What are the characteristics of functions in Go language?
Go language is an open source programming language. It is known as a simple, efficient, and practical language. Many people consider it to be one of the representatives of modern programming languages. In the Go language, function is an important concept and its role in the program is indispensable. Let’s talk about the characteristics of functions in the Go language.
- Functions are first-class objects
In the Go language, functions are first-class objects, which means that functions can be passed as parameters to other functions, or Returned outside the function as a return value. This feature allows the Go language to use functional programming techniques such as higher-order functions, making the code more concise and flexible.
For example, the following code defines a function that accepts a function as a parameter and calls the function on each element:
func forEach(data []int, f func(int)) { for _, value := range data { f(value) } }
This function can be passed to other functions, such as :
func printData(data []int) { forEach(data, func(i int) { fmt.Println(i) }) }
In this example, we use the forEach
function to print out each element in data
, where we use an anonymous function as a parameter.
- Function can return multiple values
In Go language, function can return multiple values. This is an advantage that many other languages do not have. This feature can reduce the amount of code and improve program performance.
For example, the following code:
func calculate(a float64, b float64) (float64, float64) { return a + b, a - b }
This function accepts two floating point numbers a
and b
as parameters and returns their sum and difference. And the returned result can be obtained using multiple assignment:
sum, difference := calculate(3, 2)
Heresum
and difference
are 5
and 1## respectively #.
- Functions can be variadic functions
func print(args ...interface{}) { for _, v := range args { fmt.Print(v, " ") } fmt.Println() }
print("hello", "world", 123, true)
print function to print out the four parameters.
- Functions support closures
func incr() func() int { i := 0 return func() int { i++ return i } } func main() { inc := incr() fmt.Println(inc()) // 输出 1 fmt.Println(inc()) // 输出 2 fmt.Println(inc()) // 输出 3 }
incr function returns an internally defined closure function. Each time this closure function is called, it increments the counter and returns the new value.
- Function as method
Person and defines a
SayHello method on it:
type Person struct { name string } func (p Person) SayHello() { fmt.Printf("Hello, my name is %s ", p.name) } func main() { p := Person{"Tom"} p.SayHello() }
SayHello method and create an object
p of type
Person in the
main function. Then call this method to print
Hello, my name is Tom.
The above is the detailed content of What are the characteristics of functions in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


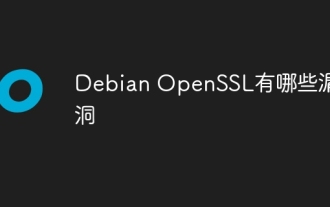
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
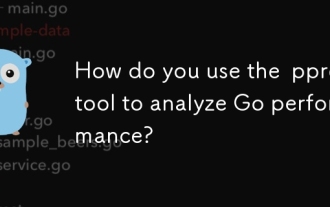
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
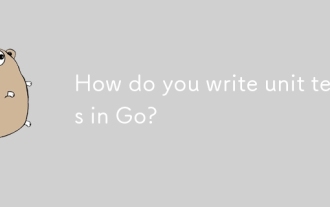
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
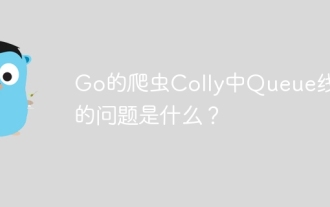
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
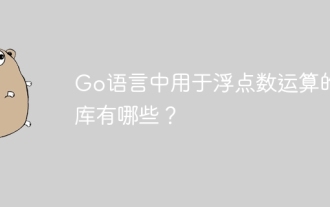
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
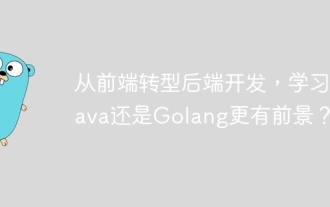
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
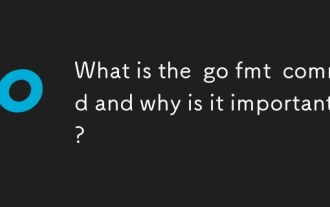
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
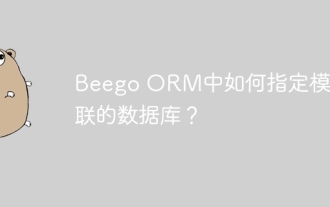
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
