How to use expressions to calculate dynamic styles in Vue
Vue is a lightweight JavaScript framework that provides an easy way to manage applications and render dynamic content. Style binding in Vue allows you to use expressions to calculate dynamic styles, giving your application more flexibility and scalability.
In this article, we will introduce how to use expressions to calculate dynamic styles in Vue.
1. Binding in Vue
There are many types of binding in Vue, including property binding, class binding, style binding, etc. Among them, style binding provides a way to use expressions to calculate styles.
To use style binding, we can use the "v-bind:style" directive in the Vue component to specify the style attribute value. The value of this directive can be an object whose properties are the style names and whose values are the style's computed expressions.
For example, the following component will dynamically calculate the "color" style based on the boolean "isRed":
<template> <div :style="{ color: isRed ? 'red' : 'black' }"> 这是一段动态颜色文字 </div> </template> <script> export default { data() { return { isRed: true } } } </script>
In this example, the ":style" binding will dynamically set the "div" element text color. The style object contains a property called "color" whose value is a ternary expression that evaluates to "red" or "black" based on the Boolean value "isRed".
2. Style binding expressions
In Vue, we can use JavaScript expressions to calculate styles. These expressions can be simple arithmetic operations, logical operations, or even function calls.
For example, we can calculate the style by adding a numeric property to a string:
<template> <div :style="{ fontSize: size + 'px' }"> 根据变量计算字体大小 </div> </template> <script> export default { data() { return { size: 16 } } } </script>
In this example, the style object contains a property named "fontSize" whose The value is an expression that adds the "size" variable to the "px" string to calculate the font size in one pixel.
In addition, we can also use ternary expressions to calculate styles. For example, in the following example, the style is dynamically calculated based on two variables:
<template> <div :style="{ backgroundColor: isActive ? activeColor : inactiveColor }"> 根据变量动态计算背景颜色 </div> </template> <script> export default { data() { return { isActive: true, activeColor: 'red', inactiveColor: 'blue' } } } </script>
In this example, the ":style" binding will dynamically calculate the background color based on the boolean value "isActive". The style object contains a property called "backgroundColor" whose value is a ternary expression that evaluates to "activeColor" or "inactiveColor" based on the Boolean value "isActive".
3. Dynamically binding styles
We can also dynamically bind styles in Vue components. For example, in the following example, we will dynamically set a style based on a variable:
<template> <div :class="{ active: isActive }" :style="{ backgroundColor: bgColor }"> 这是一个动态类和样式的元素 </div> </template> <script> export default { data() { return { isActive: true, bgColor: 'red' } } } </script>
In this example, we use style and class binding to dynamically set the background color and class of the corresponding element. Style binding uses the ":style" directive to use the "bgColor" variable as the value of the "backgroundColor" style. Class binding uses the ":class" directive to take an object containing the "active" class as its value.
Summary
This article introduces how to use expressions to calculate dynamic styles in Vue. We learned how to dynamically set styles using the "v-bind:style" directive and were shown some examples of dynamic styling. By using dynamic styles, we can make our Vue applications more flexible and extensible to better suit our needs.
The above is the detailed content of How to use expressions to calculate dynamic styles in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


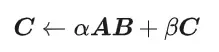
General Matrix Multiplication (GEMM) is a vital part of many applications and algorithms, and is also one of the important indicators for evaluating computer hardware performance. In-depth research and optimization of the implementation of GEMM can help us better understand high-performance computing and the relationship between software and hardware systems. In computer science, effective optimization of GEMM can increase computing speed and save resources, which is crucial to improving the overall performance of a computer system. An in-depth understanding of the working principle and optimization method of GEMM will help us better utilize the potential of modern computing hardware and provide more efficient solutions for various complex computing tasks. By optimizing the performance of GEMM
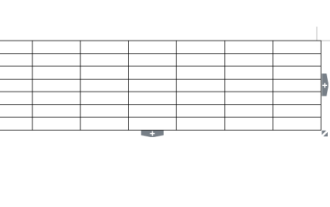
WORD is a powerful word processor. We can use word to edit various texts. In Excel tables, we have mastered the calculation methods of addition, subtraction and multipliers. So if we need to calculate the addition of numerical values in Word tables, How to subtract the multiplier? Can I only use a calculator to calculate it? The answer is of course no, WORD can also do it. Today I will teach you how to use formulas to calculate basic operations such as addition, subtraction, multiplication and division in tables in Word documents. Let's learn together. So, today let me demonstrate in detail how to calculate addition, subtraction, multiplication and division in a WORD document? Step 1: Open a WORD, click [Table] under [Insert] on the toolbar, and insert a table in the drop-down menu.

How to use Python's count() function to calculate the number of an element in a list requires specific code examples. As a powerful and easy-to-learn programming language, Python provides many built-in functions to handle different data structures. One of them is the count() function, which can be used to count the number of elements in a list. In this article, we will explain how to use the count() function in detail and provide specific code examples. The count() function is a built-in function of Python, used to calculate a certain

Introduction The Java program for calculating the area of a triangle using determinants is a concise and efficient program that can calculate the area of a triangle given the coordinates of three vertices. This program is useful for anyone learning or working with geometry, as it demonstrates how to use basic arithmetic and algebraic calculations in Java, as well as how to use the Scanner class to read user input. The program prompts the user for the coordinates of three points of the triangle, which are then read in and used to calculate the determinant of the coordinate matrix. Use the absolute value of the determinant to ensure the area is always positive, then use a formula to calculate the area of the triangle and display it to the user. The program can be easily modified to accept input in different formats or to perform additional calculations, making it a versatile tool for geometric calculations. ranks of determinants
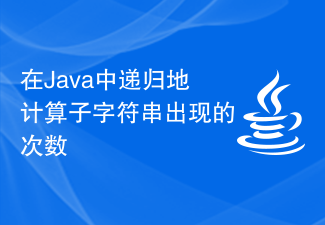
Given two strings str_1 and str_2. The goal is to count the number of occurrences of substring str2 in string str1 using a recursive procedure. A recursive function is a function that calls itself within its definition. If str1 is "Iknowthatyouknowthatiknow" and str2 is "know" the number of occurrences is -3. Let us understand through examples. For example, input str1="TPisTPareTPamTP", str2="TP"; output Countofoccurrencesofasubstringrecursi
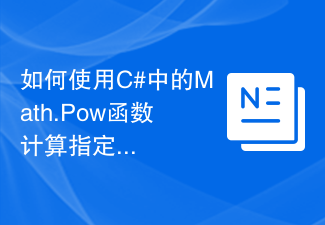
In C#, there is a Math class library, which contains many mathematical functions. These include the function Math.Pow, which calculates powers, which can help us calculate the power of a specified number. The usage of the Math.Pow function is very simple, you only need to specify the base and exponent. The syntax is as follows: Math.Pow(base,exponent); where base represents the base and exponent represents the exponent. This function returns a double type result, that is, the power calculation result. Let's
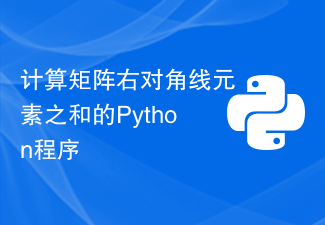
A popular general-purpose programming language is Python. It is used in a variety of industries, including desktop applications, web development, and machine learning. Fortunately, Python has a simple and easy-to-understand syntax that is suitable for beginners. In this article, we will use Python to calculate the sum of the right diagonal of a matrix. What is a matrix? In mathematics, we use a rectangular array or matrix to describe a mathematical object or its properties. It is a rectangular array or table containing numbers, symbols, or expressions arranged in rows and columns. . For example -234512367574 Therefore, this is a matrix with 3 rows and 4 columns, expressed as a 3*4 matrix. Now, there are two diagonals in the matrix, the primary diagonal and the secondary diagonal
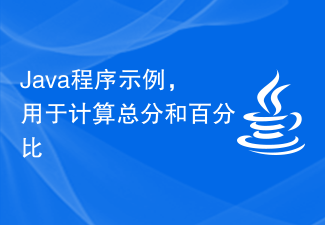
We will demonstrate how to calculate total scores and percentages using a Java program. Total score refers to the sum of all available scores, while the term percentage refers to the calculated score divided by the total score and multiplied by the resulting number 100. percentage_of_marks=(obtained_marks/total_marks)×100 Example 1 This is a Java program that demonstrates how to calculate total scores and percentages. //JavaProgramtodemonstratehowisTotalmarksandPercentagescalculatedimportjava.io.*;publicclassTotalMarks_
