How nginx handles http requests
1. Interaction between event event and http framework
After receiving the http request line and http request header, the ngx_http_process_request function will be called to start processing the http request. Because an http request consists of 11 processing stages, and each processing stage allows multiple http modules to intervene, so in this function, the http modules at each stage will be scheduled to complete the request together.
//接收到http请求行与请求头后,http的处理流程,是第一个http处理请求的读事件回调 //这个函数执行后,将把读写事件的回调设置为ngx_http_request_handler。这样下次再有事件时 //将调用ngx_http_request_handler函数来处理,而不会再调用ngx_http_process_request了 static void ngx_http_process_request(ngx_http_request_t *r) { ngx_connection_t *c; c = r->connection; //因为已经接收完http请求行、请求头部了,准备调用各个http模块处理请求了。 //因此需要接收任何来自客户端的读事件,也就不存在接收http请求头部超时问题 if (c->read->timer_set) { ngx_del_timer(c->read); } //重新设置当前连接的读写事件回调 c->read->handler = ngx_http_request_handler; c->write->handler = ngx_http_request_handler; //设置http请求对象的读事件回调,这个回调不做任何的事情。 //那http请求对象的读事件回调,与上面的连接对应的读事件回调有什么关系呢? //当读事件发生后,连接对应的读事件回调ngx_http_request_handler会被调用, //在这个回调内会调用http请求对象的读事件回调ngx_http_block_reading,而这个回调是 //不会做任何事件的,因此相当于忽略了读事件。因为已经接收完了请求行请求头,现在要做的是调用各个http模块, //对接收到的请求行请求头进行处理 r->read_event_handler = ngx_http_block_reading; //调用各个http模块协同处理这个请求 ngx_http_handler(r); //处理子请求 ngx_http_run_posted_requests(c); }
The ngx_http_process_request function will only be called once. If one schedule cannot handle all 11 http stages, the read and write event callbacks corresponding to the connection object will be set to ngx_http_request_handler. The read event of the request object is set to ngx_http_block_reading, and the write event callback of the request object is set to ngx_http_core_run_phases. This callback is set in ngx_http_handler. In this way, the
ngx_http_process_request function will not be called when the event comes again. What is the relationship between the read and write event callbacks of the event event module and the read and write event callbacks of the http request object?
//http请求处理读与写事件的回调,在ngx_http_process_request函数中设置。 //这个函数中将会调用http请求对象的读写事件回调。将event事件模块与http框架关联起来 static void ngx_http_request_handler(ngx_event_t *ev) { //如果同时发生读写事件,则只有写事件才会触发。写事件优先级更高 if (ev->write) { r->write_event_handler(r); //在函数ngx_http_handler设置为:ngx_http_core_run_phases } else { r->read_event_handler(r); //在函数ngx_http_process_request设置为:ngx_http_block_reading } //处理子请求 ngx_http_run_posted_requests(c); }
As you can see, in the read event callback of the connection object , the read event callback of the http request object will be called. The write event callback of the connection object will call the write event callback of the http request object.
It can be seen from the figure that when the read event of the event occurs, the callback ngx_http_request_handler of the read event will be called after epoll returns. In this read event callback, the http framework will be called, that is, the read event callback ngx_http_block_reading of the http request object. The read event callback of this http request object does not do anything, which is equivalent to ignoring the read event. Therefore the http frame will return to the event module. So why ignore the read event? Because all the http request lines and request headers have been received. What we need to do now is to schedule the various http modules to work together to complete the processing of the received request lines and request headers. Therefore there is no need to receive any data from the client.
The processing of write events is much more complicated. When the write event of event occurs, the callback ngx_http_request_handler of the write event will be called after epoll returns. In this write event callback , and the http framework, which is the write event callback ngx_http_core_run_phases of the http request object, will be called. The callback of this http framework will schedule the handler methods of each http module involved in the 11 request stages to jointly complete the http request.
2. Scheduling the http module to process requests
In the above code, the function ngx_http_core_run_phases will be scheduled so that each http module can intervene in the http request. And this function is set in ngx_http_handler.
//调用各个http模块协同处理这个请求 void ngx_http_handler(ngx_http_request_t *r) { //不需要进行内部跳转。什么是内部跳转? 例如有个location结构,里面的 // if (!r->internal) { //将数组序号设为0,表示从数组第一个元素开始处理http请求 //这个下标很重要,决定了当前要处理的是11个阶段中的哪一个阶段, //以及由这个阶段的哪个http模块处理请求 r->phase_handler = 0; } else { //需要做内部跳转 cmcf = ngx_http_get_module_main_conf(r, ngx_http_core_module); //将序号设置为server_rewrite_index r->phase_handler = cmcf->phase_engine.server_rewrite_index; } //设置请求对象的写事件回调,这个回调将会调度介入11个http阶段的各个http模块 //共同完成对请求的处理 r->write_event_handler = ngx_http_core_run_phases; //开始调度介入11个http阶段的各个http模块 ngx_http_core_run_phases(r); }
The ngx_http_core_run_phases function is very simple. It schedules the checker methods of all http modules involved in 11 http processing stages.
//调用各个http模块协同处理这个请求, checker函数内部会修改phase_handler void ngx_http_core_run_phases(ngx_http_request_t *r) { cmcf = ngx_http_get_module_main_conf(r, ngx_http_core_module); ph = cmcf->phase_engine.handlers; //调用各个http模块的checker方法,使得各个http模块可以介入http请求 while (ph[r->phase_handler].checker) { rc = ph[r->phase_handler].checker(r, &ph[r->phase_handler]); //从http模块返回ngx_ok,http框架则会把控制全交还给事件模块 if (rc == ngx_ok) { return; } }
Assume that there are three http modules involved in the http request in stage 2, one module involved in the http request in stage 3, and one module involved in the request in stage 4. When phase 2 starts to be processed, all http modules in phase 2 will be called for processing. At this time, phase_handler points to the start position of phase 2. After each module in phase 2 is processed, phase_handler points to the next module in phase 2 until phase 2 processing is completed.
When all http modules in phase 2 are processed, phase_handler will point to phase 3
Because there is only one http module in phase 3, when all http modules in phase 3 are processed, phase_handler will point to phase 4
When was this handlers array created? What does the checker callback of each http module do? Next, we will analyze these two issues
3. 11 http request stages Array Creation
When parsing the nginx.conf configuration file, when the http block is parsed, the ngx_http_block function will be called to start parsing the http block. In this function, all http modules that need to intervene in the 11 http request stages will also be registered in the array.
//开始解析http块 static char * ngx_http_block(ngx_conf_t *cf, ngx_command_t *cmd, void *conf) { //http配置解析完成后的后续处理,使得各个http模块可以介入到11个http阶段 for (m = 0; ngx_modules[m]; m++) { if (ngx_modules[m]->type != ngx_http_module) { continue; } module = ngx_modules[m]->ctx; if (module->postconfiguration) { //每一个http模块的在这个postconfiguration函数中,都可以把自己注册到11个http阶段 if (module->postconfiguration(cf) != ngx_ok) { return ngx_conf_error; } } } }
For example, the ngx_http_static_module static module will set the ngx_http_content_phase phase callback that intervenes in the 11 http phases to ngx_http_static_handler
//静态模块将自己注册到11个http请求阶段中的ngx_http_content_phase阶段 static ngx_int_t ngx_http_static_init(ngx_conf_t *cf) { cmcf = ngx_http_conf_get_module_main_conf(cf, ngx_http_core_module); h = ngx_array_push(&cmcf->phases[ngx_http_content_phase].handlers); //静态模块在ngx_http_content_phase阶段的处理方法 *h = ngx_http_static_handler; return ngx_ok; }
例如: ngx_http_access_module访问权限模块,会将自己介入11个http阶段的ngx_http_access_phase阶段回调设置为ngx_http_access_handler
//访问权限模块将自己注册到11个http请求阶段中的ngx_http_access_phase阶段 static ngx_int_t ngx_http_access_init(ngx_conf_t *cf) { cmcf = ngx_http_conf_get_module_main_conf(cf, ngx_http_core_module); h = ngx_array_push(&cmcf->phases[ngx_http_access_phase].handlers); //访问权限模块在ngx_http_access_phase阶段的处理方法 *h = ngx_http_access_handler; return ngx_ok; }
上面的这些操作,只是把需要介入到11个http阶段的http模块保存到了ngx_http_core_main_conf_t中的phases成员中,并没有保存到phase_engine中。那什么时候将phases的内容保存到phase_engine中呢? 还是在ngx_http_block函数中完成
//开始解析http块 static char * ngx_http_block(ngx_conf_t *cf, ngx_command_t *cmd, void *conf) { //初始化请求的各个阶段 if (ngx_http_init_phase_handlers(cf, cmcf) != ngx_ok) { return ngx_conf_error; } }
假设阶段1有一个http模块介入请求,阶段2有三个http模块介入请求、阶段3也有一个http模块介入请求。则ngx_http_init_phase_handlers这个函数调用后,从ngx_http_phase_t phases[11]数组转换到ngx_http_phase_handler_t handlers数组的过程如下图所示:
//初始化请求的各个阶段 static ngx_int_t ngx_http_init_phase_handlers(ngx_conf_t *cf, ngx_http_core_main_conf_t *cmcf) { //11个http请求阶段,每一个阶段都可以有多个http模块介入。 //这里统计11个节点一共有多个少http模块。以便下面开辟空间 for (i = 0; i < ngx_http_log_phase; i++) { n += cmcf->phases[i].handlers.nelts; } //开辟空间,存放介入11个处理阶段的所有http模块的回调 ph = ngx_pcalloc(cf->pool,n * sizeof(ngx_http_phase_handler_t) + sizeof(void *)); cmcf->phase_engine.handlers = ph; n = 0; //对于每一个http处理阶段,给该阶段中所有介入的http模块赋值 for (i = 0; i < ngx_http_log_phase; i++) { h = cmcf->phases[i].handlers.elts; switch (i) { case ngx_http_server_rewrite_phase://根据请求的uri查找location之前,修改请求的uri阶段 if (cmcf->phase_engine.server_rewrite_index == (ngx_uint_t) -1) { cmcf->phase_engine.server_rewrite_index = n; //重定向模块在数组中的位置 } checker = ngx_http_core_rewrite_phase; //每一个阶段的checker回调 break; case ngx_http_find_config_phase://根据请求的uri查找location阶段(只能由http框架实现) find_config_index = n; ph->checker = ngx_http_core_find_config_phase; n++; ph++; continue; case ngx_http_rewrite_phase: //根据请求的rui查找location之后,修改请求的uri阶段 if (cmcf->phase_engine.location_rewrite_index == (ngx_uint_t) -1) { cmcf->phase_engine.location_rewrite_index = n; } checker = ngx_http_core_rewrite_phase; break; case ngx_http_post_rewrite_phase: //ngx_http_rewrite_phase阶段修改rul后,防止递归修改uri导致死循环阶段 if (use_rewrite) { ph->checker = ngx_http_core_post_rewrite_phase; ph->next = find_config_index;//目的是为了地址重写后,跳转到ngx_http_find_config_phase阶段,根据 //url重写查找location n++; ph++; } continue; case ngx_http_access_phase: //是否允许访问服务器阶段 checker = ngx_http_core_access_phase; n++; break; case ngx_http_post_access_phase: //根据ngx_http_access_phase阶段的错误码,给客户端构造响应阶段 if (use_access) { ph->checker = ngx_http_core_post_access_phase; ph->next = n; ph++; } continue; case ngx_http_try_files_phase: //try_file阶段 if (cmcf->try_files) { ph->checker = ngx_http_core_try_files_phase; n++; ph++; } continue; case ngx_http_content_phase: //处理http请求内容阶段,大部分http模块最愿意介入的阶段 checker = ngx_http_core_content_phase; break; default: //ngx_http_post_read_phase, //ngx_http_preaccess_phase, //ngx_http_log_phase三个阶段的checker方法 checker = ngx_http_core_generic_phase; } n += cmcf->phases[i].handlers.nelts; //每一个阶段中所介入的所有http模块,同一个阶段中的所有http模块有唯一的checker回调, //但handler回调每一个模块自己实现 for (j = cmcf->phases[i].handlers.nelts - 1; j >=0; j--) { ph->checker = checker; ph->handler = h[j]; ph->next = n; ph++; } } return ngx_ok; }
四、http阶段的checker回调
在11个http处理阶段中,每一个阶段都有一个checker函数,当然有些阶段的checker函数是相同的。对每一个处理阶段,介入这个阶段的所有http模块都共用同一个checker函数。这些checker函数的作用是调度介入这个阶段的所有http模块的handler方法,或者切换到一下个http请求阶段。下面分析下ngx_http_post_read_phase,ngx_http_preaccess_phase,ngx_http_log_phase三个阶段的checker方法。
//ngx_http_post_read_phase, //ngx_http_preaccess_phase, //ngx_http_log_phase三个阶段的checker方法 //返回值: ngx_ok,http框架会将控制权交还给epoll模块 ngx_int_t ngx_http_core_generic_phase(ngx_http_request_t *r,ngx_http_phase_handler_t *ph) { ngx_int_t rc; //调用http模块的处理方法,这样这个http模块就介入到了这个请求阶段 rc = ph->handler(r); //跳转到下一个http阶段执行 if (rc == ngx_ok) { r->phase_handler = ph->next; return ngx_again; } //执行本阶段的下一个http模块 if (rc == ngx_declined) { r->phase_handler++; return ngx_again; } //表示刚执行的handler无法在这一次调度中处理完这一个阶段, //需要多次调度才能完成 if (rc == ngx_again || rc == ngx_done) { return ngx_ok; } //返回出错 /* rc == ngx_error || rc == ngx_http_... */ ngx_http_finalize_request(r, rc); return ngx_ok; }
The above is the detailed content of How nginx handles http requests. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


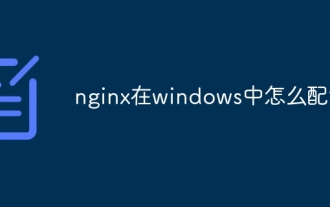
How to configure Nginx in Windows? Install Nginx and create a virtual host configuration. Modify the main configuration file and include the virtual host configuration. Start or reload Nginx. Test the configuration and view the website. Selectively enable SSL and configure SSL certificates. Selectively set the firewall to allow port 80 and 443 traffic.
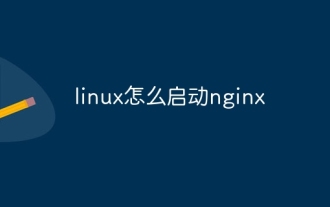
Steps to start Nginx in Linux: Check whether Nginx is installed. Use systemctl start nginx to start the Nginx service. Use systemctl enable nginx to enable automatic startup of Nginx at system startup. Use systemctl status nginx to verify that the startup is successful. Visit http://localhost in a web browser to view the default welcome page.
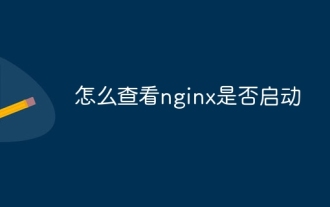
How to confirm whether Nginx is started: 1. Use the command line: systemctl status nginx (Linux/Unix), netstat -ano | findstr 80 (Windows); 2. Check whether port 80 is open; 3. Check the Nginx startup message in the system log; 4. Use third-party tools, such as Nagios, Zabbix, and Icinga.
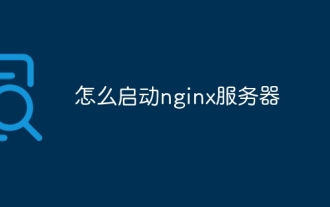
Starting an Nginx server requires different steps according to different operating systems: Linux/Unix system: Install the Nginx package (for example, using apt-get or yum). Use systemctl to start an Nginx service (for example, sudo systemctl start nginx). Windows system: Download and install Windows binary files. Start Nginx using the nginx.exe executable (for example, nginx.exe -c conf\nginx.conf). No matter which operating system you use, you can access the server IP
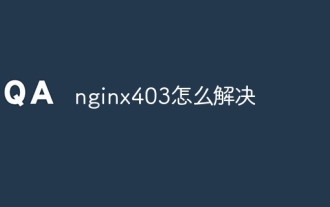
How to fix Nginx 403 Forbidden error? Check file or directory permissions; 2. Check .htaccess file; 3. Check Nginx configuration file; 4. Restart Nginx. Other possible causes include firewall rules, SELinux settings, or application issues.
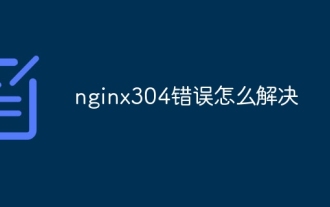
Answer to the question: 304 Not Modified error indicates that the browser has cached the latest resource version of the client request. Solution: 1. Clear the browser cache; 2. Disable the browser cache; 3. Configure Nginx to allow client cache; 4. Check file permissions; 5. Check file hash; 6. Disable CDN or reverse proxy cache; 7. Restart Nginx.
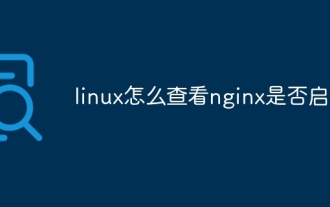
In Linux, use the following command to check whether Nginx is started: systemctl status nginx judges based on the command output: If "Active: active (running)" is displayed, Nginx is started. If "Active: inactive (dead)" is displayed, Nginx is stopped.
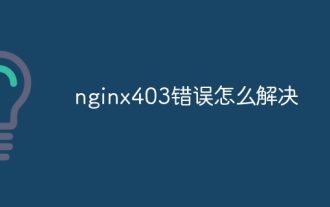
The server does not have permission to access the requested resource, resulting in a nginx 403 error. Solutions include: Check file permissions. Check the .htaccess configuration. Check nginx configuration. Configure SELinux permissions. Check the firewall rules. Troubleshoot other causes such as browser problems, server failures, or other possible errors.
