


A complete guide to implementing login authentication in Vue.js (API, JWT, axios)
Vue.js is a popular JavaScript framework for building dynamic web applications. Implementing user login authentication is one of the necessary parts of developing web applications. This article will introduce a complete guide to implementing login verification using Vue.js, API, JWT and axios.
- Creating a Vue.js application
First, we need to create a new Vue.js application. We can create a Vue.js application using the Vue CLI or manually.
- Install axios
axios is a simple and easy-to-use HTTP client for making HTTP requests. We can install axios using npm:
npm install axios --save
- Create API
We need to create an API to handle user login requests. Create APIs using Node.js and Express.js. Here is a basic API example:
const express = require('express'); const router = express.Router(); const jwt = require('jsonwebtoken'); router.post('/login', function(req, res) { // TODO: 根据请求的用户名和密码查询用户 const user = {id: 1, username: 'test', password: 'password'}; // 如果用户不存在或密码不正确则返回401 if (!user || req.body.password !== user.password) { return res.status(401).json({message: '用户名或密码错误'}); } const token = jwt.sign({sub: user.id}, 'secret'); res.json({token: token}); }); module.exports = router;
In this example, we use JWT to create a JWT token that will be used during user authentication process. We use a password to authenticate the user and issue a token to the user once the user is authenticated.
- Integrate API and axios
Now we need to integrate the API and axios so that the Vue.js application can communicate with the backend. We will create a service using axios.
import axios from 'axios'; class AuthService { constructor() { this.http = axios.create({ baseURL: process.env.API_URL }); } login(username, password) { return this.http.post('/login', {username, password}) .then(response => { if (response.data.token) { localStorage.setItem('token', response.data.token); } return Promise.resolve(response.data); }); } logout() { localStorage.removeItem('token'); return Promise.resolve(); } isLoggedIn() { const token = localStorage.getItem('token'); return !!token; } } export default AuthService;
In this example, we create a service called "AuthService" that uses axios to access the API and communicate with the backend. We use LocalStorage to store the authentication token and check the login status based on the authentication token.
- Creating a Vue.js component
Now, we need to create a Vue.js component that handles user authentication and renders the login page. In this example, we will create a component called "SignIn".
<template> <div> <h2>登录</h2> <form v-on:submit.prevent="handleSubmit"> <div> <label for="username">用户名</label> <input id="username" type="text" v-model="username"/> </div> <div> <label for="password">密码</label> <input id="password" type="password" v-model="password"/> </div> <button type="submit">提交</button> </form> </div> </template> <script> import AuthService from './services/AuthService'; export default { name: 'SignIn', data() { return { username: '', password: '' }; }, methods: { handleSubmit() { AuthService.login(this.username, this.password) .then(() => { this.$router.push('/'); }) .catch(error => { console.log(error); }); } } }; </script>
In this example, we create a component called "SignIn" and bind a handler method called "handleSubmit" on the component. This method calls the "login" method of the "AuthService" service, which makes an API request based on the provided username and password.
6. Routing configuration
Now, we need to configure the Vue.js routing so that our SignIn component can be displayed on the routing path.
import Vue from 'vue'; import VueRouter from 'vue-router'; import SignIn from './SignIn.vue'; import Home from './Home.vue'; Vue.use(VueRouter); const routes = [ {path: '/login', component: SignIn}, {path: '/', component: Home} ]; const router = new VueRouter({ routes }); export default router;
In this example, our routing configuration includes two paths: /login and /. We bind the SignIn component to the /login path and the Home component to the / path.
- Configuring the Vue.js application
Finally, we need to configure the Vue.js application and add the Vue.js routing component to the Vue.js application template middle.
import Vue from 'vue'; import App from './App.vue'; import router from './router'; Vue.config.productionTip = false; new Vue({ router, render: h => h(App) }).$mount('#app');
In this example, we create a Vue instance using the "new Vue" statement of Vue.js and render the App.vue component using the render function "h" of Vue.js and the Vue.js router Passed to the Vue instance.
Conclusion
This article presents a complete guide on how to implement user login verification using Vue.js, axios and API. We created an API using Node.js and Express.js, created the service using JWT and axios, and created Vue.js components to handle user authentication and render the login page. After configuring the routing, we can use the Vue.js application and authenticate user login.
The above is the detailed content of A complete guide to implementing login authentication in Vue.js (API, JWT, axios). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


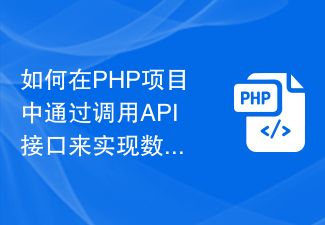
How to crawl and process data by calling API interface in PHP project? 1. Introduction In PHP projects, we often need to crawl data from other websites and process these data. Many websites provide API interfaces, and we can obtain data by calling these interfaces. This article will introduce how to use PHP to call the API interface to crawl and process data. 2. Obtain the URL and parameters of the API interface. Before starting, we need to obtain the URL of the target API interface and the required parameters.
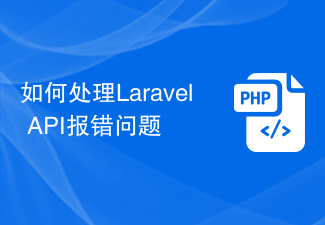
Title: How to deal with Laravel API error problems, specific code examples are needed. When developing Laravel, API errors are often encountered. These errors may come from various reasons such as program code logic errors, database query problems, or external API request failures. How to handle these error reports is a key issue. This article will use specific code examples to demonstrate how to effectively handle Laravel API error reports. 1. Error handling in Laravel
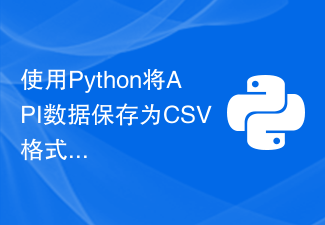
In the world of data-driven applications and analytics, APIs (Application Programming Interfaces) play a vital role in retrieving data from various sources. When working with API data, you often need to store the data in a format that is easy to access and manipulate. One such format is CSV (Comma Separated Values), which allows tabular data to be organized and stored efficiently. This article will explore the process of saving API data to CSV format using the powerful programming language Python. By following the steps outlined in this guide, we will learn how to retrieve data from the API, extract relevant information, and store it in a CSV file for further analysis and processing. Let’s dive into the world of API data processing with Python and unlock the potential of the CSV format
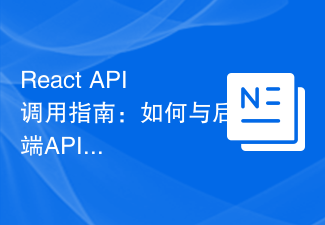
ReactAPI Call Guide: How to interact with and transfer data to the backend API Overview: In modern web development, interacting with and transferring data to the backend API is a common need. React, as a popular front-end framework, provides some powerful tools and features to simplify this process. This article will introduce how to use React to call the backend API, including basic GET and POST requests, and provide specific code examples. Install the required dependencies: First, make sure Axi is installed in the project
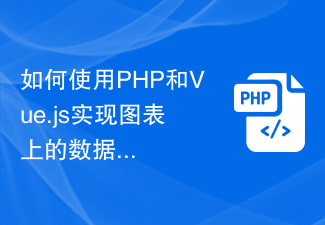
How to use PHP and Vue.js to implement data filtering and sorting functions on charts. In web development, charts are a very common way of displaying data. Using PHP and Vue.js, you can easily implement data filtering and sorting functions on charts, allowing users to customize the viewing of data on charts, improving data visualization and user experience. First, we need to prepare a set of data for the chart to use. Suppose we have a data table that contains three columns: name, age, and grades. The data is as follows: Name, Age, Grades Zhang San 1890 Li
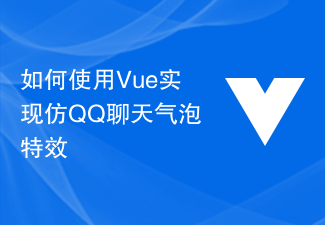
How to use Vue to implement QQ-like chat bubble effects In today’s social era, the chat function has become one of the core functions of mobile applications and web applications. One of the most common elements in the chat interface is the chat bubble, which can clearly distinguish the sender's and receiver's messages, effectively improving the readability of the message. This article will introduce how to use Vue to implement QQ-like chat bubble effects and provide specific code examples. First, we need to create a Vue component to represent the chat bubble. The component consists of two main parts
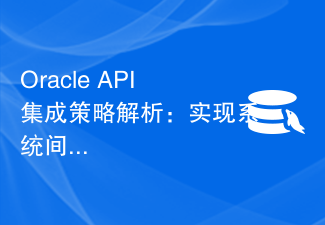
OracleAPI integration strategy analysis: To achieve seamless communication between systems, specific code examples are required. In today's digital era, internal enterprise systems need to communicate with each other and share data, and OracleAPI is one of the important tools to help achieve seamless communication between systems. This article will start with the basic concepts and principles of OracleAPI, explore API integration strategies, and finally give specific code examples to help readers better understand and apply OracleAPI. 1. Basic Oracle API
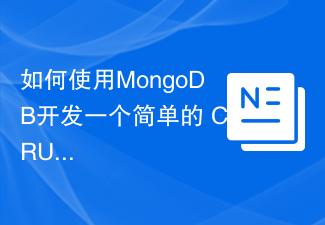
How to use MongoDB to develop a simple CRUD API In modern web application development, CRUD (Create, Delete, Modify, Check) operations are one of the most common and important functions. In this article, we will introduce how to develop a simple CRUD API using MongoDB database and provide specific code examples. MongoDB is an open source NoSQL database that stores data in the form of documents. Unlike traditional relational databases, MongoDB does not have a predefined schema
