How to use Go language for network programming?
In today's highly connected world, network programming is very important. As a fast, powerful and simple programming language, Go language is becoming more and more popular in the field of network programming. The following will introduce how to use Go language for network programming.
- TCP Network Programming
TCP is a connection-based protocol that provides reliable data transmission and guarantees the sequence of data. In Go language, network programming can be implemented using the net library. Below is a simple TCP client and server example.
Client code:
package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("tcp", "localhost:8000") if err != nil { fmt.Println(err) return } defer conn.Close() fmt.Fprintln(conn, "Hello, server!") }
Server code:
package main import ( "fmt" "net" ) func main() { ln, err := net.Listen("tcp", ":8000") if err != nil { fmt.Println(err) return } defer ln.Close() fmt.Println("Listening on :8000...") for { conn, err := ln.Accept() if err != nil { fmt.Println(err) continue } handleConnection(conn) } } func handleConnection(conn net.Conn) { defer conn.Close() buf := make([]byte, 1024) n, err := conn.Read(buf) if err != nil { fmt.Println(err) return } fmt.Println("Received:", string(buf[:n])) }
- UDP network programming
Different from TCP, UDP is a A connectionless protocol that does not guarantee the reliability and sequence of data transmission. However, its advantage lies in its high speed and ability to transfer data quickly. In the Go language, UDP is implemented similarly to TCP, also using the net library.
The following is a basic UDP client and server example.
Client code:
package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("udp", "127.0.0.1:8000") if err != nil { fmt.Println(err) return } defer conn.Close() fmt.Fprintln(conn, "Hello, server!") }
Server code:
package main import ( "fmt" "net" ) func main() { addr, err := net.ResolveUDPAddr("udp", ":8000") if err != nil { fmt.Println(err) return } conn, err := net.ListenUDP("udp", addr) if err != nil { fmt.Println(err) return } defer conn.Close() fmt.Println("Listening on ", addr) buf := make([]byte, 1024) for { n, _, err := conn.ReadFromUDP(buf) if err != nil { fmt.Println(err) continue } fmt.Println("Received:", string(buf[:n])) } }
- HTTP network programming
HTTP is based on the request-response model protocol, widely used in network communications. In Go language, you can use the built-in net/http library for HTTP network programming.
The following is a basic HTTP server example, which listens to the local port 8080 and returns a simple "Hello, World!" string:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, World!") }) http.ListenAndServe(":8080", nil) }
The above is common in the Go language Network programming implementation. Of course, this is just an entry-level implementation of network programming. Based on this, you can further explore the powerful network programming capabilities of the Go language.
The above is the detailed content of How to use Go language for network programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


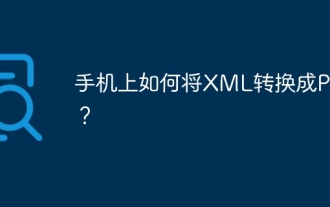
It is not easy to convert XML to PDF directly on your phone, but it can be achieved with the help of cloud services. It is recommended to use a lightweight mobile app to upload XML files and receive generated PDFs, and convert them with cloud APIs. Cloud APIs use serverless computing services, and choosing the right platform is crucial. Complexity, error handling, security, and optimization strategies need to be considered when handling XML parsing and PDF generation. The entire process requires the front-end app and the back-end API to work together, and it requires some understanding of a variety of technologies.
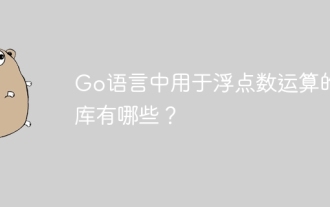
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
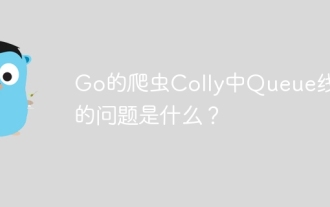
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
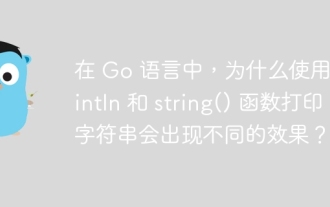
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
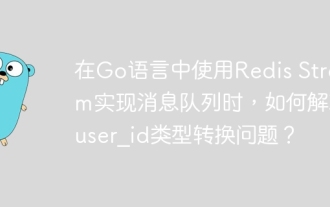
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
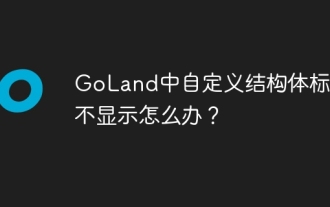
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
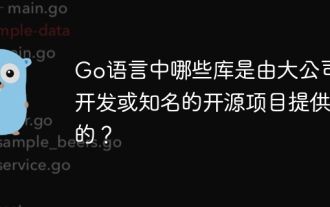
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
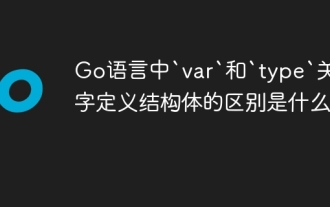
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
