How to develop a single-page web application using Golang
With the continuous development of the Internet, the demand for Web applications is also increasing day by day. In the past, web applications were usually composed of multiple pages, but now more and more applications choose to use single page applications (SPA). Single-page applications are very suitable for mobile access, and users do not need to wait for the entire page to reload, which increases the user experience. In this article, we will introduce how to use Golang to develop SPA applications.
- What is a single-page application?
A single-page application refers to a Web application with only one HTML file. It uses JavaScript, usually through Ajax technology to interact with the server. By storing the application's state on the client side, users can interact with the application without refreshing the entire page.
- Advantages of SPA
When using traditional multi-page applications, the entire page will be reloaded every time you switch pages. SPA only needs to be loaded once, and all subsequent interactions will be conducted on a single page. This approach can reduce workload and improve user experience.
SPA can also respond to user actions faster because users do not need to wait for the entire page to reload. This not only improves user experience, but also improves the quality of SEO.
However, SPA applications also have some disadvantages, such as they usually use more JavaScript. Excessive use of JavaScript may cause the page to take too long to load, affecting the user experience.
- Golang as a backend language
When developing SPA on the front end, we need a backend language to support the regular operation of the application. Here we choose to use Golang.
Golang is an open source programming language developed by Google. It is an efficient and concise language. Golang has a simple syntax, garbage collection, and native support for concurrency. These features make Golang very suitable for web development, especially the development of single-page web applications.
- Basic elements of SPA application
When building a SPA application, we need to consider the following basic elements:
4.1 Routing
Routing refers to the process of mapping different parts of an application to URLs. In SPA, each page corresponds to a URL address. When the user clicks on the navigation menu, the application automatically loads the corresponding section or page.
In Golang, we can use the gorilla/mux package to implement routing.
4.2 Controller
Controller refers to the code that handles web requests. Controllers typically include menus and other user interface components. In SPA, controllers usually include front-end frameworks such as React or AngularJS.
In Golang, we can use the net/http package to implement controllers.
4.3 Database
Databases are often used to store data for web applications. In SPA, the database usage is the same as in traditional web applications. We can use common relational databases such as MySQL or PostgreSQL.
In Golang, we can use the sql package to interact with the database.
- Recommended technology stack for SPA applications
SPA applications can be implemented using many back-end languages and front-end frameworks. Here we provide a technology stack suitable for Golang:
- Web Framework:
Among the web frameworks for Golang, the most popular ones are Gin and Echo. Both frameworks are lightweight, efficient, and easy to use.
- Front-end framework:
The most popular front-end frameworks are React, Vue and AngularJS. Among these frameworks, React is one of the easiest to use options.
- UI Library:
We can also use UI library to implement SPA applications. Currently the more popular ones are Bootstrap and Material UI.
- Database:
In terms of data storage, we can choose MySQL or PostgreSQL. If we consider NoSQL, MongoDB is a good choice.
The above technology stack can provide us with a good SPA application development environment. In addition, we can also use other technologies to enhance the application, such as Redis, MongoDB, etc.
- How to build a SPA application
Now that we have identified the technology stack, here are the basic steps for building a SPA application:
- Create routing configuration: In Golang, we can configure routing using the gorilla/mux package.
- Create a controller: Use the net/http package to create a controller.
- Create and store data: Use the sql package to create and store data.
- Design UI: Use front-end frameworks such as React, Vue or AngularJS to design the SPA interface.
- Integrate UI libraries: Integrate UI libraries such as Bootstrap or Material UI to add a wide range of UI components to the application.
- Build and deploy: Use the Go compiler to build the application as an executable and deploy it to the server.
- Conclusion
Using Golang to develop SPA applications allows us to use an efficient, concise and easy-to-use language to create a website with a good user experience.
In this article, we discuss the basic elements of SPA, the advantages of Golang as a back-end language, and the technology stack and development process of SPA applications.
Although SPA applications may suffer from performance limitations in some cases, they are still an excellent choice to support better performance and user experience for web applications.
The above is the detailed content of How to develop a single-page web application using Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
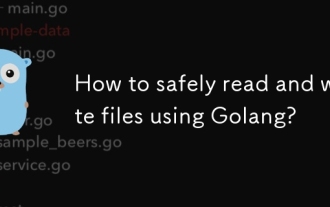
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
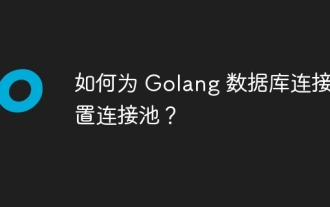
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
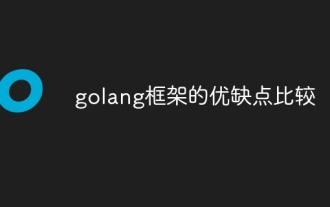
The Go framework stands out due to its high performance and concurrency advantages, but it also has some disadvantages, such as being relatively new, having a small developer ecosystem, and lacking some features. Additionally, rapid changes and learning curves can vary from framework to framework. The Gin framework is a popular choice for building RESTful APIs due to its efficient routing, built-in JSON support, and powerful error handling.

Best practices: Create custom errors using well-defined error types (errors package) Provide more details Log errors appropriately Propagate errors correctly and avoid hiding or suppressing Wrap errors as needed to add context
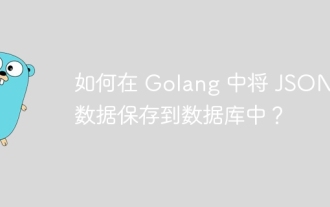
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
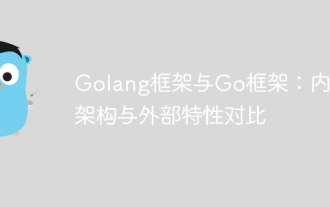
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
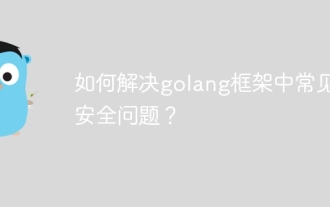
How to address common security issues in the Go framework With the widespread adoption of the Go framework in web development, ensuring its security is crucial. The following is a practical guide to solving common security problems, with sample code: 1. SQL Injection Use prepared statements or parameterized queries to prevent SQL injection attacks. For example: constquery="SELECT*FROMusersWHEREusername=?"stmt,err:=db.Prepare(query)iferr!=nil{//Handleerror}err=stmt.QueryR
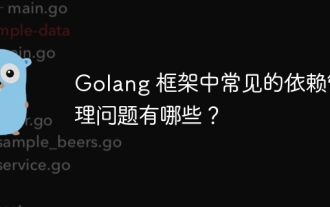
Common problems and solutions in Go framework dependency management: Dependency conflicts: Use dependency management tools, specify the accepted version range, and check for dependency conflicts. Vendor lock-in: Resolved by code duplication, GoModulesV2 file locking, or regular cleaning of the vendor directory. Security vulnerabilities: Use security auditing tools, choose reputable providers, monitor security bulletins and keep dependencies updated.
