


A complete guide to implementing component communication in Vue2.x (props, $emit, Vuex)
Vue2.x Complete Guide to Implementing Component Communication (props, $emit, Vuex)
Vue, as a modern JavaScript framework, is very popular when developing Web applications. Vue's componentized architecture allows developers to easily separate code and functionality, while also enabling flexible communication through different components.
In this article, we will explore three methods of component communication in Vue2.x: props, $emit and Vuex, to help you better manage resources when building Vue applications.
Props
props is one of Vue’s component parameter passing methods. Values can be passed to child components using props. In child components, the values of props are read-only, which means they cannot be modified. This enables one-way flow of data, making it easier to maintain and debug Vue applications.
Here is an example:
In the parent component, we can create a prop named "parent" and pass it a prop named "message".
<template> <div> <child :message="msg"></child> </div> </template> <script> import Child from "./Child.vue"; export default { name: "Parent", components: { Child }, data() { return { msg: "Hello World!" }; } }; </script>
In the child component, we can receive the passed props value and use it in the template.
<template> <div> {{ message }} </div> </template> <script> export default { name: "Child", props: { message: { type: String, required: true } } }; </script>
In this example, "msg" is defined in the parent component and "message" is the name of the props - which must match the value in the parent component. Child components must use the "props" option to define the data type of the props and the values that must be passed.
This is a basic example for data passing through props. If you have multiple props that need to be passed, you can put them in an object and pass them to the child component.
$emit
$emit is another widely used component communication method in Vue. You can use $emit to trigger custom events and pass data to the parent component. Unlike props, $emit can achieve two-way data transfer, making resource sharing between components in Vue applications more convenient.
Unlike props, $emit can pass data from child components to parent components. Here is an example:
In this example, we define a custom event name "greeting" and fire the event when a button is clicked. We also pass the selected item into the event.
<template> <div> <button @click="sayHi()">Click me</button> </div> </template> <script> export default { name: "Child", methods: { sayHi() { this.$emit("greeting", { message: "Hello World!" }); } } }; </script>
In the parent component, we can listen to custom events in the child component and use the passed data when the event fires.
<template> <div> <child @greeting="handleGreeting"></child> <div>{{ greeting }}</div> </div> </template> <script> import Child from "./Child.vue"; export default { name: "Parent", components: { Child }, data() { return { greeting: "" }; }, methods: { handleGreeting(data) { this.greeting = data.message; } } }; </script>
In this example, "handleGreeting" is the method used to handle the event. This method receives as parameter a custom event triggered by the child component. Data transfer can be obtained from the $emit event in the child component.
Vuex
Vuex is a state management library for Vue.js applications. It allows components to share state, making communication between components easier and more efficient.
Here is an example:
In this example, we share data by creating a Vuex store named "store". In the state attribute, we can define the data that needs to be shared. In the mutations attribute, we can define functions for modifying data in the store. In the getter attribute, we can define functions that process the data and return the shared value.
import Vue from "vue"; import Vuex from "vuex"; Vue.use(Vuex); export default new Vuex.Store({ state: { greeting: "Hello World!" }, mutations: { changeGreeting(state, payload) { state.greeting = payload.greeting; } }, getters: { getGreeting(state) { return state.greeting; } } });
You can use data and functions in the store in any Vue component. In this example, we have two buttons set up. Clicking the "greeting" button will display the value of the "greeting" attribute in the store. The "change greeting" button will change the "greeting" value in the store by calling the function we defined in the mutations attribute through the commit function.
<template> <div> <div>{{ greeting }}</div> <button @click="getGreeting">greeting</button> <button @click="changeGreeting">change greeting</button> </div> </template> <script> import { mapGetters, mapMutations } from "vuex"; export default { name: "Child", computed: { ...mapGetters(["getGreeting"]) }, methods: { ...mapMutations(["changeGreeting"]), getGreeting() { alert(this.getGreeting); } } }; </script>
In this example, "mapGetters" and "mapMutations" can be used to map data and functions in the store to the component's computed properties and methods. In the method, we use alert to display the value of the "greeting" attribute in the store. When the "change greeting" button is clicked the changeGreeting function will be called to change the "greeting" attribute in the store.
Summary
The above are the three methods to implement component communication in Vue2.x: props, $emit and Vuex. In actual development, you can choose which communication method to use based on different needs and scenarios.
Through props, one-way data transmission can be achieved to ensure that the data flow between components is clear and clear; $emit can perform two-way data transmission between components, so that the resources between components in the Vue application Sharing is more convenient; and by using Vuex, public data can be saved in the store, making communication between components easier and more efficient.
The above is the detailed content of A complete guide to implementing component communication in Vue2.x (props, $emit, Vuex). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


![How to solve the problem 'Error: [vuex] unknown action type: xxx' when using vuex in a Vue application?](https://img.php.cn/upload/article/000/887/227/168766615217161.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
In Vue.js projects, vuex is a very useful state management tool. It helps us share state among multiple components and provides a reliable way to manage state changes. But when using vuex, sometimes you will encounter the error "Error:[vuex]unknownactiontype:xxx". This article will explain the cause and solution of this error. 1. Cause of the error When using vuex, we need to define some actions and mu
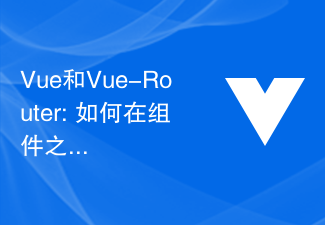
Vue and Vue-Router: How to share data between components? Introduction: Vue is a popular JavaScript framework for building user interfaces. Vue-Router is Vue's official routing manager, used to implement single-page applications. In Vue applications, components are the basic units for building user interfaces. In many cases we need to share data between different components. This article will introduce some methods to help you achieve data sharing in Vue and Vue-Router, and
![How to solve the problem 'Error: [vuex] do not mutate vuex store state outside mutation handlers.' when using vuex in a Vue application?](https://img.php.cn/upload/article/000/000/164/168760467048976.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
In Vue applications, using vuex is a common state management method. However, when using vuex, we may sometimes encounter such an error message: "Error:[vuex]donotmutatevuexstorestateoutsidemutationhandlers." What does this error message mean? Why does this error message appear? How to fix this error? This article will cover this issue in detail. The error message contains
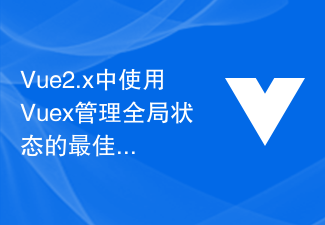
Vue2.x is one of the most popular front-end frameworks currently, which provides Vuex as a solution for managing global state. Using Vuex can make state management clearer and easier to maintain. The best practices of Vuex will be introduced below to help developers better use Vuex and improve code quality. 1. Use modular organization state. Vuex uses a single state tree to manage all the states of the application, extracting the state from the components, making state management clearer and easier to understand. In applications with a lot of state, modules must be used
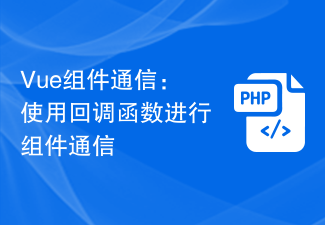
Vue component communication: using callback functions for component communication In Vue applications, sometimes we need to let different components communicate with each other so that they can share information and collaborate with each other. Vue provides a variety of ways to implement communication between components, one of the common ways is to use callback functions. A callback function is a mechanism in which a function is passed as an argument to another function and is called when a specific event occurs. In Vue, we can use callback functions to implement communication between components, so that a component can
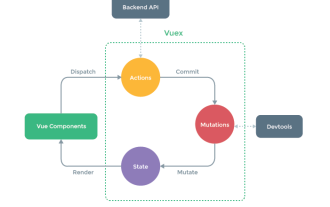
What does Vuex do? Vue official: State management tool What is state management? State that needs to be shared among multiple components, and it is responsive, one change, all changes. For example, some globally used status information: user login status, user name, geographical location information, items in the shopping cart, etc. At this time, we need such a tool for global status management, and Vuex is such a tool. Single-page state management View–>Actions—>State view layer (view) triggers an action (action) to change the state (state) and responds back to the view layer (view) vuex (Vue3.
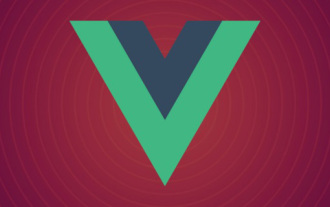
In the project we write vue3, we will all communicate with components. In addition to using the pinia public data source, what simpler API methods can we use? Next, I will introduce to you several ways of communicating between parent-child components and child-parent components.
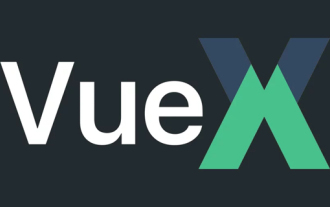
When asked in an interview about the implementation principle of vuex, how should you answer? The following article will give you an in-depth understanding of the implementation principle of vuex. I hope it will be helpful to you!
