Binary tree algorithm in PHP and FAQs
With the continuous development of Web development, PHP, as a widely used server scripting language, its algorithms and data structures are becoming more and more important. Among these algorithms and data structures, the binary tree algorithm is a very important concept. This article will introduce the binary tree algorithm and its applications in PHP, as well as answers to common questions.
What is a binary tree?
Binary tree is a tree structure in which each node has at most two child nodes, namely the left child node and the right child node. If a node has no child nodes, it is called a leaf node. Binary trees are commonly used in search and sorting algorithms.
In PHP, you can use classes to implement binary trees. The following is an example binary tree node class:
class TreeNode { public $val; public $left; public $right; function __construct($val) { $this->val = $val; $this->left = null; $this->right = null; } }
In this TreeNode class, $val represents the value of the node, $left and $right represent the left child node and right child node of the node respectively.
How to build a binary tree?
In PHP, you can build a simple binary tree with the following code:
$root = new TreeNode(1); $root->left = new TreeNode(2); $root->right = new TreeNode(3); $root->left->left = new TreeNode(4); $root->left->right = new TreeNode(5);
This will create a binary tree whose root node has a value of 1 and whose left child node has a value of 2 , the value of its right child node is 3, the value of its left child node's left child node is 4, and the value of its left child node's right child node is 5.
How to traverse a binary tree?
There are usually three ways to traverse a binary tree: pre-order traversal, in-order traversal and post-order traversal.
Pre-order traversal refers to visiting the root node first, and then traversing the left subtree and right subtree. In PHP, pre-order traversal can be implemented through the following code:
function preorderTraversal($root) { if ($root == null) { return; } echo $root->val . " "; preorderTraversal($root->left); preorderTraversal($root->right); }
In-order traversal means first traversing the left subtree, then visiting the root node, and finally traversing the right subtree. In PHP, in-order traversal can be achieved through the following code:
function inorderTraversal($root) { if ($root == null) { return; } inorderTraversal($root->left); echo $root->val . " "; inorderTraversal($root->right); }
Post-order traversal means first traversing the left subtree and right subtree, and then visiting the root node. In PHP, post-order traversal can be achieved through the following code:
function postorderTraversal($root) { if ($root == null) { return; } postorderTraversal($root->left); postorderTraversal($root->right); echo $root->val . " "; }
How to find nodes in a binary tree?
To find nodes in a binary tree, you can use a recursive algorithm. The following is a sample code:
function search($root, $val) { if ($root == null || $root->val == $val) { return $root; } if ($val < $root->val) { return search($root->left, $val); } return search($root->right, $val); }
In this code, if the value of the node is equal to $val, the node is returned. Otherwise, if $val is less than the node's value, search in the left subtree. Otherwise, search in the right subtree.
How to insert a node into a binary tree?
To insert a node into a binary tree, you can use a recursive algorithm. The following is a sample code:
function insert($root, $val) { if ($root == null) { return new TreeNode($val); } if ($val < $root->val) { $root->left = insert($root->left, $val); } else { $root->right = insert($root->right, $val); } return $root; }
In this code, if the binary tree is empty, a new node is returned. Otherwise, if $val is less than the node's value, insert in the left subtree. Otherwise, insert in the right subtree.
How to delete nodes in a binary tree?
To delete a node in a binary tree, you need to consider the following three situations:
- The node to be deleted has no child nodes, you only need to delete the node directly.
- The node to be deleted has a child node, and the node needs to be replaced with this child node.
- The node to be deleted has two child nodes. You need to find the successor node of the node (that is, the smallest node in the right subtree of the node), replace the node with the successor node, and then delete the successor node.
The following is a sample code:
function deleteNode($root, $val) { if ($root == null) { return null; } if ($val < $root->val) { $root->left = deleteNode($root->left, $val); } else if ($val > $root->val) { $root->right = deleteNode($root->right, $val); } else { if ($root->left == null) { return $root->right; } else if ($root->right == null) { return $root->left; } $successor = $root->right; while ($successor->left != null) { $successor = $successor->left; } $root->val = $successor->val; $root->right = deleteNode($root->right, $successor->val); } return $root; }
Conclusion
The binary tree algorithm is a very important concept in PHP. Through recursive algorithms, various functions such as binary tree construction, traversal, node search, node insertion, and node deletion can be realized. Understanding these applications is very helpful for developing efficient web applications.
The above is the detailed content of Binary tree algorithm in PHP and FAQs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


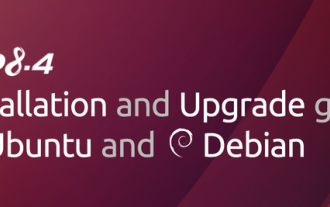
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
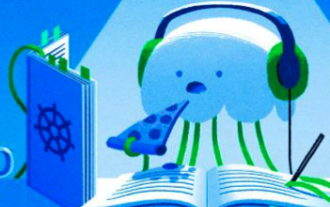
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
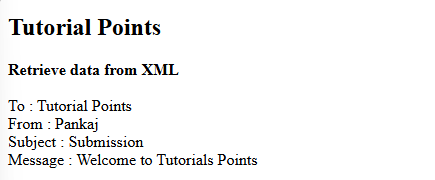
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
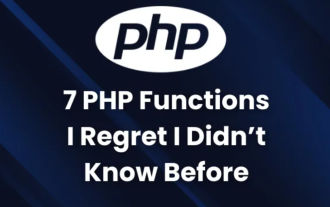
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
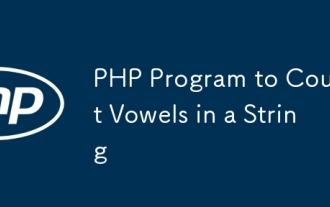
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
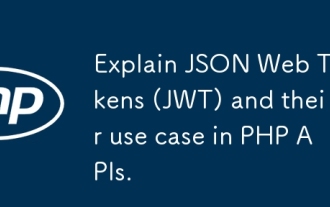
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
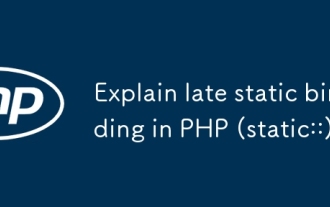
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
