ORM framework in Python Django ORM practice
With the development of the Internet, development software has become more and more popular. In order to improve development efficiency and code management, many languages provide ORM frameworks, and Python is no exception. Django is a Python web framework that provides a powerful ORM framework - Django ORM. This article will introduce how to use Django ORM to manage databases.
- Installing Django
Before using Django ORM, you need to install Django. You can use pip to install it, the command is as follows:
pip install django
- New Django project
After installing Django, you can use it to create a new project, the command is as follows:
django-admin startproject project_name
This will create a Django project named project_name.
- Create database model
In Django ORM, the database model can be defined as a Python class. In the project's app directory, create a file named models.py and define the model in it. For example, to create a model named Book with title, author, pub_date and price attributes, it can be defined as follows:
from django.db import models class Book(models.Model): title = models.CharField(max_length=100) author = models.CharField(max_length=200) pub_date = models.DateField() price = models.DecimalField(max_digits=5, decimal_places=2)
Here, Book inherits the Model class in Django ORM and defines four Attributes. CharField represents character type, DateField represents date type, and DecimalField represents decimal type. When defining each property, you can use parameters to control their type, length, precision, etc.
In addition, you can also define methods and class methods for models. For example, define a class method named get_books in the Book model to obtain all books from the database, as follows:
class Book(models.Model): # 类属性 title = models.CharField(max_length=100) author = models.CharField(max_length=200) pub_date = models.DateField() price = models.DecimalField(max_digits=5, decimal_places=2) # 类方法 @classmethod def get_books(cls): return cls.objects.all()
Here, use the classmethod modifier to modify the get_books method, and Defined as a class method. This method uses the objects attribute in Django ORM to get all Book objects.
- Run database migration
After defining the model, you need to create tables in the database. This process can be accomplished using the makemigrations and migrate commands provided by Django ORM. Using the makemigrations command will generate a migration file containing changes to the model. Using the migrate command will execute the migration file and apply the changes to the database. The command is as follows:
python manage.py makemigrations python manage.py migrate
- Use Django ORM to manage the database
After defining the database model and completing the database migration, you can use Django ORM to manage the database. For example, in views.py you can write the following code:
from django.shortcuts import render from .models import Book def book_list(request): books = Book.get_books() return render(request, 'book_list.html', {'books': books})
Here, import the Book model from models.py and use the get_books method to get all the books. They are then passed as parameters into the render function, which will use the template file book_list.html to render the page.
In book_list.html, you can use the following code to display a list of books:
{% for book in books %} <div class="book"> <h2>{{ book.title }}</h2> <p>{{ book.author }} - {{ book.pub_date|date:"Y年m月d日" }}</p> <p>价格:{{ book.price }}</p> </div> {% endfor %}
Here, use a for loop to traverse all books and output their attributes. It should be noted that when outputting the pub_date attribute, a date filter is used to format the date into the form of year, month and day.
- Summary
Django ORM is a powerful ORM framework that can help developers manage databases easily. This article introduces the process of using Django ORM to create models, run database migrations, and manage databases. I hope this article can help beginners better understand the application of Django ORM.
The above is the detailed content of ORM framework in Python Django ORM practice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
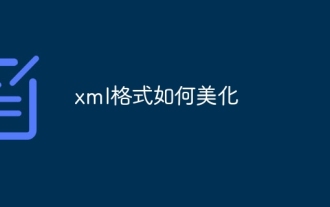
XML beautification is essentially improving its readability, including reasonable indentation, line breaks and tag organization. The principle is to traverse the XML tree, add indentation according to the level, and handle empty tags and tags containing text. Python's xml.etree.ElementTree library provides a convenient pretty_xml() function that can implement the above beautification process.
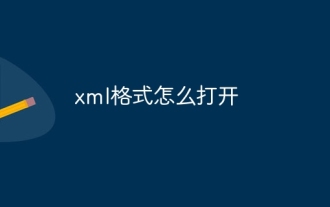
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
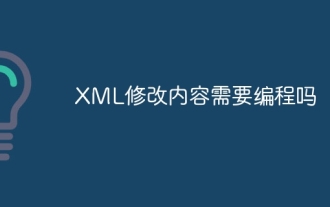
Modifying XML content requires programming, because it requires accurate finding of the target nodes to add, delete, modify and check. The programming language has corresponding libraries to process XML and provides APIs to perform safe, efficient and controllable operations like operating databases.
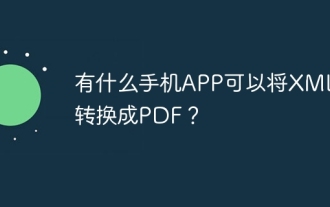
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
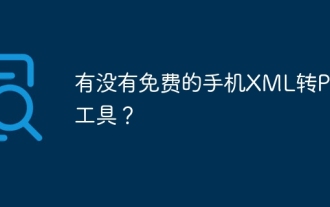
There is no simple and direct free XML to PDF tool on mobile. The required data visualization process involves complex data understanding and rendering, and most of the so-called "free" tools on the market have poor experience. It is recommended to use computer-side tools or use cloud services, or develop apps yourself to obtain more reliable conversion effects.
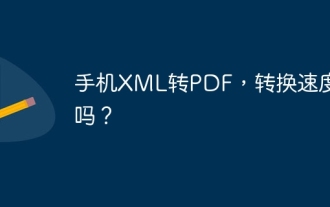
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
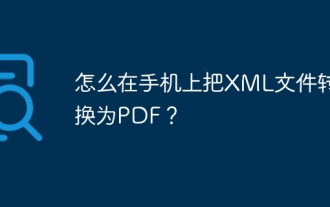
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
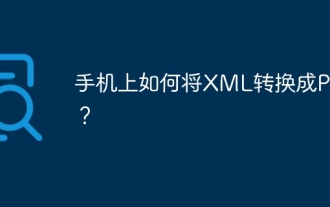
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
