Best practices for exception classification in PHP programs
When writing PHP code, exception handling is an integral part, which can make the code more robust and maintainable. However, exception handling also needs to be used with caution, otherwise it may cause more problems. In this article, I will share some best practices for exception classification in PHP programs to help you make better use of exception handling to improve code quality.
The concept of exception
In PHP, exception refers to an error or unexpected situation that occurs when the program is running. Typically, exceptions cause the program to stop running and output an exception message. Exception handling refers to pre-defining how to handle exception situations in the code so that the program can continue to run and provide useful error information.
Exception classification
In PHP, exception classification is very important. The following are some best practices that can help you get better results in exception handling:
1. Runtime exception (Runtime exception) and checked exception (Checked exception)
PHP Exceptions in can be divided into two types: runtime exceptions and checked exceptions.
Runtime exceptions are exceptions detected by the PHP runtime, such as division by 0 exceptions, null pointer exceptions, etc. These exceptions usually involve program logic in the code or invalid input.
In contrast, a checked exception is an exception that is implicitly defined by the code and needs to be caught explicitly when called. For example, if you try to read a file that does not exist, PHP will throw an uncaught exception. This type of exception usually involves access to external resources or operating system level errors.
2. Application-level exceptions and library-level exceptions
Application-level exceptions are exceptions thrown by the application itself. If some application-level error occurs, such as a file not being found or a database connection failure, the application should throw an appropriate exception.
In contrast, library-level exceptions are exceptions thrown by the underlying library or PHP extension. These exceptions usually involve access to underlying resources such as file systems, network connections, or databases.
3. System exception and business exception
The last exception classification is system exception and business exception. When writing code, you should prioritize handling system exceptions because these exceptions are often unrelated to the logic and data in the code and may cause system crashes. For example, if an error occurs that fails to connect to the database, a system exception should be thrown.
In contrast, business exceptions refer to application-specific errors. For example, if the user provides invalid input, a business exception should be thrown.
Best Practices
Understanding exception classification is very important, but there are several other best practices that can help you make better use of exception handling and improve code quality.
1. Use try-catch block
The try-catch block is a common way to handle exceptions. An example is as follows:
try { // 一些可能会抛出异常的代码 } catch (Exception $e) { // 当异常发生时,进行异常处理 }
In this example, the try block contains code that may throw an exception. If an error occurs, control is transferred to the catch block. Within a catch block, the program can handle the exception, such as logging it or displaying a useful error message to the user.
2. Use specific exception classes
In PHP, exception classes can be defined as custom classes, and these classes can be used to represent different types of exceptions. For example, a custom exception named DatabaseException can be used to represent exceptions related to database operations.
When using the PHP Exceptions API, using specific exception classes can better organize and structure exception handling code. If multiple exception classes are used in the code, you can use the instanceof operator to filter and handle them accordingly.
3. Avoid catching all exceptions
In PHP, you can use the catch(Exception $e) syntax to catch all types of exceptions. However, this approach is not a best practice because it may mask the real problem and make it difficult to locate the root cause of the problem.
Instead, throw and catch exceptions as specifically as possible. This way, it's clear where the problem occurred in the code, and helpful error messages are provided whenever possible.
Conclusion
Exception handling is an important part of improving the quality of PHP code. Understanding exception classifications and best practices is essential to help you better organize your exception handling code and provide useful error messages. When you write PHP code, always pay attention to exception handling and use the best practices possible to build your code base.
The above is the detailed content of Best practices for exception classification in PHP programs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


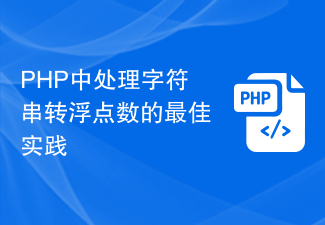
Converting strings to floating point numbers in PHP is a common requirement during the development process. For example, the amount field read from the database is of string type and needs to be converted into floating point numbers for numerical calculations. In this article, we will introduce the best practices for converting strings to floating point numbers in PHP and give specific code examples. First of all, we need to make it clear that there are two main ways to convert strings to floating point numbers in PHP: using (float) type conversion or using (floatval) function. Below we will introduce these two
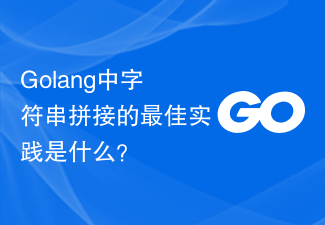
What are the best practices for string concatenation in Golang? In Golang, string concatenation is a common operation, but efficiency and performance must be taken into consideration. When dealing with a large number of string concatenations, choosing the appropriate method can significantly improve the performance of the program. The following will introduce several best practices for string concatenation in Golang, with specific code examples. Using the Join function of the strings package In Golang, using the Join function of the strings package is an efficient string splicing method.
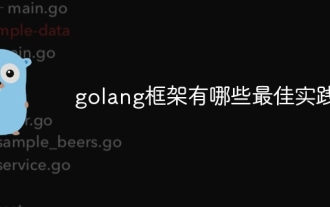
When using Go frameworks, best practices include: Choose a lightweight framework such as Gin or Echo. Follow RESTful principles and use standard HTTP verbs and formats. Leverage middleware to simplify tasks such as authentication and logging. Handle errors correctly, using error types and meaningful messages. Write unit and integration tests to ensure the application is functioning properly.

In Go language, good indentation is the key to code readability. When writing code, a unified indentation style can make the code clearer and easier to understand. This article will explore the best practices for indentation in the Go language and provide specific code examples. Use spaces instead of tabs In Go, it is recommended to use spaces instead of tabs for indentation. This can avoid typesetting problems caused by inconsistent tab widths in different editors. The number of spaces for indentation. Go language officially recommends using 4 spaces as the number of spaces for indentation. This allows the code to be
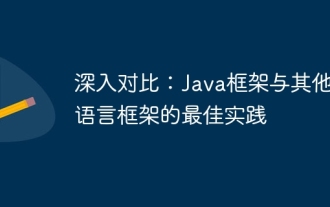
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
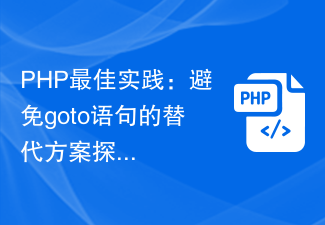
PHP Best Practices: Alternatives to Avoiding Goto Statements Explored In PHP programming, a goto statement is a control structure that allows a direct jump to another location in a program. Although the goto statement can simplify code structure and flow control, its use is widely considered to be a bad practice because it can easily lead to code confusion, reduced readability, and debugging difficulties. In actual development, in order to avoid using goto statements, we need to find alternative methods to achieve the same function. This article will explore some alternatives,
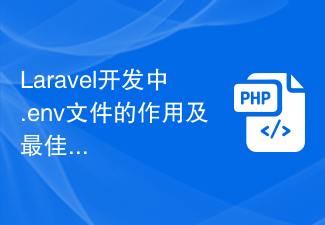
The role and best practices of .env files in Laravel development In Laravel application development, .env files are considered to be one of the most important files. It carries some key configuration information, such as database connection information, application environment, application keys, etc. In this article, we’ll take a deep dive into the role of .env files and best practices, along with concrete code examples. 1. The role of the .env file First, we need to understand the role of the .env file. In a Laravel should
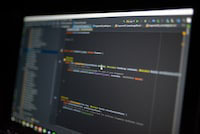
This article will explain in detail about starting a new or restoring an existing session in PHP. The editor thinks it is very practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Session Management: Start a New Session or Resume an Existing Session Introduction Session management is crucial in PHP, it allows you to store and access user data during the user session. This article details how to start a new session or resume an existing session in PHP. Start a new session The function session_start() checks whether a session exists, if not, it creates a new session. It can also read session data and convert it
