How to create custom pagination in CakePHP?
CakePHP is a powerful PHP framework that provides developers with many useful tools and features. One of them is pagination, which helps us divide large amounts of data into several pages, making browsing and manipulation easier.
By default, CakePHP provides some basic pagination methods, but sometimes you may need to create some custom pagination methods. This article will show you how to create custom pagination in CakePHP.
Step 1: Create a custom paging class
First, we need to create a custom paging class. This class will be responsible for handling all paging related logic. Create a new file named CustomPaginator.php in the app/Lib/Utility directory and add the following code to the file:
<?php App::uses('PaginatorComponent', 'Controller/Component'); class CustomPaginator extends PaginatorComponent { // Override the default method to customize the pagination logic public function paginate($object = null, $scope = array(), $whitelist = array()) { // Get the current page number $page = isset($this->Controller->request->params['named']['page']) ? $this->Controller->request->params['named']['page'] : 1; // Set the default pagination values $perPage = 10; $start = ($page - 1) * $perPage; // Get the total count of records $count = $object->find('count', array('conditions' => $scope)); // Build the pagination data $result = array( 'count' => $count, 'perPage' => $perPage, 'page' => $page, 'totalPages' => ceil($count / $perPage), 'start' => $start, 'end' => ($start + $perPage) > $count ? $count : ($start + $perPage - 1), 'hasPrevPage' => $page > 1, 'hasNextPage' => ($start + $perPage) < $count ); // Set the pagination data in the controller $this->Controller->set('paging', $result); // Return the paginated records return $object->find('all', array('conditions' => $scope, 'limit' => $perPage, 'offset' => $start)); } }
This custom pagination class is based on CakePHP’s default paginator class PaginatorComponent. We overridden the paginate() method to implement custom pagination logic. It takes the following parameters:
- $object: The model object to be paginated.
- $scope: Query conditions, used to filter records to be paginated.
- $whitelist: Whitelist array, used to allow or deny specific query parameters
In our implementation, we first get the number of the current page, and then set the default per page Number of records and starting record number. Next, we use the find() method to get the total number of records, and then calculate the total number of pages and the number of ending records. Finally, we set all the paging data to the controller's 'paging' variable and return the paginated records.
Step 2: Instantiate the custom paging class
Now that we have created the custom paging class, we need to instantiate it in the controller. To do this, we need to add the following code to our controller:
<?php App::uses('AppController', 'Controller'); App::uses('CustomPaginator', 'Lib/Utility'); class UsersController extends AppController { public $components = array('CustomPaginator'); public $paginate = array('CustomPaginator'); public function index() { // Get all users $this->set('users', $this->CustomPaginator->paginate($this->User)); } }
We use App::uses() to load the custom pagination class and then instantiate it in the controller. We also added the custom pagination class to the controller using the $components and $paginate attributes.
In our index() action, we call $CustomPaginator->paginate() and pass our User model object to it. We then set the paginated user data into view variables.
Step 3: Create a paginated view
Finally, we need to create a view to display the paginated data. Add the following code in the 'views/users/index.ctp' file:
<h1> Users </h1> <ul> <?php foreach ($users as $user): ?> <li> <?php echo $user['User']['name']; ?> </li> <?php endforeach; ?> </ul> <div class="pagination"> <?php echo $this->Paginator->prev('<< ' . __('Previous'), array(), null, array('class' => 'disabled')); echo $this->Paginator->numbers(); echo $this->Paginator->next(__('Next') . ' >>', array(), null, array('class' => 'disabled')); ?> </div>
This view is just a simple list of Users and then displays paginated navigation links.
We use the prev(), numbers() and next() methods of PaginatorHelper to generate navigation links. These methods will generate links based on the '$CustomPaginator' component we defined in the controller.
Conclusion
Custom pagination can give you greater control and flexibility to meet your specific needs. In this article, we show you how to create custom pagination in CakePHP. Now you can apply this knowledge to develop more customized applications.
The above is the detailed content of How to create custom pagination in CakePHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


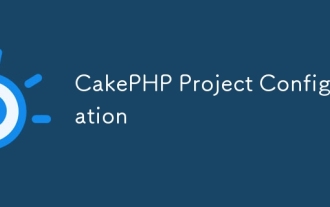
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
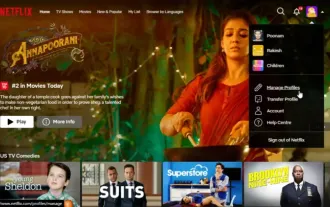
An avatar on Netflix is a visual representation of your streaming identity. Users can go beyond the default avatar to express their personality. Continue reading this article to learn how to set a custom profile picture in the Netflix app. How to quickly set a custom avatar in Netflix In Netflix, there is no built-in feature to set a profile picture. However, you can do this by installing the Netflix extension on your browser. First, install a custom profile picture for the Netflix extension on your browser. You can buy it in the Chrome store. After installing the extension, open Netflix on your browser and log into your account. Navigate to your profile in the upper right corner and click
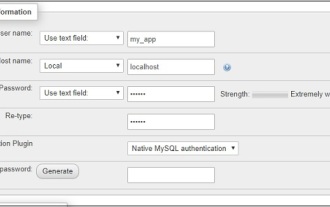
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
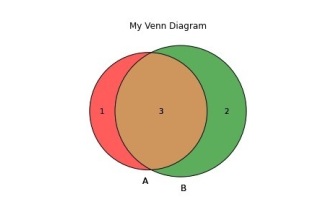
A Venn diagram is a diagram used to represent relationships between sets. To create a Venn diagram we will use matplotlib. Matplotlib is a commonly used data visualization library in Python for creating interactive charts and graphs. It is also used to create interactive images and charts. Matplotlib provides many functions to customize charts and graphs. In this tutorial, we will illustrate three examples to customize Venn diagrams. The Chinese translation of Example is: Example This is a simple example of creating the intersection of two Venn diagrams; first, we imported the necessary libraries and imported venns. Then we create the dataset as a Python set, after that we use the "venn2()" function to create
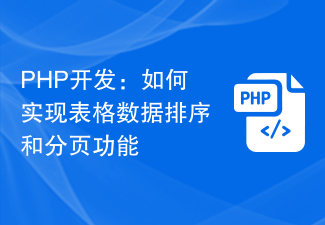
PHP development: How to implement table data sorting and paging functions In web development, processing large amounts of data is a common task. For tables that need to display a large amount of data, it is usually necessary to implement data sorting and paging functions to provide a good user experience and optimize system performance. This article will introduce how to use PHP to implement the sorting and paging functions of table data, and give specific code examples. The sorting function implements the sorting function in the table, allowing users to sort in ascending or descending order according to different fields. The following is an implementation form
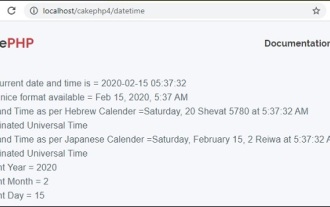
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
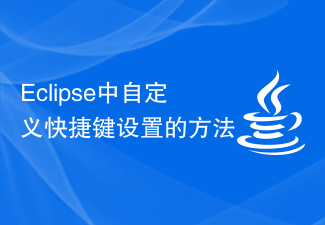
How to customize shortcut key settings in Eclipse? As a developer, mastering shortcut keys is one of the keys to improving efficiency when coding in Eclipse. As a powerful integrated development environment, Eclipse not only provides many default shortcut keys, but also allows users to customize them according to their own preferences. This article will introduce how to customize shortcut key settings in Eclipse and give specific code examples. Open Eclipse First, open Eclipse and enter
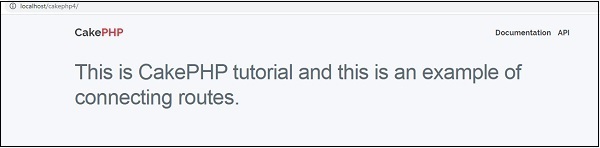
In this chapter, we are going to learn the following topics related to routing ?
