Automated testing techniques in Python
Python is an open source programming language with efficient programming and rich functions, and is widely used in the field of automated testing. In the process of implementing automated testing, Python provides many techniques and tools that can significantly improve testing efficiency and quality.
This article will introduce some Python automated testing techniques, including using Python's unittest library to write test cases, using automation tools such as Selenium to test web applications, using simulation tools such as Mock for unit testing, and using Pytest Wait for the testing framework to perform integration testing.
1. Use Python’s unittest library to write test cases
Unittest is a testing framework that comes with Python. It organizes tests by defining test cases and test suites, and provides various test reports. and result statistics tools. The following is a sample code for writing test cases using the unittest library:
import unittest class MyTestCase(unittest.TestCase): def test_addition(self): a = 2 b = 3 self.assertEqual(a + b, 5) def test_subtraction(self): a = 5 b = 3 self.assertEqual(a - b, 2) if __name__ == '__main__': unittest.main()
In the above code, the MyTestCase class inherits from the unittest.TestCase class, and the test case consists of methods starting with test_. In each test case, verify whether the logic is correct by asserting using the assertEqual() method.
2. Use automated tools such as Selenium to test web applications
Selenium is a popular automated testing tool that can be used to test various functions and behaviors of web applications. By using the Selenium WebDriver library and Python, automated test scripts can be written to simulate human actions such as clicking, entering text, selecting options, etc.
The following is a sample code for web application testing using Selenium WebDriver library:
from selenium import webdriver class MyTestCase(unittest.TestCase): def setUp(self): self.driver = webdriver.Chrome() def tearDown(self): self.driver.quit() def test_login(self): self.driver.get("http://example.com") self.driver.find_element_by_id("username").send_keys("user") self.driver.find_element_by_id("password").send_keys("password") self.driver.find_element_by_id("login-button").click() assert "Welcome, user!" in self.driver.page_source
In the above sample code, the setUp() method and tearDown() method can be used in each test case Executed at start and end. In the test_login() test case, use the Selenium WebDriver library to automate the login process and check whether the login is successful.
3. Use simulation tools such as Mock for unit testing
Mock is a simulation library in Python that can create virtual objects to simulate the behavior of actual objects. Mock libraries can be used in unit tests to mock and isolate code dependencies during testing.
The following is a sample code for unit testing using the Mock library:
from unittest.mock import MagicMock def test_addition(): mock_object = MagicMock() mock_object.add = MagicMock(return_value=5) result = mock_object.add(2, 3) assert result == 5
In the above code, use the MagicMock() method to create a virtual object. The behavior of an actual object is simulated by calling the object's add() method and setting the return value using MagicMock(). In the assert statement, it is tested whether the result returned by the function is as expected.
4. Use testing frameworks such as Pytest for integration testing
Pytest is a popular Python testing framework that provides a wealth of functions and plug-ins to support various types of automated testing. Using Pytest, you can write Python test cases and perform integration testing on multiple modules and components.
The following is a sample code for integration testing using the Pytest framework:
def test_addition(): a = 2 b = 3 result = add(a, b) assert result == 5 def test_subtraction(): a = 5 b = 3 result = subtract(a, b) assert result == 2
In the above code, two test cases are written using the Pytest framework to test the addition and subtraction functions respectively. The assert statement is used to check whether the results of the test are as expected.
Summary
Python provides many techniques and tools that can be used to automate testing and improve testing efficiency and quality. From writing test cases to using automation tools, simulation tools, and testing frameworks, this article introduces some common techniques for Python automated testing. These techniques and tools can help the testing team find and correct errors more quickly, ensuring the high quality and stability of the software.
The above is the detailed content of Automated testing techniques in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


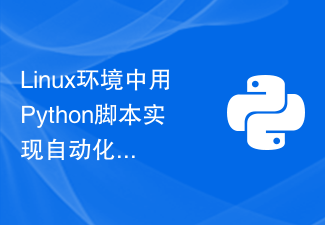
How to use Python scripts to implement automated testing in the Linux environment. With the rapid development of software development, automated testing plays a vital role in ensuring software quality and improving development efficiency. As a simple and easy-to-use programming language, Python has strong portability and development efficiency, and is widely used in automated testing. This article will introduce how to use Python to write automated test scripts in a Linux environment and provide specific code examples. Environment Preparation for Automation in Linux Environment
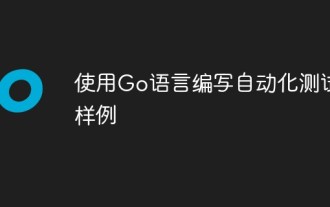
With the rapid development of software development, automated testing plays an increasingly important role in the development process. Compared with manual testing, automated testing can improve the efficiency and accuracy of testing and reduce delivery time and costs. Therefore, mastering automated testing becomes very necessary. Go language is a modern and efficient programming language. Due to its unique concurrency model, memory management and garbage collection mechanism, it has been widely used in web applications, network programming, large-scale concurrency, distributed systems and other fields. In terms of automated testing,
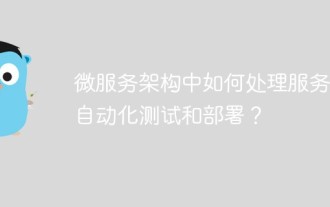
With the rapid development of Internet technology, microservice architecture is becoming more and more widely used. Using a microservice architecture can effectively avoid the complexity and code coupling of a single application, and improve the scalability and maintainability of the application. However, unlike monolithic applications, in a microservice architecture, there are a huge number of services, and each service requires automated testing and deployment to ensure the quality and reliability of the service. This article will discuss how to handle automated testing and deployment of services in a microservices architecture. 1. Automated testing in microservice architecture Automated testing is the guarantee

As Internet companies continue to grow, software development becomes more and more complex, and testing becomes more and more important. In order to ensure the correctness and stability of the program, various types of tests must be performed. Among them, automated testing is a very important way. It can improve the efficiency of testing work, reduce error rates, and allow repeated execution of test cases to detect problems early. However, in the actual operation process, we will also encounter various problems, such as Issues such as selection of testing tools, writing of test cases, and setting up of test environment. go-zero
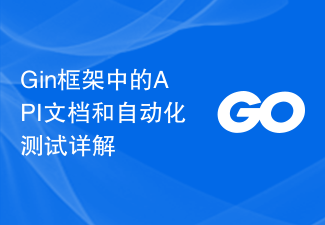
Gin is a web framework written in Golang. It has the advantages of efficiency, lightweight, flexibility, relatively high performance, and easy to use. In Gin framework development, API documentation and automated testing are very important. This article will take an in-depth look at API documentation and automated testing in the Gin framework. 1. API documentation API documentation is used to record the detailed information of all API interfaces to facilitate the use and understanding of other developers. The Gin framework provides a variety of API documentation tools, including Swagger, GoSwa
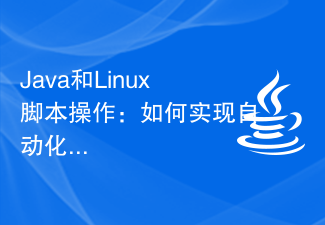
Java and Linux Script Operations: Methods and Examples for Implementing Automated Testing Introduction: In the software development process, automated testing can greatly improve testing efficiency and quality. By using Java language and Linux scripts, we can write powerful automated test scripts to automatically execute test cases, generate test reports and other functions. This article will introduce how to use Java and Linux scripts to implement automated testing and provide some specific code examples. 1. Java automated testing: Java is a
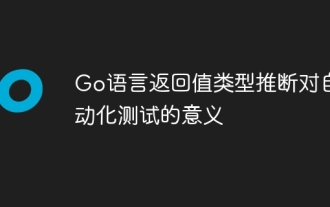
Go language return type inference simplifies automated testing: it allows the compiler to infer the return type based on the function implementation, eliminating the need for explicit declarations. Improve the simplicity and readability of test functions and simplify function output verification. Practical cases show how to use type inference to write automated tests to verify that function output meets expectations.
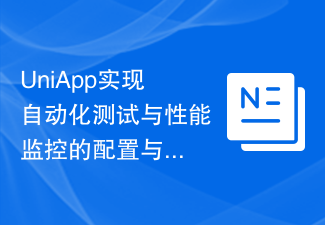
UniApp is a cross-platform application development framework that can quickly develop applications that adapt to multiple platforms at the same time. During the development process, we often need to conduct automated testing and performance monitoring to ensure the quality and performance of the application. This article will introduce how to configure and use automated testing and performance monitoring tools in UniApp. 1. Automated test configuration and usage guide. Download and install the necessary tools. UniApp’s automated testing relies on Node.js and WebdriverIO. First, we need to
