String manipulation techniques in Java
As a widely used programming language, Java has strong processing capabilities in string operations. This article will introduce some commonly used string manipulation techniques in Java to help readers better handle strings.
1. Creation of strings
In Java, we can create string objects through string literals or using constructors. For example:
String str1 = "hello"; String str2 = new String("hello");
When creating a string using string literals, Java will first check whether the string already exists in the string constant pool. If it exists, it will directly return a reference to the string; if If it does not exist, a new string object will be created in the string constant pool and its reference will be returned.
When you use the new
keyword and constructor to create a string, Java will create a new string object in the heap memory.
2. String splicing
The string splicing operation in Java can use the
operator or the concat()
method. For example:
String str1 = "hello"; String str2 = "world"; String str3 = str1 + " " + str2; String str4 = str1.concat(" ").concat(str2);
Using the
operator can easily splice two strings together. If multiple string splicing is involved, multiple
can be used. Splicing. The concat()
method is generally used to concatenate one string to the end of another string.
It should be noted that the string splicing operation will generate a new string object and will not modify the original string object.
3. String interception
In Java, you can use the substring()
method to intercept a string. The parameters of this method include the starting position and end of the interception. Location. For example:
String str = "hello world"; String subStr1 = str.substring(0, 5); String subStr2 = str.substring(6);
The first parameter indicates the starting position of interception (inclusive), and the second parameter indicates the end position of interception (exclusive). When only one parameter is passed in, it means intercepting from that position to the end of the string.
It should be noted that this method does not modify the original string, but returns a new string object.
4. Search and replace strings
In Java, you can use the indexOf()
method to find the first occurrence of a specified substring in a string. You can also use the lastIndexOf()
method to find the last occurrence. For example:
String str = "hello world"; int index1 = str.indexOf("o"); int index2 = str.lastIndexOf("o");
If the substring does not exist in the string, return -1
.
In addition, you can also use the replace()
method in Java to replace substrings in a string. For example:
String str = "hello world"; String newStr = str.replace("world", "Java");
This method will return a new string object, and the original string will not be modified.
5. String separation
In Java, you can use the split()
method to split a string according to the specified delimiter and return the split characters string array. For example:
String str = "apple,orange,banana"; String[] array = str.split(",");
The above code will separate the strings by commas and return an array containing three strings.
6. String conversion
In Java, you can use the valueOf()
method to convert other types of data into strings, for example:
String str1 = String.valueOf(123); String str2 = String.valueOf(3.14); String str3 = String.valueOf(true);
In addition, when converting between strings and other data types, you can use the parseXxx()
method, where Xxx
represents the data type, for example:
int n1 = Integer.parseInt("123"); double n2 = Double.parseDouble("3.14"); boolean n3 = Boolean.parseBoolean("true");
It should be noted that this method can only be used to convert numerical values or Boolean values represented by strings, otherwise an exception will be thrown.
Summary
This article introduces common string manipulation techniques in Java, including string creation, splicing, interception, search, replacement, separation and conversion, etc. I hope readers can flexibly apply these methods in their work and better handle strings.
The above is the detailed content of String manipulation techniques in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


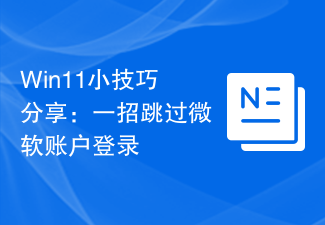
Win11 Tips Sharing: One trick to skip Microsoft account login Windows 11 is the latest operating system launched by Microsoft, with a new design style and many practical functions. However, for some users, having to log in to their Microsoft account every time they boot up the system can be a bit annoying. If you are one of them, you might as well try the following tips, which will allow you to skip logging in with a Microsoft account and enter the desktop interface directly. First, we need to create a local account in the system to log in instead of a Microsoft account. The advantage of doing this is
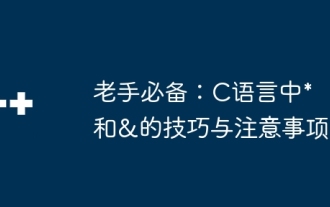
In C language, it represents a pointer, which stores the address of other variables; & represents the address operator, which returns the memory address of a variable. Tips for using pointers include defining pointers, dereferencing pointers, and ensuring that pointers point to valid addresses; tips for using address operators & include obtaining variable addresses, and returning the address of the first element of the array when obtaining the address of an array element. A practical example demonstrating the use of pointer and address operators to reverse a string.
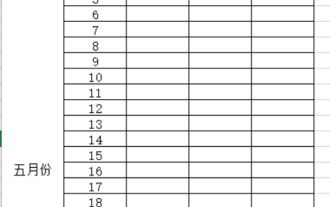
We often create and edit tables in excel, but as a novice who has just come into contact with the software, how to use excel to create tables is not as easy as it is for us. Below, we will conduct some drills on some steps of table creation that novices, that is, beginners, need to master. We hope it will be helpful to those in need. A sample form for beginners is shown below: Let’s see how to complete it! 1. There are two methods to create a new excel document. You can right-click the mouse on a blank location on the [Desktop] - [New] - [xls] file. You can also [Start]-[All Programs]-[Microsoft Office]-[Microsoft Excel 20**] 2. Double-click our new ex
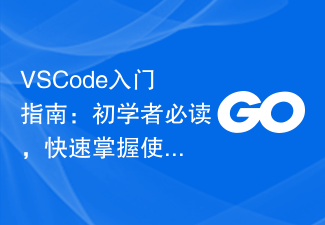
VSCode (Visual Studio Code) is an open source code editor developed by Microsoft. It has powerful functions and rich plug-in support, making it one of the preferred tools for developers. This article will provide an introductory guide for beginners to help them quickly master the skills of using VSCode. In this article, we will introduce how to install VSCode, basic editing operations, shortcut keys, plug-in installation, etc., and provide readers with specific code examples. 1. Install VSCode first, we need
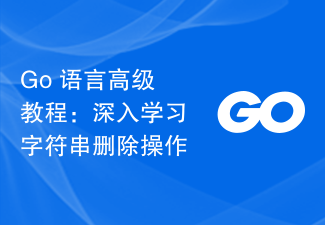
Go language is a very popular programming language, and its powerful features make it favored by many developers. String operations are one of the most common operations in programming, and in the Go language, string deletion operations are also very common. This article will delve into the string deletion operation in the Go language and use specific code examples to help you better understand and master this knowledge point. String deletion operation In the Go language, we usually use the strings package to perform string operations, including deletion operations.
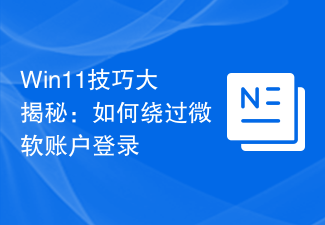
Win11 tricks revealed: How to bypass Microsoft account login Recently, Microsoft launched a new operating system Windows11, which has attracted widespread attention. Compared with previous versions, Windows 11 has made many new adjustments in terms of interface design and functional improvements, but it has also caused some controversy. The most eye-catching point is that it forces users to log in to the system with a Microsoft account. For some users, they may be more accustomed to logging in with a local account and are unwilling to bind their personal information to a Microsoft account.
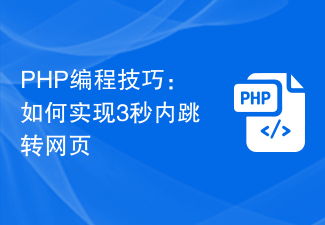
Title: PHP Programming Tips: How to Jump to a Web Page within 3 Seconds In web development, we often encounter situations where we need to automatically jump to another page within a certain period of time. This article will introduce how to use PHP to implement programming techniques to jump to a page within 3 seconds, and provide specific code examples. First of all, the basic principle of page jump is realized through the Location field in the HTTP response header. By setting this field, the browser can automatically jump to the specified page. Below is a simple example demonstrating how to use P
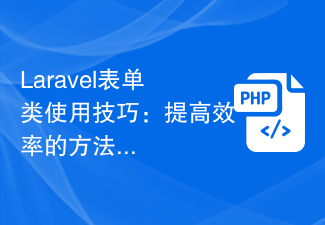
Forms are an integral part of writing a website or application. Laravel, as a popular PHP framework, provides rich and powerful form classes, making form processing easier and more efficient. This article will introduce some tips on using Laravel form classes to help you improve development efficiency. The following explains in detail through specific code examples. Creating a form To create a form in Laravel, you first need to write the corresponding HTML form in the view. When working with forms, you can use Laravel
