Command line and programming skills in Go language
Jun 01, 2023 am 08:27 AMWith the continuous development of computer technology, more and more programming languages continue to emerge. Among them, the Go language has attracted much attention because of its simplicity and efficiency. The Go language aims to provide a system programming language similar to C for modern computers, but with the features and convenience of a high-level language. In the programming process of Go language, command line and programming skills are very important aspects. This article will introduce the relevant command line and programming skills in the Go language to help readers better master the Go language.
1. Command line related skills
The command line is a very important part of the Go language. It provides support for startup parameters, configuration and options for the program. In the command line, the most commonly used is the flag package, which can easily parse command line parameters.
- Parsing command line parameters
In the Go language, the flag package is a very commonly used package. The main function of the flag package is to parse command line flags and provide corresponding parameter values after the parsing is completed. The flag package has three main functions for parsing command line parameters and returning corresponding values:
• flag.String("name", "default value", "usage of name") • flag.Int("num", 123, "usage of num") • flag.Bool("debug", false, "usage of debug")
The above functions are used to parse command line parameters of string, integer and Boolean types respectively, and return corresponding values. . The first parameter is the parameter name, the second parameter is the default value, and the third parameter is the annotation information of the parameter.
It is very simple to use the flag package to parse command line parameters. Just call the flag.Parse() function in the main function. The following is an example for parsing command line parameters and outputting corresponding values:
package main import ( "flag" "fmt" ) func main() { // 定义命令行参数 var name = flag.String("name", "", "Input your name.") var age = flag.Int("age", 0, "Input your age.") var debug = flag.Bool("debug", false, "Enable debug mode.") // 解析命令行参数 flag.Parse() // 输出解析结果 fmt.Printf("Name: %s Age: %d Debug mode: %t ", *name, *age, *debug) }
- Help information for program parameters
In the process of programming, help information It is very necessary, it allows users to better understand the use and introduction of the program. In the Go language, the Usage() function of the flag package can be used to output help information very conveniently. Here is an example:
package main import ( "flag" "fmt" "os" ) func main() { var name string var age int var debug bool flag.StringVar(&name, "name", "", "Input your name.") flag.IntVar(&age, "age", 0, "Input your age.") flag.BoolVar(&debug, "debug", false, "Enable debug mode.") flag.Usage = func() { fmt.Fprintf(os.Stderr, `Usage: %s [options] Options: `, os.Args[0]) flag.PrintDefaults() } flag.Parse() fmt.Printf("Name: %s Age: %d Debug mode: %t ", name, age, debug) }
In the above code, use the flag.StringVar(), flag.IntVar() and flag.BoolVar() functions to define command line parameters, and use the flag.Usage function to output Help information for the program. In the flag.Usage function, use the fmt.Fprintf function to output the header of the help information, and then use the flag.PrintDefaults() function to output the definition and default values of the command line parameters.
2. Programming related skills
In addition to command line related skills, Go language programming is also a very important part. During the programming process, aspects such as code readability, flexibility, and scalability need to be considered.
- Producer-Consumer Model
The producer-consumer model is a very commonly used concurrency model, which can improve the parallel processing capabilities of the program. In Go language, the producer-consumer model can be implemented very conveniently using goroutine and channel.
The following is an example of a producer-consumer model implemented using goroutine and channel:
package main import ( "fmt" "math/rand" "time" ) func producer(out chan<- int) { for { num := rand.Intn(10) out <- num time.Sleep(100 * time.Millisecond) } } func consumer(in <-chan int) { for num := range in { fmt.Printf("Received: %d ", num) } } func main() { data := make(chan int) go producer(data) go consumer(data) time.Sleep(1 * time.Second) }
In the above code, use the rand package to generate a random number between 0 and 9 , and put it into the channel. Use the time.Sleep function to simulate the delay between the producer and the consumer, and then start the goroutine of the producer and consumer in the main function.
- Abstract interface implementation
In the programming process, the interface is a very important part, which can provide an abstraction layer between the application and the library. In the Go language, interfaces can help programs achieve modular development and improve code reusability.
The following is an example of an HTTP request implemented using an abstract interface:
package main import ( "fmt" "net/http" ) type HTTPClient interface { Do(req *http.Request) (*http.Response, error) } type MyHTTPClient struct{} func (c MyHTTPClient) Do(req *http.Request) (*http.Response, error) { return http.DefaultClient.Do(req) } func main() { client := MyHTTPClient{} request, _ := http.NewRequest("GET", "https://www.baidu.com", nil) response, err := client.Do(request) if err != nil { fmt.Println(err) return } fmt.Println(response.Status) }
In the above code, an HTTPClient interface is defined, including a method Do for sending HTTP requests. Then, the MyHTTPClient type is implemented, and the HTTP request is sent by implementing the method Do of the HTTPClient interface. Finally, use an instance client of the MyHTTPClient type to send the HTTP request.
Summary
This article mainly introduces the command line and programming skills in Go language, including using the flag package to parse command line parameters, output help information, producer-consumer model and abstract interface accomplish. Mastering these skills is very helpful to improve Go language programming capabilities and development efficiency. At the same time, readers can further explore and learn other skills and features of the Go language, and continuously improve their programming level and abilities.
The above is the detailed content of Command line and programming skills in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
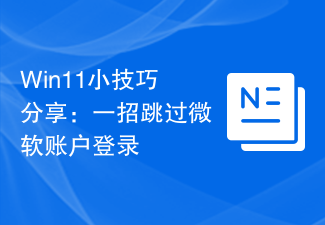
Win11 Tips Sharing: Skip Microsoft Account Login with One Trick
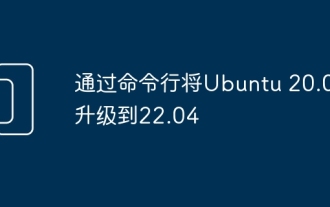
Upgrade Ubuntu 20.04 to 22.04 via command line
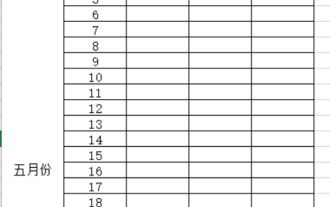
What are the tips for novices to create forms?
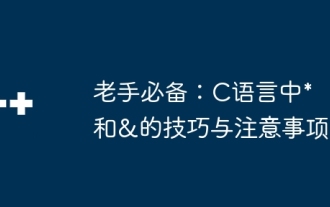
A must-have for veterans: Tips and precautions for * and & in C language
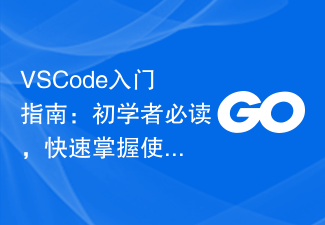
VSCode Getting Started Guide: A must-read for beginners to quickly master usage skills!
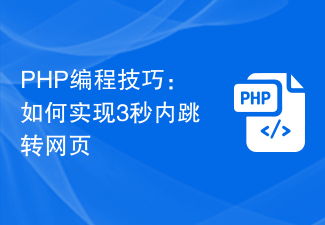
PHP programming skills: How to jump to the web page within 3 seconds
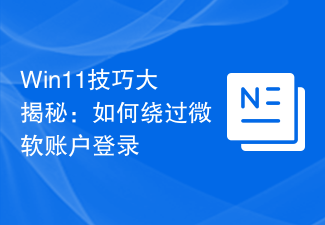
Win11 Tricks Revealed: How to Bypass Microsoft Account Login
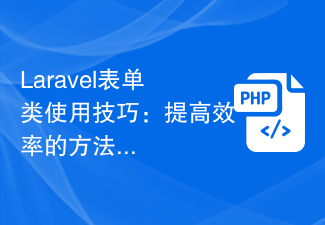
Tips for using Laravel form classes: ways to improve efficiency
