How to learn and use Go's ORM framework GORM
GORM is an ORM framework for the Go programming language, whose full name is "Go Object Relational Mapping". It is a powerful ORM that is easy to use and efficient. Below we will introduce in detail how to learn and use GORM.
- What is the ORM framework?
The ORM framework is a software tool that allows developers to access and manipulate databases using an object model provided by a programming language. ORM is the abbreviation of "Object Relational Mapping". ORM helps simplify database access, making code more readable and easier to maintain. - Introduction to the GORM framework
GORM is a lightweight ORM framework that can be used to handle the interaction between the Go programming language and MySQL, PostgreSQL and SQLite. It is based on the concepts of other ORM frameworks and provides ease of use and efficiency without losing flexibility. - Steps to use GORM
The following are the steps to use the GORM framework: -
Installing GORM
Before you start using GORM, you need to download and install it. The installation process is simple. You can use the following command to install GORM:go get -u github.com/jinzhu/gorm
Copy after login Connect to the database
When using GORM, you need to set the database connection information in the configuration file. Here is an example of connecting to a MySQL database:import ( "github.com/jinzhu/gorm" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := gorm.Open("mysql", "{username}:{password}@tcp({host}:{port})/{database}?charset=utf8&parseTime=True&loc=Local") if err != nil { panic(err) } defer db.Close() }
Copy after loginIn order to perform database operations, you need to pass it to GORM. The following is sample code for passing a database example to GORM:
db, err := gorm.Open("mysql", "{username}:{password}@tcp({host}:{port})/{database}?charset=utf8&parseTime=True&loc=Local") if err != nil { panic(err) } defer db.Close() type User struct { ID uint `gorm:"primary_key"` Name string `gorm:"size:255"` } // 创建表 db.CreateTable(&User{})
Copy after login- Operation data table
GORM provides various methods for adding, deleting, modifying, and querying data tables. Here are some common usage example codes for GORM: query
db.First(&user, 1) // SELECT * FROM users WHERE id = 1; db.Find(&users) // SELECT * FROM users; db.Where("name = ?", "jinzhu").Find(&users) // SELECT * FROM users WHERE name = 'jinzhu';
Copy after logininsert
db.Create(&User{Name: "jinzhu"}) // INSERT INTO users (name) VALUES ("jinzhu");
Copy after loginupdate
db.Model(&user).Update("name", "jinzhu") // UPDATE users SET name = "jinzhu" WHERE id = 1;
Copy after loginDelete
db.Delete(&user) // DELETE FROM users WHERE id = 1;
Copy after login- Summary
GORM is a simple, easy-to-use Go ORM framework. You can easily use it for database access. In the process of learning to use GORM, you need to become familiar with its basics. With proper study and practice, you will be able to effectively use GORM to solve various database access problems.
The above is the detailed content of How to learn and use Go's ORM framework GORM. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


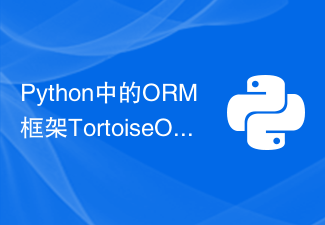
TortoiseORM is an asynchronous ORM framework developed based on the Python language and can be used to manage relational databases in Python asynchronous applications. This article will introduce how to use the TortoiseORM framework to create, read, update and delete data. You will also learn how to perform simple and complex queries from a relational database. Preparation Before starting this tutorial, you need to install Python (Python3.6+ is recommended) and install TortoiseOR.
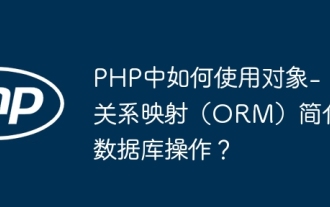
Database operations in PHP are simplified using ORM, which maps objects into relational databases. EloquentORM in Laravel allows you to interact with the database using object-oriented syntax. You can use ORM by defining model classes, using Eloquent methods, or building a blog system in practice.

With the development of the Internet, the development of Web applications has gradually been widely used. One of the most important languages is PHP. However, data management and processing has always been a problem faced by developers. For this reason, ORM has become a good choice for data processing. What is an ORM? ORM stands for Object-Relational Mapping. It is a method of converting objects in object-oriented programming language programs by using metadata that describes the mapping between objects and databases.
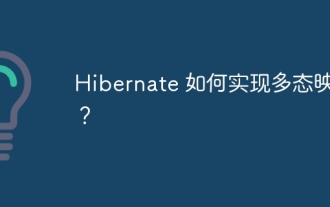
Hibernate polymorphic mapping can map inherited classes to the database and provides the following mapping types: joined-subclass: Create a separate table for the subclass, including all columns of the parent class. table-per-class: Create a separate table for subclasses, containing only subclass-specific columns. union-subclass: similar to joined-subclass, but the parent class table unions all subclass columns.
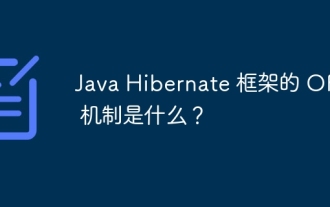
Hibernate is a JavaORM framework for mapping between Java objects and relational databases. Its ORM mechanism includes the following steps: Annotation/Configuration: The object class is marked with annotations or XML files, specifying its mapped database tables and columns. Session factory: manages the connection between Hibernate and the database. Session: Represents an active connection to the database and is used to perform query and update operations. Persistence: Save data to the database through the save() or update() method. Query: Use Criteria and HQL to define complex queries to retrieve data.
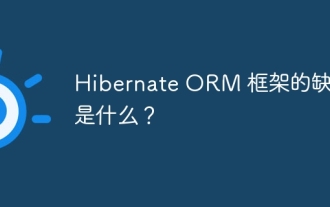
The HibernateORM framework has the following shortcomings: 1. Large memory consumption because it caches query results and entity objects; 2. High complexity, requiring in-depth understanding of the architecture and configuration; 3. Delayed loading delays, leading to unexpected delays; 4. Performance bottlenecks, in May occur when a large number of entities are loaded or updated at the same time; 5. Vendor-specific implementation, resulting in differences between databases.
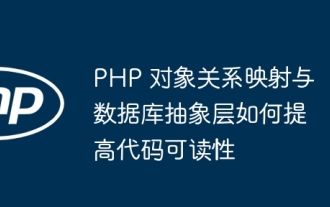
Answer: ORM (Object Relational Mapping) and DAL (Database Abstraction Layer) improve code readability by abstracting the underlying database implementation details. Detailed description: ORM uses an object-oriented approach to interact with the database, bringing the code closer to the application logic. DAL provides a common interface that is independent of database vendors, simplifying interaction with different databases. Using ORM and DAL can reduce the use of SQL statements and make the code more concise. In practical cases, ORM and DAL can simplify the query of product information and improve code readability.
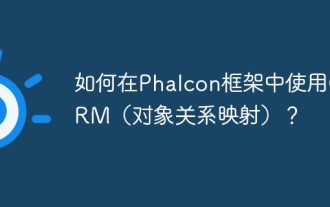
With the continuous development of web applications, corresponding web development frameworks are also emerging. Among them, the Phalcon framework is favored by more and more developers because of its high performance and flexibility. Phalcon framework provides many useful components, among which ORM (Object Relational Mapping) is considered one of the most important. This article will introduce how to use ORM in the Phalcon framework and some practical application examples. What is ORM First, we need to understand what ORM is. ORM is Object-Rel
