


PCA principal component analysis (dimensionality reduction) techniques in Python
PCA principal component analysis (dimensionality reduction) skills in Python
PCA (Principal Component Analysis) principal component analysis is a very commonly used data dimensionality reduction technology. The PCA algorithm can be used to process data to discover the inherent characteristics of the data and provide a more accurate and effective data collection for subsequent data analysis and modeling.
Below we will introduce some techniques for using PCA principal component analysis in Python.
- How to normalize data
Before performing PCA dimensionality reduction analysis, you first need to normalize the data. This is because the PCA algorithm calculates the principal components through variance maximization, rather than simply the size of the element values, so it fully takes into account the impact of the corresponding variance of each element.
There are many methods in Python for data normalization. The most basic method is to standardize the data into a standard normal distribution with a mean of 0 and a variance of 1 through the StandardScaler class of the sklearn library. The code is as follows:
from sklearn.preprocessing import StandardScaler scaler = StandardScaler() data_std = scaler.fit_transform(data)
In this way, we can get a data that has been normalized The processed data collection data_std.
- Using PCA for dimensionality reduction
The code for using PCA to reduce the dimensionality of data is very simple. The PCA module has been integrated in the sklearn library. We only need to set the number of principal components retained after dimensionality reduction when calling the PCA class. For example, the following code reduces the data to 2 principal components:
from sklearn.decomposition import PCA pca = PCA(n_components=2) data_pca = pca.fit_transform(data_std)
Among them, data_pca returns the new data after PCA dimensionality reduction processing.
- How to choose the number of principal components after dimensionality reduction
When actually using PCA for data dimensionality reduction, we need to choose the appropriate number of principal components to achieve the best Dimensionality reduction effect. Usually, we can judge by plotting the cumulative variance contribution rate graph.
The cumulative variance contribution rate represents the percentage of the sum of the variances of the first n principal components to the total variance, for example:
import numpy as np pca = PCA() pca.fit(data_std) cum_var_exp = np.cumsum(pca.explained_variance_ratio_)
By drawing the cumulative variance contribution rate graph, we can observe the number of principal components The changing trend of the cumulative variance contribution rate when gradually increasing from 1 to estimate the appropriate number of principal components. The code is as follows:
import matplotlib.pyplot as plt plt.bar(range(1, 6), pca.explained_variance_ratio_, alpha=0.5, align='center') plt.step(range(1, 6), cum_var_exp, where='mid') plt.ylabel('Explained variance ratio') plt.xlabel('Principal components') plt.show()
The red line in the figure represents the cumulative variance contribution rate, the x-axis represents the number of principal components, and the y-axis represents the proportion of variance explained. It can be found that the variance contribution rate of the first two principal components is close to 1, so selecting two principal components can meet the needs of most analysis tasks.
- How to visualize the data after PCA dimensionality reduction
Finally, we can use the scatter function of the matplotlib library to visualize the data after PCA dimensionality reduction. For example, the following code reduces the data from the original 4 dimensions to 2 dimensions through PCA, and then displays it visually:
import matplotlib.pyplot as plt x = data_pca[:, 0] y = data_pca[:, 1] labels = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9'] colors = ['b', 'g', 'r', 'c', 'm', 'y', 'k', 'pink', 'brown', 'orange'] for i, label in enumerate(np.unique(labels)): plt.scatter(x[labels == label], y[labels == label], c=colors[i], label=label, alpha=0.7) plt.legend() plt.xlabel('Principal Component 1') plt.ylabel('Principal Component 2') plt.show()
The colors and labels in the figure correspond to the numerical labels in the original data respectively. Through visualization With dimensionally reduced data, we can better understand the structure and characteristics of the data.
In short, using PCA principal component analysis technology can help us reduce the dimensionality of the data and thereby better understand the structure and characteristics of the data. Through Python's sklearn and matplotlib libraries, we can implement and visualize the PCA algorithm very conveniently.
The above is the detailed content of PCA principal component analysis (dimensionality reduction) techniques in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


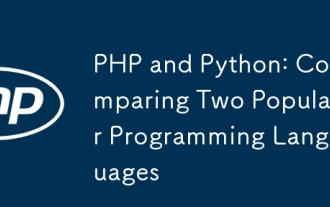
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
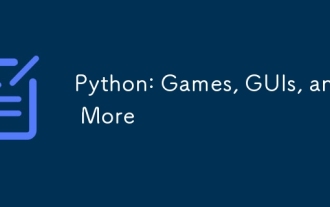
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
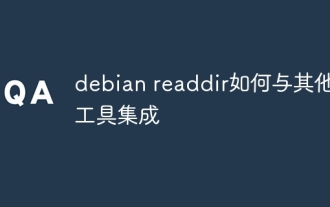
The readdir function in the Debian system is a system call used to read directory contents and is often used in C programming. This article will explain how to integrate readdir with other tools to enhance its functionality. Method 1: Combining C language program and pipeline First, write a C program to call the readdir function and output the result: #include#include#include#includeintmain(intargc,char*argv[]){DIR*dir;structdirent*entry;if(argc!=2){
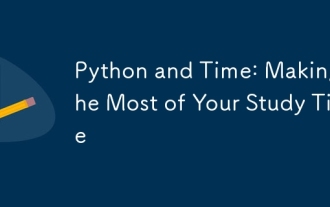
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
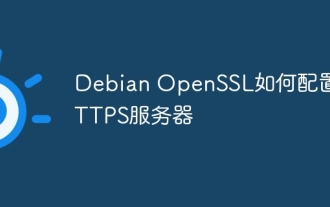
Configuring an HTTPS server on a Debian system involves several steps, including installing the necessary software, generating an SSL certificate, and configuring a web server (such as Apache or Nginx) to use an SSL certificate. Here is a basic guide, assuming you are using an ApacheWeb server. 1. Install the necessary software First, make sure your system is up to date and install Apache and OpenSSL: sudoaptupdatesudoaptupgradesudoaptinsta
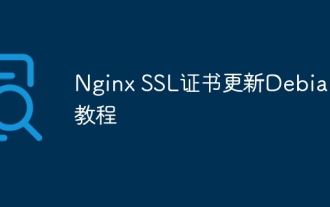
This article will guide you on how to update your NginxSSL certificate on your Debian system. Step 1: Install Certbot First, make sure your system has certbot and python3-certbot-nginx packages installed. If not installed, please execute the following command: sudoapt-getupdatesudoapt-getinstallcertbotpython3-certbot-nginx Step 2: Obtain and configure the certificate Use the certbot command to obtain the Let'sEncrypt certificate and configure Nginx: sudocertbot--nginx Follow the prompts to select
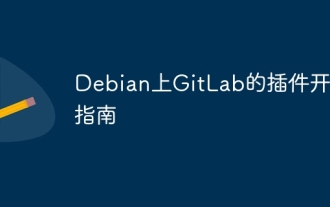
Developing a GitLab plugin on Debian requires some specific steps and knowledge. Here is a basic guide to help you get started with this process. Installing GitLab First, you need to install GitLab on your Debian system. You can refer to the official installation manual of GitLab. Get API access token Before performing API integration, you need to get GitLab's API access token first. Open the GitLab dashboard, find the "AccessTokens" option in the user settings, and generate a new access token. Will be generated
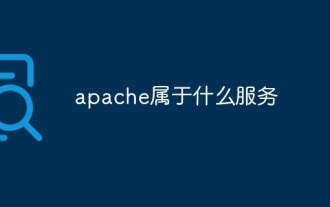
Apache is the hero behind the Internet. It is not only a web server, but also a powerful platform that supports huge traffic and provides dynamic content. It provides extremely high flexibility through a modular design, allowing for the expansion of various functions as needed. However, modularity also presents configuration and performance challenges that require careful management. Apache is suitable for server scenarios that require highly customizable and meet complex needs.
