Data persistence and CRUD operations in Go language
With the development of the Internet, the storage and use of data have become more and more important, especially for some data-intensive applications. As an emerging programming language, Go language has gradually emerged in the evolving data warehouse and big data scenarios with its fast, safe, concurrency and efficient features. This article will introduce how to implement data persistence and CRUD operations in Go language.
Step One: Choose a Suitable Database
Go language provides many database drivers, including PostgreSQL, SQLite, MySQL, MariaDB and MongoDB, etc. Before starting to write a program, you first need to choose a database suitable for your application and continue to learn the relevant drivers. Among them, some of the most commonly used are:
- SQL databases: PostgreSQL, MySQL, SQLite, and MariaDB.
- NoSQL database: MongoDB, etc.
Here we will not discuss the differences between various databases in detail. Simply put, when establishing complex relationships with relational data, SQL databases are usually more practical; while when managing semi-structured data, NoSQL databases may be more suitable when efficient processing of multidimensional data queries is required.
Step 2: Write a database driver
The Go language provides many built-in database drivers, such as database/sql, which provides a unified SQL interface for all Go programmers. Of course, the interfaces implemented by different database drivers are also different.
Here, we take the MySQL database as an example to explain how to establish a database connection in the Go language. Since the database/sql driver of the Go language provides a unified sql interface for various relational databases, programmers only need to change the relevant parameters to use different types of relational databases in applications. The following is an example of creating a MySQL driver:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" ) func getDatabaseConnection() (*sql.DB, error) { db, err := sql.Open("mysql", "root:@tcp(127.0.0.1:3306)/test") return db, err }
In the above code, you can see that the MySQL driver is introduced using import
. Next, we use the sql.Open()
method to establish a database connection and pass in some parameters of the connection, such as account number, password, host address and port number, etc. Here we are using the root
account with no password, the host address is 127.0.0.1
, the port number is 3306
, and the database name is test
.
After the process is completed, you need to call the db.Ping()
method to test whether you have successfully connected to the database and return a connection object of type *sql.DB
.
Step 3: Perform CRUD operations
Now that we have established a database connection, we can perform CRUD operations. CRUD operations represent add (Create), read (Read), update (Update) and delete (Delete) respectively. These operations usually involve reading, querying, modifying, and deleting data from tables in the database.
The following is a simple CRUD example, which can add a record to the database with three fields: "id, name, age":
func createUser(newUser User) error { db, err := getDatabaseConnection() if err != nil { return err } defer db.Close() _, err = db.Exec("INSERT INTO users(name, age) VALUES(?, ?)", newUser.Name, newUser.Age) if err != nil { return err } return nil }
In the above code, ## is used #db.Exec()method writes data into the database. Among them,
? is a dynamic binding parameter. In this way, the precompiled SQL query statement will automatically fill in the corresponding parameters, thus ensuring the security of the program.
func getUserByID(id int64) (*User, error) { db, err := getDatabaseConnection() if err != nil { return nil, err } defer db.Close() row := db.QueryRow("SELECT id, name, age FROM users WHERE id = ?", id) user := new(User) err = row.Scan(&user.ID, &user.Name, &user.Age) if err != nil { return nil, err } return user, nil }
row object. Use the
row.Scan() method to fill the value of a specific column in the query result into the
User structure (assuming that the
User structure has the field
ID , Name and Age).
The above is the detailed content of Data persistence and CRUD operations in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


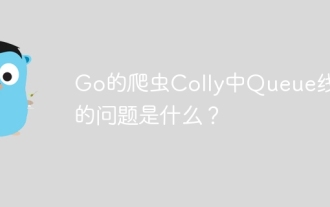
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
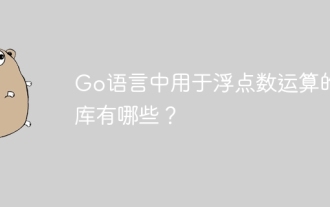
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
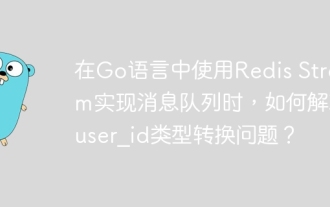
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
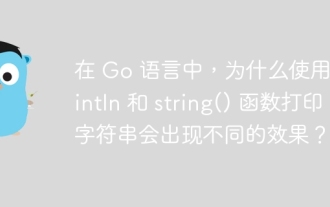
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
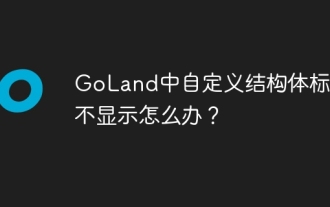
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
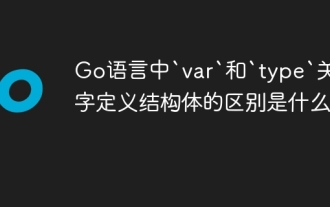
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
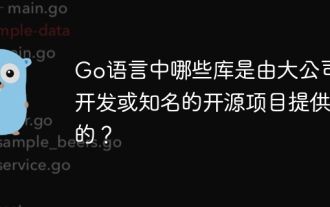
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
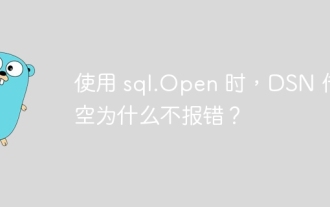
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
