Neural network algorithm examples in Python
Neural network algorithm example in Python
Neural network is an artificial intelligence model that simulates the human nervous system. It can automatically identify patterns and perform tasks such as classification, regression, and clustering by learning data samples. . As a programming language that is easy to learn and has a powerful scientific computing library, Python excels in developing neural network algorithms. This article will introduce examples of neural network algorithms in Python.
- Install related libraries
Commonly used neural network libraries in Python include Keras, Tensorflow, PyTorch, etc. The Keras library is based on Tensorflow, which can simplify the process of building neural networks. , so this article will choose the Keras library as the development tool for neural network algorithms. Before using the Keras library, you need to install the Tensorflow library as a backend. Execute the following command on the command line to install the dependent libraries:
pip install tensorflow pip install keras
- Dataset Preprocessing
Before training the neural network, the data needs to be preprocessed . Common data preprocessing includes data normalization, data missing value processing, data feature extraction, etc. In this article, we will use the iris data set for example demonstration. The data set contains 150 records, each record has four features: sepal length, sepal width, petal length, petal width, and the corresponding classification label: Iris Setosa, Iris Versicolour, Iris Virginica. In this dataset, every record is of numeric type, so we just need to normalize the data.
from sklearn.datasets import load_iris from sklearn.preprocessing import MinMaxScaler import numpy as np # 导入数据集 data = load_iris().data labels = load_iris().target # 归一化数据 scaler = MinMaxScaler() data = scaler.fit_transform(data) # 将标签转化为 one-hot 向量 one_hot_labels = np.zeros((len(labels), 3)) for i in range(len(labels)): one_hot_labels[i, labels[i]] = 1
- Building a neural network model
In Keras, you can use the Sequential model to build a neural network model. In this model, we can add multiple layers, each layer has a specific role, such as fully connected layer, activation function layer, Dropout layer, etc. In this example, we use two fully connected layers and one output layer to build a neural network model, in which the number of neurons in the hidden layer is 4.
from keras.models import Sequential from keras.layers import Dense, Dropout from keras.optimizers import Adam # 构建神经网络模型 model = Sequential() model.add(Dense(4, activation='relu')) model.add(Dense(4, activation='relu')) model.add(Dense(3, activation='softmax')) # 配置优化器和损失函数 optimizer = Adam(lr=0.001) model.compile(optimizer=optimizer, loss='categorical_crossentropy', metrics=['accuracy'])
- Training model
Before training the model, we need to divide the data set into a training set and a test set in order to evaluate the accuracy of the model. In this example, we use 80% of the data as the training set and 20% of the data as the test set. When training, we need to specify parameters such as batch size and number of iterations to control the training speed and model accuracy.
from sklearn.model_selection import train_test_split # 将数据集分为训练集和测试集 train_data, test_data, train_labels, test_labels = train_test_split(data, one_hot_labels, test_size=0.2) # 训练神经网络 model.fit(train_data, train_labels, batch_size=5, epochs=100) # 评估模型 accuracy = model.evaluate(test_data, test_labels)[1] print('准确率:%.2f' % accuracy)
- The complete code of the example
The complete code of this example is as follows:
from sklearn.datasets import load_iris from sklearn.preprocessing import MinMaxScaler from sklearn.model_selection import train_test_split import numpy as np from keras.models import Sequential from keras.layers import Dense, Dropout from keras.optimizers import Adam # 导入数据集 data = load_iris().data labels = load_iris().target # 归一化数据 scaler = MinMaxScaler() data = scaler.fit_transform(data) # 将标签转化为 one-hot 向量 one_hot_labels = np.zeros((len(labels), 3)) for i in range(len(labels)): one_hot_labels[i, labels[i]] = 1 # 将数据集分为训练集和测试集 train_data, test_data, train_labels, test_labels = train_test_split(data, one_hot_labels, test_size=0.2) # 构建神经网络模型 model = Sequential() model.add(Dense(4, activation='relu')) model.add(Dense(4, activation='relu')) model.add(Dense(3, activation='softmax')) # 配置优化器和损失函数 optimizer = Adam(lr=0.001) model.compile(optimizer=optimizer, loss='categorical_crossentropy', metrics=['accuracy']) # 训练神经网络 model.fit(train_data, train_labels, batch_size=5, epochs=100) # 评估模型 accuracy = model.evaluate(test_data, test_labels)[1] print('准确率:%.2f' % accuracy)
- Conclusion
This article introduces examples of neural network algorithms in Python, and uses the iris data set as an example for demonstration. During the implementation process, we used the Keras library and Tensorflow library as neural network development tools, and used the MinMaxScaler library to normalize the data. The results of this example show that our neural network model achieved an accuracy of 97.22%, proving the effectiveness and applicability of the neural network.
The above is the detailed content of Neural network algorithm examples in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


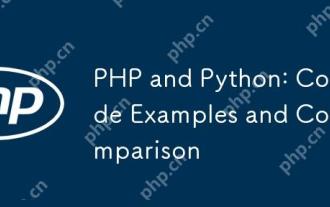
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
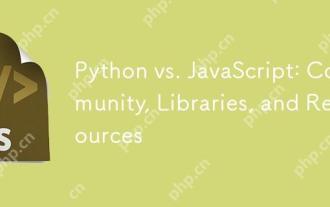
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
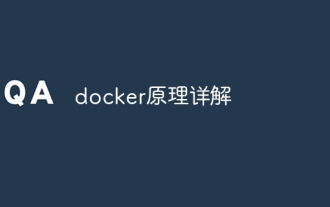
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
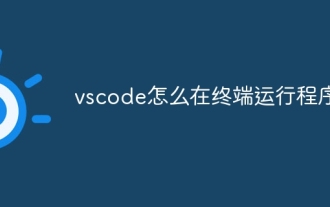
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
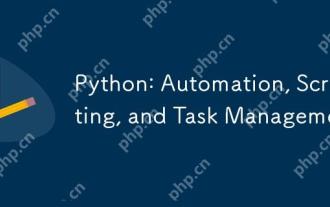
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
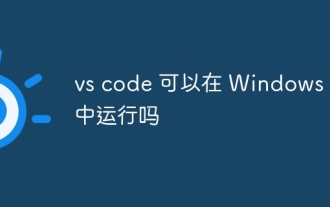
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
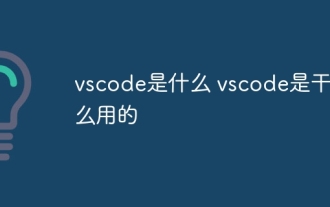
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
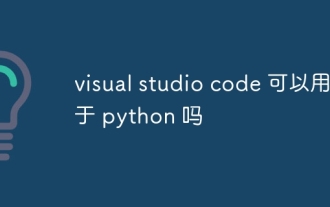
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
