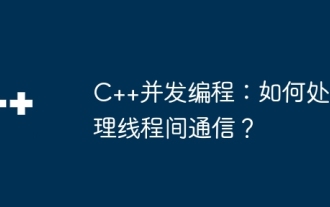
Methods for inter-thread communication in C++ include: shared memory, synchronization mechanisms (mutex locks, condition variables), pipes, and message queues. For example, use a mutex lock to protect a shared counter: declare a mutex lock (m) and a shared variable (counter); each thread updates the counter by locking (lock_guard); ensure that only one thread updates the counter at a time to prevent race conditions.

To avoid thread starvation, you can use fair locks to ensure fair allocation of resources, or set thread priorities. To solve priority inversion, you can use priority inheritance, which temporarily increases the priority of the thread holding the resource; or use lock promotion, which increases the priority of the thread that needs the resource.
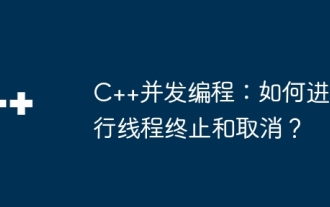
Thread termination and cancellation mechanisms in C++ include: Thread termination: std::thread::join() blocks the current thread until the target thread completes execution; std::thread::detach() detaches the target thread from thread management. Thread cancellation: std::thread::request_termination() requests the target thread to terminate execution; std::thread::get_id() obtains the target thread ID and can be used with std::terminate() to immediately terminate the target thread. In actual combat, request_termination() allows the thread to decide the timing of termination, and join() ensures that on the main line
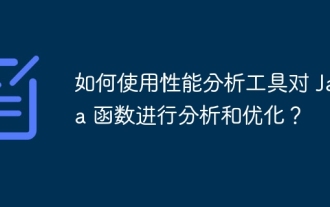
Java performance analysis tools can be used to analyze and optimize the performance of Java functions. Choose performance analysis tools: JVisualVM, VisualVM, JavaFlightRecorder (JFR), etc. Configure performance analysis tools: set sampling rate, enable events. Execute the function and collect data: Execute the function after enabling the profiling tool. Analyze performance data: identify bottleneck indicators such as CPU usage, memory usage, execution time, hot spots, etc. Optimize functions: Use optimization algorithms, refactor code, use caching and other technologies to improve efficiency.
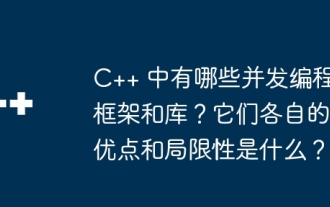
The C++ concurrent programming framework features the following options: lightweight threads (std::thread); thread-safe Boost concurrency containers and algorithms; OpenMP for shared memory multiprocessors; high-performance ThreadBuildingBlocks (TBB); cross-platform C++ concurrency interaction Operation library (cpp-Concur).
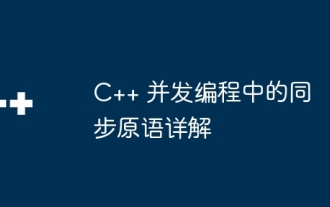
In C++ multi-threaded programming, the role of synchronization primitives is to ensure the correctness of multiple threads accessing shared resources. It includes: Mutex (Mutex): protects shared resources and prevents simultaneous access; Condition variable (ConditionVariable): thread Wait for specific conditions to be met before continuing execution; atomic operation: ensure that the operation is executed in an uninterruptible manner.
