Why is exception handling in my Go program not working?
Golang (Go) is a language that is very good at handling errors and exceptions. Unlike other languages, Go handles exceptions through a simple yet effective error handling mechanism. Although Go's error handling mechanism is very powerful and flexible, some programmers still have trouble implementing error handling in their programs. This article is intended to help address the question of why exception handling in Go programs doesn't work, and how to handle exception situations correctly.
Invalid exception handling in Go is usually caused by programmers not handling errors correctly or misunderstanding Go's error handling mechanism. Below are some common error handling problems and solutions.
1. Use panic instead of errors
Although panic is an effective handler, using too much in some cases can cause the program to crash. When an error occurs while the application is running, it should first attempt to handle the error and return an error. When a programmer uses panic in place of an error, there is nothing the programmer can do to resolve or avoid the error and may cause the application to break. Instead, if you use an error handler, it will be easier to handle errors than using panic directly.
2. Assume no errors
Some programmers may assume that errors may not occur, or that even if errors occur, they will not affect the operation of the program. This assumption may lead to overlooking the importance of error handling. In Go, all functions and operations can cause errors, so error handling must always be taken into account.
3. Ignore the return value
Go functions usually return a value and an error. If the programmer ignores the return value, there is no way to obtain error information and handle the error. The error information returned after the function call must be checked so that errors can be handled promptly.
4. Unsure of the type of error
In Go, errors are usually interfaces of type error. If the programmer doesn't know the type of error, he can't handle it correctly. Therefore, you must always check the documentation to understand the error types returned and their possible values in order to handle errors correctly.
5. Incomplete error handling
Error handling must handle all possible error conditions. If the programmer only handles part of the error, it will cause the program to be abnormal and not work properly. Therefore, you must ensure that your application fully handles all possible error conditions.
Therefore, we can ensure the effectiveness of the error handling mechanism through the following measures:
1. Use return error values instead of panic statements to handle errors.
2. Consider error situations as much as possible and don't assume there are no errors.
3. Always check the error value returned by the function and ensure that each error condition is handled correctly.
4. Learn and understand Go language error types and error values, and handle each error correctly in the program.
5. Ensure error handling is complete and handle all possible error conditions.
In short, error handling is a crucial aspect in Go programming. Ignored or ineffective error handling can cause code to become unstable and important information can be lost. Therefore, programmers must always handle errors carefully to ensure the stability and correctness of the program.
The above is the detailed content of Why is exception handling in my Go program not working?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
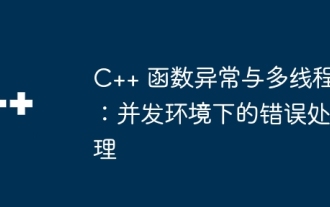
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
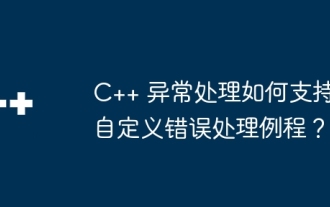
C++ exception handling allows the creation of custom error handling routines to handle runtime errors by throwing exceptions and catching them using try-catch blocks. 1. Create a custom exception class derived from the exception class and override the what() method; 2. Use the throw keyword to throw an exception; 3. Use the try-catch block to catch exceptions and specify the exception types that can be handled.
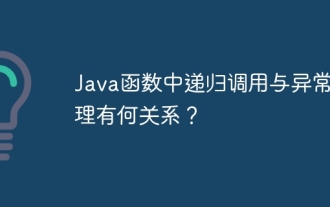
Exception handling in recursive calls: Limiting recursion depth: Preventing stack overflow. Use exception handling: Use try-catch statements to handle exceptions. Tail recursion optimization: avoid stack overflow.

In multithreaded C++, exception handling follows the following principles: timeliness, thread safety, and clarity. In practice, you can ensure thread safety of exception handling code by using mutex or atomic variables. Additionally, consider reentrancy, performance, and testing of your exception handling code to ensure it runs safely and efficiently in a multi-threaded environment.
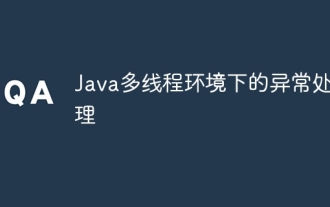
Key points of exception handling in a multi-threaded environment: Catching exceptions: Each thread uses a try-catch block to catch exceptions. Handle exceptions: print error information or perform error handling logic in the catch block. Terminate the thread: When recovery is impossible, call Thread.stop() to terminate the thread. UncaughtExceptionHandler: To handle uncaught exceptions, you need to implement this interface and assign it to the thread. Practical case: exception handling in the thread pool, using UncaughtExceptionHandler to handle uncaught exceptions.
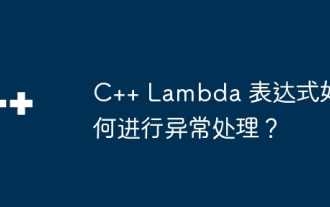
Exception handling in C++ Lambda expressions does not have its own scope, and exceptions are not caught by default. To catch exceptions, you can use Lambda expression catching syntax, which allows a Lambda expression to capture a variable within its definition scope, allowing exception handling in a try-catch block.
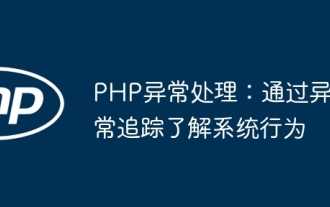
PHP exception handling: Understanding system behavior through exception tracking Exceptions are the mechanism used by PHP to handle errors, and exceptions are handled by exception handlers. The exception class Exception represents general exceptions, while the Throwable class represents all exceptions. Use the throw keyword to throw exceptions and use try...catch statements to define exception handlers. In practical cases, exception handling is used to capture and handle DivisionByZeroError that may be thrown by the calculate() function to ensure that the application can fail gracefully when an error occurs.
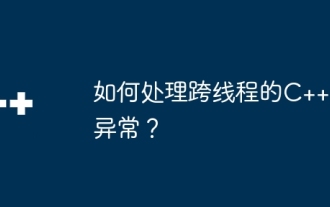
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
