How to do functional programming with PHP
PHP is a widely used server-side language. One of the reasons why many web developers like to use PHP is its rich function library and simple and easy-to-use function syntax. Functional programming is a programming paradigm that well encapsulates data and behavior, making the code more modular and easy to maintain and test. In this article, we will introduce how to use PHP for functional programming.
- Functional Programming Basics
The core idea of functional programming is to treat functions as first-class citizens, and functions themselves can be passed, returned, and composed like variables. In functional programming, we don't modify mutable state, but we transform and filter it through functions.
PHP itself supports functional programming, and there are many built-in functions that can be used for functional processing. For example array_map, array_filter, etc. Below we will demonstrate how to use these functions to implement common functional programming operations.
- Higher-order functions
Higher-order functions refer to functions that can accept functions as parameters or return functions. Such functions can be used to compose and reuse code. In PHP, commonly used higher-order functions include array_map, array_filter, array_reduce, etc.
The array_map function can accept a function and an array as parameters and return a new array. The elements of the new array are the values obtained after the elements in the original array are converted by the function.
For example, we have an array $x=[1,2,3,4]$ and want to square each element in the array. You can use the following code:
function square($x) { return $x * $x; } $array = [1, 2, 3, 4]; $new_array = array_map('square', $array); var_dump($new_array); // 输出 [1, 4, 9, 16]
function is_even($x) { return $x % 2 == 0; } $array = [1, 2, 3, 4]; $new_array = array_filter($array, 'is_even'); var_dump($new_array); // 输出 [2, 4]
function add($a, $b) { return $a + $b; } $array = [1, 2, 3, 4]; $result = array_reduce($array, 'add'); var_dump($result); // 输出 10
- Anonymous function
$square = function($x) { return $x * $x; }; $result = $square(3); var_dump($result); // 输出 9
$square = fn($x) => $x * $x; $result = $square(3); var_dump($result); // 输出 9
- Curi Currying is a technique that converts a function with multiple parameters into a function with a single parameter. In functional programming, currying can be used to reuse and simplify functions. In PHP, you can use closures and higher-order functions to implement currying. For example, we have a function add(x,y,z) and hope to implement a curried version:
function add($x, $y, $z) { return $x + $y + $z; } $curried_add = function($x) use ($add) { return function($y) use ($x, $add) { return function($z) use ($x, $y, $add) { return $add($x, $y, $z); }; }; }; $result = $curried_add(1)(2)(3); var_dump($result); // 输出 6
- Combined function refers to combining multiple Functions are combined together to form new functions, which can be used to simplify the code and enhance the readability and maintainability of the code. In PHP, you can use closures and higher-order functions to achieve function combination. For example, we have two functions $f(x)$ and $g(x)$, and want to implement a combined function $h(x)$ such that $h(x) = f(g(x))$:
function f($x) { return $x + 1; } function g($x) { return $x * 2; } $compose = function($f, $g) { return function($x) use ($f, $g) { return $f($g($x)); }; }; $h = $compose('f', 'g'); $result = $h(2); var_dump($result); // 输出 5
- This article introduces how to use PHP for functional programming, including common techniques such as higher-order functions, anonymous functions, currying, and function composition. Functional programming can make code more modular, easier to test and maintain. For web developers, understanding functional programming is very valuable.
The above is the detailed content of How to do functional programming with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


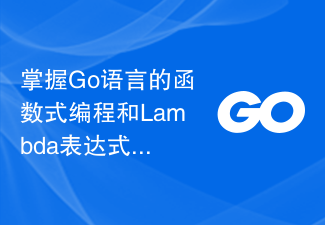
In the contemporary programming world, Functional Programming (FP for short) has gradually become a popular programming paradigm. It emphasizes using functions as basic building blocks to build programs, and regards the calculation process as the continuous transfer and conversion between functions. In recent years, Go language (also known as Golang) has gradually been widely used in various fields due to its simplicity, efficiency, concurrency safety and other characteristics. Although the Go language itself is not a purely functional programming language, it provides sufficient functionality.
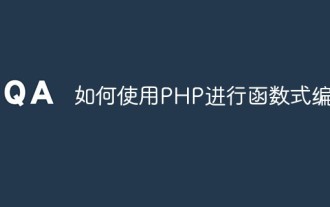
PHP is a widely used server-side language. One of the reasons why many web developers like to use PHP is its rich function library and simple and easy-to-use function syntax. Functional programming is a programming paradigm that well encapsulates data and behavior, making the code more modular and easy to maintain and test. In this article, we will introduce how to use PHP for functional programming. Functional Programming Basics The core idea of functional programming is to treat functions as first-class citizens. Functions themselves can be passed, returned, and composed like variables.
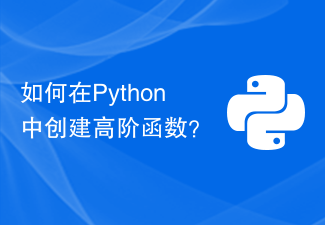
In Python, a function that takes another function as an argument or returns a function as output is called a higher-order function. Let's see its features - the function can be stored in a variable. This function can be passed as a parameter to another function. Higher-order functions can be stored in the form of lists, hash tables, etc. Functions can be returned from functions. Let's look at some examples − Functions as objects The Chinese translation of Example is: Example In this example, these functions are treated as objects. Here, function demo() is assigned to a variable - #Creatingafunctiondefdemo(mystr):returnmystr.swapcase()#swappingthecase
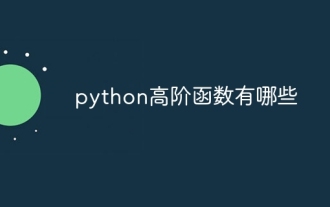
High-order functions include map(), filter(), reduce(), lambda function, partial(), etc. Detailed introduction: 1. map(): This built-in function accepts a function and one or more iterable objects as input, and then returns an iterator that applies the input function to each element of the iterable object; 2. filter() : This built-in function takes a function and an iterable object as input, and returns an iterator that yields those elements that cause the input function to return True, etc.
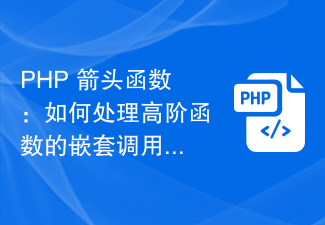
PHP arrow functions: How to handle nested calls of higher-order functions, specific code examples are needed Introduction: In PHP7.4 version, the concept of arrow functions (arrowfunctions) was introduced. Arrow functions are a concise way of writing and can be processed elegantly. Nested calls to higher-order functions. This article will introduce the basic use of arrow functions and demonstrate how to handle nested calls of higher-order functions through specific code examples. 1. What is an arrow function? Arrow function is a new feature introduced in PHP7.4 version. It is a
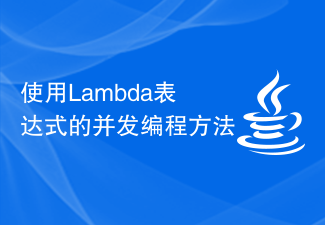
An important addition to JavaSE8 is the lambda expression feature. Use expressions to express method interfaces clearly and concisely. Collection libraries are very helpful. Collections can be iterated, filtered, and data extracted for useful purposes. To implement functional interfaces, lambda expressions are widely used. It saves a lot of code. Lambda expressions allow us to provide implementations without redefining methods. It is only here that the implementation code is formed by writing the code. The compiler does not create a .class file because Javalambda expressions are treated as functions. Functional interface @FunctionalInterface is a Java annotation that declares an interface as a functional interface.
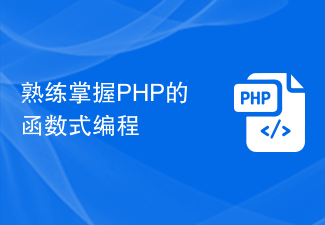
With the continuous development of Internet technology, PHP is becoming more and more popular among developers as an efficient, flexible, easy-to-learn and easy-to-use programming language. In PHP, functional programming is a commonly used programming method, which uses functions as basic building blocks to build programs and achieve code reuse and modularization. In this article, we will explore how to become proficient in functional programming in PHP. 1. Basic concepts of functional programming Functional programming is a function-based programming paradigm and a powerful tool for building health programs. The core idea is to write letters
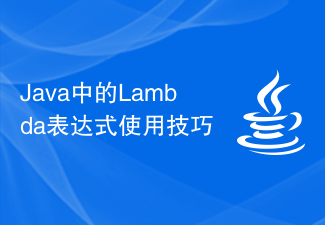
In Java 8, Lambda expressions are a very powerful tool that can greatly simplify code, making it more compact and easier to read. Lambda expressions can also improve the reusability and maintainability of code, thus effectively promoting development efficiency. This article will introduce some tips for using Lambda expressions in Java to help developers make better use of this powerful tool. Tip 1: Use Lambda expressions instead of anonymous inner classes before Java8 if we need
