How to use springboot programmatic transaction TransactionTemplate
Use of TransactionTemplate
Summary: Inject TransactionTemplate into the class to use programmatic transactions in springboot.
spring supports two methods: programmatic transaction management and declarative transaction management.
Programmatic transaction management uses TransactionTemplate or directly uses the underlying PlatformTransactionManager. Spring recommends using TransactionTemplate to manage programming transactions.
Declarative transaction management is built on AOP. Its essence is to intercept the method before and after, and then create or join a transaction before the target method starts. After the target method is executed, the transaction is committed or rolled back according to the execution status. Spring Boot recommends using the @Transactional annotation to implement declarative transaction management.
1. Why use it?
In most cases, just declare the @Transactional annotation on the method to declare the transaction, which is simple, fast and convenient. However, the controllability of the @Transactional declarative transaction is too weak, and it can only be declared on the method or class. Fine-grained transaction control is not possible.
If the first 10 sql statements of a method are all select query statements, and only the last 2 sql statements are update statements, then only the last 2 sql statements can be processed.
2. How to use
<dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.2.0</version> </dependency>
to introduce the mybatis-spring-boot-starter dependency package into springboot.
The mybatis-spring-boot-starter dependency package contains the dependencies of spring-boot-starter-jdbc. spring-boot-starter-jdbc contains the DataSourceTransactionManager transaction manager and the automatic injection configuration class DataSourceTransactionManagerAutoConfiguration.
Used in code, just inject TransactionTemplate into the bean:
@Service public class TestServiceImpl { @Resource private TransactionTemplate transactionTemplate; public Object testTransaction() { //数据库查询 dao.select(1); return transactionTemplate.execute(status -> { //数据库新增 dao.insert(2); dao.insert(3); return new Object(); }); } }
TransactionTemplate is simple to use
/** * 事务模板 * @author zz * */ public class TransactionTemplateSupport { @Autowired private PlatformTransactionManager transactionManager; private TransactionTemplate requiredTransactionTemplate; protected TransactionTemplate getRequiresNewTransactionTemplate(){ if (requiredTransactionTemplate == null){ requiredTransactionTemplate = new TransactionTemplate(transactionManager); requiredTransactionTemplate.setPropagationBehavior(TransactionTemplate.PROPAGATION_REQUIRED); // requiredTransactionTemplate.setReadOnly(true); // requiredTransactionTemplate.setTimeout(30000); } return requiredTransactionTemplate; } }
@Service public class TestTransaction extends TransactionTemplateSupport { @Autowired private JdbcTemplate jdbcTemplate ; @Autowired private TransactionTemplate transactionTemplate; // @Transactional public void test(){ jdbcTemplate.execute("insert into user value (1,'aaa','aaa','aaa')"); int i = 1/0; jdbcTemplate.execute("insert into user value (2,'aaa','aaa','aaa')"); } public void test2(){ getRequiresNewTransactionTemplate() // transactionTemplate .execute(new TransactionCallback<Void>() { @Override public Void doInTransaction(TransactionStatus status) { jdbcTemplate.execute("insert into user value (11,'BBBB','aaa','aaa')"); int i = 1/0; jdbcTemplate.execute("insert into user value (21,'aaa','NNNN','aaa')"); return null; } }); } }
The above is the detailed content of How to use springboot programmatic transaction TransactionTemplate. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
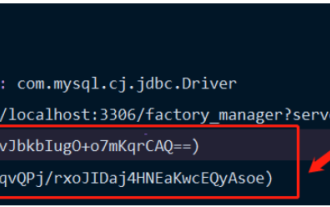
Introduction to Jasypt Jasypt is a java library that allows a developer to add basic encryption functionality to his/her project with minimal effort and does not require a deep understanding of how encryption works. High security for one-way and two-way encryption. , standards-based encryption technology. Encrypt passwords, text, numbers, binaries... Suitable for integration into Spring-based applications, open API, for use with any JCE provider... Add the following dependency: com.github.ulisesbocchiojasypt-spring-boot-starter2. 1.1Jasypt benefits protect our system security. Even if the code is leaked, the data source can be guaranteed.
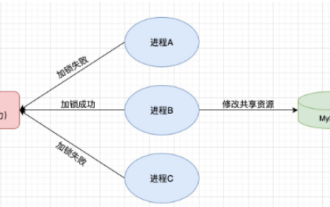
1. Redis implements distributed lock principle and why distributed locks are needed. Before talking about distributed locks, it is necessary to explain why distributed locks are needed. The opposite of distributed locks is stand-alone locks. When we write multi-threaded programs, we avoid data problems caused by operating a shared variable at the same time. We usually use a lock to mutually exclude the shared variables to ensure the correctness of the shared variables. Its scope of use is in the same process. If there are multiple processes that need to operate a shared resource at the same time, how can they be mutually exclusive? Today's business applications are usually microservice architecture, which also means that one application will deploy multiple processes. If multiple processes need to modify the same row of records in MySQL, in order to avoid dirty data caused by out-of-order operations, distribution needs to be introduced at this time. The style is locked. Want to achieve points
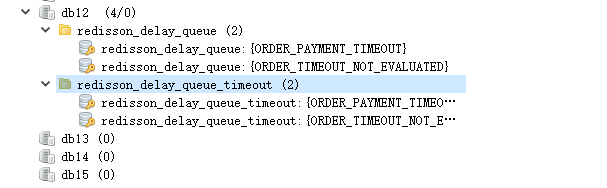
Usage scenario 1. The order was placed successfully but the payment was not made within 30 minutes. The payment timed out and the order was automatically canceled. 2. The order was signed and no evaluation was conducted for 7 days after signing. If the order times out and is not evaluated, the system defaults to a positive rating. 3. The order is placed successfully. If the merchant does not receive the order for 5 minutes, the order is cancelled. 4. The delivery times out, and push SMS reminder... For scenarios with long delays and low real-time performance, we can Use task scheduling to perform regular polling processing. For example: xxl-job Today we will pick
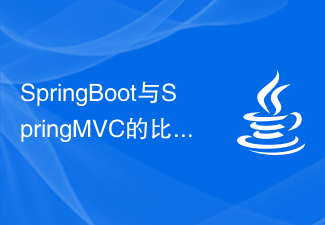
SpringBoot and SpringMVC are both commonly used frameworks in Java development, but there are some obvious differences between them. This article will explore the features and uses of these two frameworks and compare their differences. First, let's learn about SpringBoot. SpringBoot was developed by the Pivotal team to simplify the creation and deployment of applications based on the Spring framework. It provides a fast, lightweight way to build stand-alone, executable
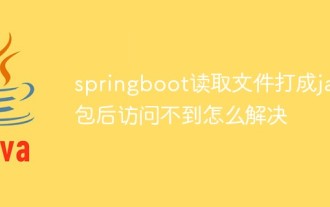
Springboot reads the file, but cannot access the latest development after packaging it into a jar package. There is a situation where springboot cannot read the file after packaging it into a jar package. The reason is that after packaging, the virtual path of the file is invalid and can only be accessed through the stream. Read. The file is under resources publicvoidtest(){Listnames=newArrayList();InputStreamReaderread=null;try{ClassPathResourceresource=newClassPathResource("name.txt");Input
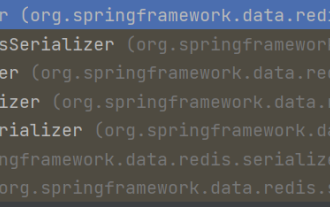
1. Customize RedisTemplate1.1, RedisAPI default serialization mechanism. The API-based Redis cache implementation uses the RedisTemplate template for data caching operations. Here, open the RedisTemplate class and view the source code information of the class. publicclassRedisTemplateextendsRedisAccessorimplementsRedisOperations, BeanClassLoaderAware{//Declare key, Various serialization methods of value, the initial value is empty @NullableprivateRedisSe
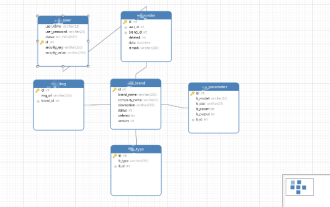
When Springboot+Mybatis-plus does not use SQL statements to perform multi-table adding operations, the problems I encountered are decomposed by simulating thinking in the test environment: Create a BrandDTO object with parameters to simulate passing parameters to the background. We all know that it is extremely difficult to perform multi-table operations in Mybatis-plus. If you do not use tools such as Mybatis-plus-join, you can only configure the corresponding Mapper.xml file and configure The smelly and long ResultMap, and then write the corresponding sql statement. Although this method seems cumbersome, it is highly flexible and allows us to
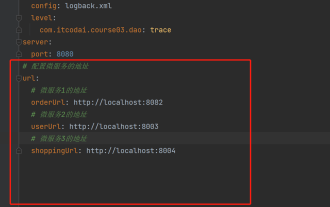
In projects, some configuration information is often needed. This information may have different configurations in the test environment and the production environment, and may need to be modified later based on actual business conditions. We cannot hard-code these configurations in the code. It is best to write them in the configuration file. For example, you can write this information in the application.yml file. So, how to get or use this address in the code? There are 2 methods. Method 1: We can get the value corresponding to the key in the configuration file (application.yml) through the ${key} annotated with @Value. This method is suitable for situations where there are relatively few microservices. Method 2: In actual projects, When business is complicated, logic
