How to use image recognition technology in Python?
In the field of contemporary science and technology, image recognition technology is becoming more and more important. Image recognition technology helps us identify and classify entities extracted from digital images, which are then used in data analysis and prediction. Python is a very popular programming language that is also well suited for working with image recognition technology. In this article, we will learn how to use image recognition technology in Python and what we can do with it.
1. Image processing library
Before starting to use image recognition technology, it is best to understand some basic knowledge of image processing libraries. The most commonly used image processing libraries in Python include OpenCV, Pillow, and Scikit-image. In this article, we will focus on using two libraries, OpenCV and Scikit-image.
2. OpenCV
OpenCV is an open source computer vision library that can be used on different platforms. OpenCV provides a large number of algorithms and functions that can be used to implement digital image processing, analysis and computer vision. Here are the basic steps for using OpenCV for image recognition:
1. Install OpenCV
Before you start using OpenCV, you need to install it on your computer. The OpenCV library can be installed through pip and conda commands. On Windows, you can install it with the following command:
pip install opencv-python
Alternatively, you can use conda to install OpenCV:
conda install -c conda-forge opencv
2. Load the image
Next, you need to load the image you want to analyze Image. In Python, you can load a single image or multiple images using the OpenCV function cv2.imread().
import cv2 # load an image image = cv2.imread("path/to/image")
3. Preprocessing images
Before using OpenCV, the image needs to be preprocessed. The following processing can be done on the image:
# convert the image to grayscale gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # apply a Gaussian blur to remove noise blurred = cv2.GaussianBlur(gray, (5, 5), 0) # apply edge detection to extract edges edges = cv2.Canny(blurred, 50, 200)
4. Identify objects
Once the image has been preprocessed, objects can be identified using OpenCV’s algorithms and functions. Objects can be marked as rectangles or circles, etc.
# perform an object detection (contours, _) = cv2.findContours(edges.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) for c in contours: # compute the bounding box of the object (x, y, w, h) = cv2.boundingRect(c) # draw the bounding box around the object cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
5. Display results
Use OpenCV to display the processed image.
# display the result cv2.imshow("Object Detection", image) cv2.waitKey(0)
3. Scikit-image
Scikit-image is an image processing library based on the Python language. It also provides many image processing algorithms and functions. The following are the basic steps for using Scikit-image for image recognition:
1. Install Scikit-image
You can use the following command to install the Scikit-image library:
pip install scikit-image
2 .Load Image
Similarly, before using Scikit-image, you need to load the image you want to analyze.
from skimage import io # load the image image = io.imread("path/to/image")
3. Preprocess the image
Before using Scikit-image, you also need to preprocess the image. The following processing can be done on the image:
from skimage.filters import threshold_local from skimage.color import rgb2gray # convert the image to grayscale gray = rgb2gray(image) # apply a threshold to the image thresh = threshold_local(gray, 51, offset=10)
4. Identify objects
Use Scikit-image's algorithms and functions to identify objects and mark the objects as rectangles or circles, etc.
from skimage import measure from skimage.color import label2rgb from skimage.draw import rectangle # find contours in the image contours = measure.find_contours(thresh, 0.8) # draw a rectangle around each object for n, contour in enumerate(contours): row_min, col_min = contour.min(axis=0) row_max, col_max = contour.max(axis=0) rect = rectangle((row_min, col_min), (row_max, col_max), shape=image.shape) image[rect] = 0
5. Display results
Use Scikit-image to display the processed image.
io.imshow(image) io.show()
Conclusion
Through this article, we learned how to use OpenCV and Scikit-image in Python for image recognition. These two libraries are one of the most popular image processing libraries in Python and can help us with image processing, analysis, and computer vision work. Using image recognition technology, invisible entities can be easily extracted from digital images and used in data analysis and prediction, for example, it can be applied to medicine, security and finance. Although this article provides some basic usage methods, image recognition technology is a very complex and varied field, and there are many other algorithms and techniques that can be used. Therefore, learning and exploring this field is a very interesting and worthwhile process.
The above is the detailed content of How to use image recognition technology in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


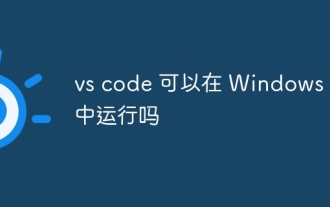
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
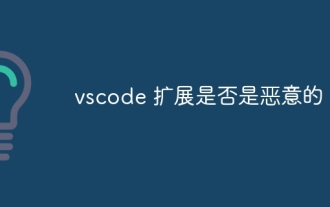
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
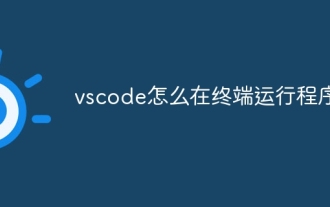
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
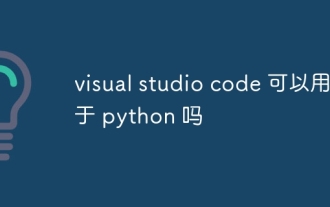
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
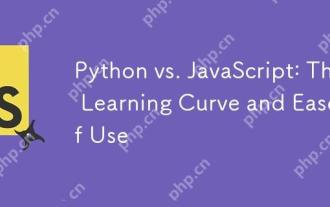
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
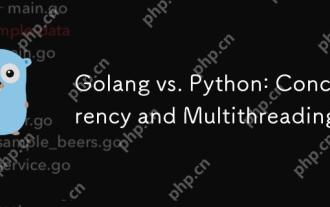
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
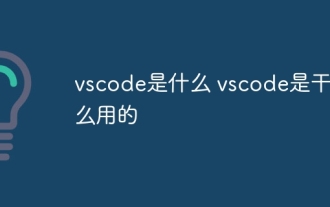
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
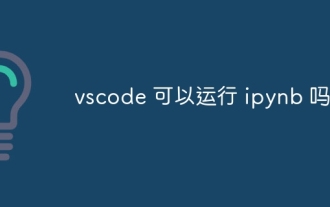
The key to running Jupyter Notebook in VS Code is to ensure that the Python environment is properly configured, understand that the code execution order is consistent with the cell order, and be aware of large files or external libraries that may affect performance. The code completion and debugging functions provided by VS Code can greatly improve coding efficiency and reduce errors.
