A Complete Guide to Implementing Infinite Scroll Loading with Vue.js
As the amount of data continues to increase, scrolling loading of web pages has gradually become an important part of the user experience. In this post, we will discuss a complete guide on how to implement infinite scroll loading using Vue.js.
What is infinite scroll loading?
Infinite scroll loading, also known as infinite scroll, is a web design technique used to add more content as the user scrolls to the bottom of the page. This technology is commonly used on blogs, social media, online stores and other websites that need to display content dynamically.
Infinite scrolling is different from paging. In traditional paging, users must turn pages to load the content of the next page. In infinite scrolling, the page will automatically load the content of the next page, and users can continue to browse the list without turning pages.
What is Vue.js?
Vue.js is a lightweight JavaScript framework for building user interfaces. It has good scalability and maintainability, and is easy to integrate into existing projects. Vue.js provides many useful features, such as two-way data binding, componentized architecture, and virtual DOM, making developing web applications faster and easier.
Now, we start to explore how to use Vue.js to achieve infinite scroll loading.
Step 1: Prepare the project
First, we need to set up the Vue.js project through Node.js and npm. Then, create a Vue.js component to display our list items.
Install Vue.js and prepare the project:
npm install -g vue-cli vue init webpack my-project cd my-project npm install
Create components:
You can use the following command to create components:
vue generate component List
Step 2: Implementation Infinite Scroll
Here’s the most important part: how to achieve infinite scroll.
Suppose we have an API that requires pagination, and it returns one page of data and the URL address of the next page.
To achieve infinite scrolling, we need to load the next page of data until no more data is available or the user stops scrolling the page. We can use Vue.js' watch
API to listen for scroll events and trigger an event to load the next page when scrolling to the bottom of the page.
In the component we created previously, add the following code:
<template> <div> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> export default { data() { return { items: [], nextUrl: "https://api.example.com/page1" }; }, watch: { $route(to, from) { window.addEventListener("scroll", this.handleScroll); } }, methods: { async loadMore() { const response = await fetch(this.nextUrl); const data = await response.json(); this.items = [...this.items, ...data.items]; this.nextUrl = data.nextUrl; }, handleScroll() { if ( window.innerHeight + window.pageYOffset >= document.body.offsetHeight ) { this.loadMore(); } } }, mounted() { window.addEventListener("scroll", this.handleScroll); }, destroyed() { window.removeEventListener("scroll", this.handleScroll); } }; </script>
We first defined two data items: items
and nextUrl
, items
is used to store the loaded list items, and nextUrl
is used to store the URL address of the next page.
In the mounted
life cycle hook, we bind the handleScroll
method to the scroll event.
We used the watch
API to listen for route change events, here to rebind the scroll event when the component is reused.
loadMore
method is used to load the data of the next page. We use the fetch
API to get the data and add it to the items
array. The handleScroll
method is used to check the scroll event. When the page scrolls to the bottom, the loadMore
method is called to load the data of the next page.
Finally, we release the monitoring of scroll events when the component is destroyed.
Now, we have completed the implementation of infinite scrolling. Whenever the user scrolls to the bottom of the page, the data for the next page loads automatically.
Step 3: Add loading prompts and error prompts
Better user experience usually requires adding a loading prompt at the end of the list, and also needs to display an error prompt when an error occurs.
Add the following code in the previous component:
<template> <div> <ul> <li v-for="item in items" :key="item.id">{{ item.name }}</li> </ul> <div v-if="isLoading">Loading...</div> <div v-if="errorMessage">{{ errorMessage }}</div> </div> </template> <script> export default { data() { return { items: [], nextUrl: "https://api.example.com/page1", isLoading: false, errorMessage: null }; }, watch: { $route(to, from) { window.addEventListener("scroll", this.handleScroll); } }, methods: { async loadMore() { try { this.isLoading = true; const response = await fetch(this.nextUrl); const data = await response.json(); this.items = [...this.items, ...data.items]; this.nextUrl = data.nextUrl; } catch (error) { this.errorMessage = "Something went wrong." + error.message; } finally { this.isLoading = false; } }, handleScroll() { if ( window.innerHeight + window.pageYOffset >= document.body.offsetHeight ) { this.loadMore(); } } }, mounted() { window.addEventListener("scroll", this.handleScroll); }, destroyed() { window.removeEventListener("scroll", this.handleScroll); } }; </script>
We added two data items isLoading
and errorMessage
, which are used to display the loading respectively. Hints and error messages. In the loadMore
method, we add a try-catch block to catch errors that may occur when data is loaded, and close the loading prompt in the finally block. Display the error message in errorMessage
.
Now, we have completed an infinite scrolling list with loading prompts and error prompts.
Conclusion
In this article, we learned how to use Vue.js to implement infinite scrolling loading list. We learned about important technologies such as monitoring scroll events, dynamically loading data, display loading and error prompts.
When implementing infinite scrolling, we need to be careful not to load too much data at once, which will cause performance degradation. We should also provide users with appropriate loading prompts and error prompts to improve user experience.
Of course, this is just a simple example. Vue.js provides more functions and APIs, allowing us to create more complex components and applications. Hope this article can be helpful to you.
The above is the detailed content of A Complete Guide to Implementing Infinite Scroll Loading with Vue.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
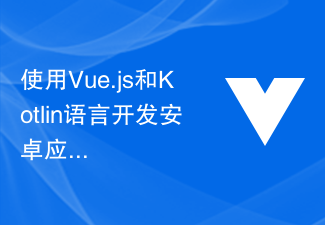
Some tips for developing Android applications using Vue.js and Kotlin language. With the popularity of mobile applications and the continuous growth of user needs, the development of Android applications has attracted more and more attention from developers. When developing Android apps, choosing the right technology stack is crucial. In recent years, Vue.js and Kotlin languages have gradually become popular choices for Android application development. This article will introduce some techniques for developing Android applications using Vue.js and Kotlin language, and give corresponding code examples. 1. Set up the development environment at the beginning
![Error loading plugin in Illustrator [Fixed]](https://img.php.cn/upload/article/000/465/014/170831522770626.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
When launching Adobe Illustrator, does a message about an error loading the plug-in pop up? Some Illustrator users have encountered this error when opening the application. The message is followed by a list of problematic plugins. This error message indicates that there is a problem with the installed plug-in, but it may also be caused by other reasons such as a damaged Visual C++ DLL file or a damaged preference file. If you encounter this error, we will guide you in this article to fix the problem, so continue reading below. Error loading plug-in in Illustrator If you receive an "Error loading plug-in" error message when trying to launch Adobe Illustrator, you can use the following: As an administrator
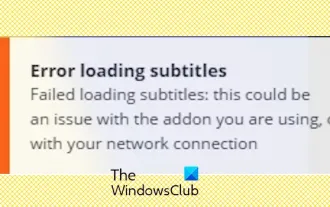
Subtitles not working on Stremio on your Windows PC? Some Stremio users reported that subtitles were not displayed in the videos. Many users reported encountering an error message that said "Error loading subtitles." Here is the full error message that appears with this error: An error occurred while loading subtitles Failed to load subtitles: This could be a problem with the plugin you are using or your network. As the error message says, it could be your internet connection that is causing the error. So please check your network connection and make sure your internet is working properly. Apart from this, there could be other reasons behind this error, including conflicting subtitles add-on, unsupported subtitles for specific video content, and outdated Stremio app. like
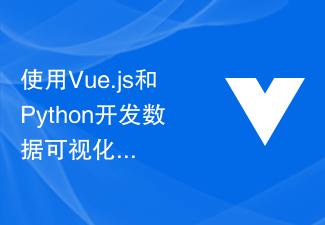
Some tips for developing data visualization applications using Vue.js and Python Introduction: With the advent of the big data era, data visualization has become an important solution. In the development of data visualization applications, the combination of Vue.js and Python can provide flexibility and powerful functions. This article will share some tips for developing data visualization applications using Vue.js and Python, and attach corresponding code examples. 1. Introduction to Vue.js Vue.js is a lightweight JavaScript
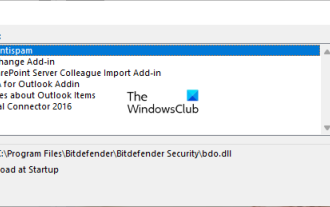
If you encounter freezing issues when inserting hyperlinks into Outlook, it may be due to unstable network connections, old Outlook versions, interference from antivirus software, or add-in conflicts. These factors may cause Outlook to fail to handle hyperlink operations properly. Fix Outlook freezes when inserting hyperlinks Use the following fixes to fix Outlook freezes when inserting hyperlinks: Check installed add-ins Update Outlook Temporarily disable your antivirus software and then try creating a new user profile Fix Office apps Program Uninstall and reinstall Office Let’s get started. 1] Check the installed add-ins. It may be that an add-in installed in Outlook is causing the problem.
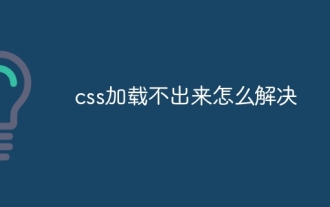
The solutions to the problem that CSS cannot be loaded include checking the file path, checking the file content, clearing the browser cache, checking the server settings, using developer tools and checking the network connection. Detailed introduction: 1. Check the file path. First, please make sure the path of the CSS file is correct. If the CSS file is located in a different part or subdirectory of the website, you need to provide the correct path. If the CSS file is located in the root directory, the path should be direct. ; 2. Check the file content. If the path is correct, the problem may lie in the CSS file itself. Open the CSS file to check, etc.
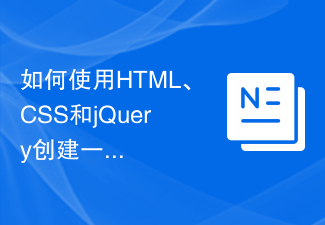
How to create an infinite scrolling news list using HTML, CSS and jQuery In web development, infinite scrolling is a common interaction technology, especially suitable for pages such as news lists that need to load a large amount of data. This article will introduce how to use HTML, CSS and jQuery to implement an infinite scrolling news list, and provide specific code examples. First, we need a basic HTML structure to display the news list. Here's a simple example: <!DOCTYPE
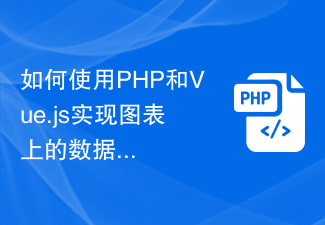
How to use PHP and Vue.js to implement data filtering and sorting functions on charts. In web development, charts are a very common way of displaying data. Using PHP and Vue.js, you can easily implement data filtering and sorting functions on charts, allowing users to customize the viewing of data on charts, improving data visualization and user experience. First, we need to prepare a set of data for the chart to use. Suppose we have a data table that contains three columns: name, age, and grades. The data is as follows: Name, Age, Grades Zhang San 1890 Li
