PHP string processing methods and FAQs
PHP is a powerful server-side scripting language widely used for web development. In PHP, string processing is a very common operation. This article will introduce some PHP string processing methods and answer some common questions.
- String connection
You can use the "." symbol in PHP to connect two strings. For example:
$str1 = "Hello"; $str2 = "World"; $str3 = $str1 . $str2; echo $str3;
The output result is: HelloWorld
- String length
You can use the PHP built-in function strlen() to get the length of a string. For example:
$str = "Hello World"; $len = strlen($str); echo $len;
The output result is: 11
- String search
You can use the strpos() function to find whether a string contains another string. For example:
$str = "Hello World"; $pos = strpos($str, "World"); if ($pos !== false) { echo "Found"; } else { echo "Not Found"; }
The output result is: Found
- String replacement
You can use the str_replace() function to replace a certain section of a string into another string. For example:
$str = "Hello World"; $newStr = str_replace("World", "PHP", $str); echo $newStr;
The output result is: Hello PHP
- String splitting
You can use the explode() function to separate a string according to a certain symbols into arrays. For example:
$str = "Hello,World,PHP"; $arr = explode(",", $str); print_r($arr);
The output is:
Array ( [0] => Hello [1] => World [2] => PHP )
- FAQ
Q: How to insert a variable into a string?
A: You can use double quotes to enclose the string and add the "$" symbol before the variable. For example:
$name = "Tom"; $str = "Hello $name"; echo $str;
The output result is: Hello Tom
Q: How to insert special characters into a string?
A: You can use the escape character "\" to escape special characters. For example:
$str = "It is a "good" day"; echo $str;
The output result is: It is a "good" day
Q: How to remove spaces from the beginning and end of a string?
A: You can use the trim() function to remove leading and trailing spaces from a string. For example:
$str = " Hello World "; $newStr = trim($str); echo $newStr;
The output result is: Hello World
Q: How to convert a string to lowercase or uppercase?
A: You can use the strtolower() function to convert a string to lowercase, and the strtoupper() function to convert a string to uppercase. For example:
$str = "Hello World"; $newStr1 = strtolower($str); $newStr2 = strtoupper($str); echo $newStr1; echo $newStr2;
The output result is:
hello world HELLO WORLD
Summary
PHP is a powerful server-side scripting language, and string processing is a very common operation. This article introduces some methods of PHP string processing and answers some common questions. Mastering these techniques requires practice and practice.
The above is the detailed content of PHP string processing methods and FAQs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
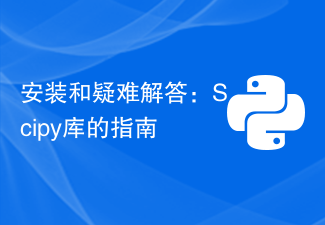
Scipy library installation tutorial and FAQ Introduction: Scipy (ScientificPython) is a Python library for numerical calculations, statistics, and scientific calculations. It is based on NumPy and can easily perform various scientific computing tasks such as array operations, numerical calculations, optimization, interpolation, signal processing, and image processing. This article will introduce the installation tutorial of Scipy library and answer some common questions. 1. Scipy installation tutorial Installation prerequisites Before installing Scipy, you need to
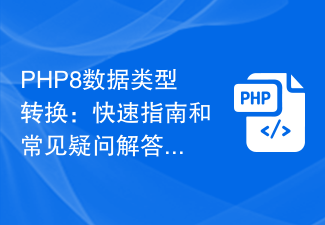
PHP8 Data Type Conversion: A Concise Guide and FAQ Overview: In PHP development, we often need to convert between data types. PHP8 provides us with many convenient data type conversion methods, which can easily convert between different data types and process data effectively. This article will provide you with a concise guide and FAQ, covering commonly used data type conversion methods and sample code in PHP8. Converting strings to integers When processing user input, database queries, etc., we often need to convert characters
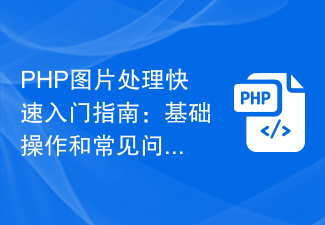
PHP Image Processing Quick Start Guide: Basic Operations and FAQs Introduction: In web development, image processing is a very common and important task. Whether it is used for image uploading, cropping, watermarking and other operations in website development, or used for image compression and processing in mobile applications, some operations need to be performed on images. As a popular server-side scripting language, PHP has powerful image processing capabilities. This article will help you quickly get started with PHP image processing, including basic operations and answers to frequently asked questions. 1. Basic exercises
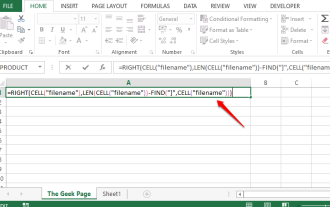
Excel does not provide a built-in formula to immediately return the name of the active Excel worksheet. However, in some cases, you may need to dynamically populate the values of the active worksheet in your Excel file. For example, if the table name on a worksheet must be the name of the worksheet itself, and if you hardcode the table name and later change the worksheet name, you must also change the table name manually. However, if the table's name is populated dynamically, such as using a formula, then if the sheet name changes, the table's name will also change automatically. As mentioned before, there is no direct formula for extracting the name of the active sheet, although the requirement is very possible. However, we do have some combinations of formulas that you can use to successfully extract the active sheet's
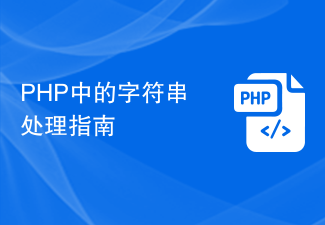
PHP is a popular programming language that is widely used in web application development. In PHP, string is a basic data type that allows us to store and manipulate text information. In this article, we will cover guidelines for string processing in PHP. Definition of string In PHP, a string is a sequence of adjacent characters, which can be represented by single quotes or double quotes. For example: $str1='Hello,world!';$str2="We
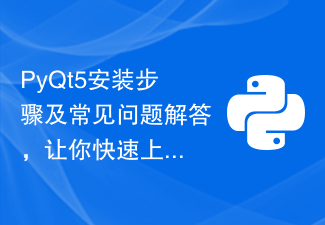
PyQt5 is a toolkit for developing graphical user interfaces in Python. It provides rich GUI components and functions that can help developers create interactive and visual applications quickly and easily. This article will introduce the installation steps of PyQt5 and answer some common questions to help readers get started quickly. 1. Install PyQt5 Install Python: PyQt5 is a Python library. First, you need to install Python on your computer. You can download it from the Python official website (htt
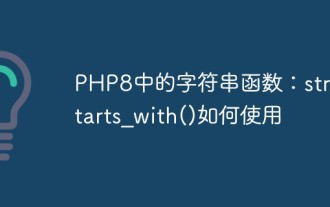
A new practical string function str_starts_with() has been added to PHP8. This article will introduce the introduction, usage and examples of this function. Introduction to str_starts_with() The str_starts_with() function can determine whether a string starts with another string and return a Boolean value. The syntax is as follows: str_starts_with(string$haystack,string$nee
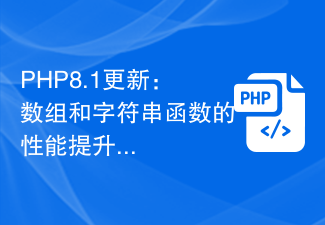
PHP8.1 update: Performance improvements for array and string functions The PHP programming language has continued to evolve and improve over time. The recently released version of PHP 8.1 brings many new features and performance enhancements, especially when it comes to array and string functions. These improvements allow developers to handle array and string operations more efficiently, improving overall performance and efficiency. Performance improvements of array functions In PHP8.1, array functions have been improved and optimized. Here are some important examples of performance improvements for array functions: (1
