How to use REST API in PHP programming?
In today's Internet world, the interconnection and interaction of applications have become routine operations. REST API is a communication protocol, a simple Web service interface architecture that does not require knowing the implementation details of the other party, and provides an abstraction layer of resource information to the client. When writing PHP applications, REST API can help us interact better with other applications. In this article, we will discuss in depth how to use REST API in PHP programming.
What is REST API?
REST API is a web development architecture. In REST (Representational State Transfer) architecture, server-side data is saved in different forms. Common storage formats include: XML, JSON and HTML. REST is based on the HTTP protocol and uses GET, POST, DELETE and PUT methods to perform requests.
REST API is a web service that performs HTTP requests. APIs can be public or private. In REST API, resources can be accessed through URI (Uniform Resource Identifier). There are many advantages to using REST API, such as:
- It uses the working principle of HTTP protocol to transmit data more efficiently;
- REST API can be used in different ways for different clients. Present different data in a way to meet the needs of the client;
- By using the REST API, applications can be more easily platformed or ported.
How to use REST API in PHP programming
Here are the steps to use REST API in PHP programming:
Step 1: Determine API
First, you need to identify the API you are using. If you want to interact with different applications then you need to use their API. Before you start using the API, you need to read the API documentation to understand how to call it.
Step 2: Use the CURL library to perform the request
The CURL library is a simple and easy-to-use PHP library that can be used to perform HTTP requests. This library is included in many PHP versions. For PHP versions without this extension, you need to install it manually. The CURL library can easily establish HTTP requests, including GET requests, POST requests, PUT requests, DELETE requests, etc.
The basic code to send an HTTP request using the CURL library is as follows:
$url = 'http://example.com/data.json'; $options = array( CURLOPT_RETURNTRANSFER => true, // Return web page as a string CURLOPT_HEADER => false, // Don't include headers in the returned string CURLOPT_FOLLOWLOCATION => true, // Follow redirects CURLOPT_ENCODING => "", // Handle all encodings CURLOPT_USERAGENT => "spider", // User-agent header to send CURLOPT_AUTOREFERER => true, // Automatically set the referer where following or redirecting CURLOPT_CONNECTTIMEOUT => 120, // Timeout (in seconds) for the connection phase CURLOPT_TIMEOUT => 120, // Timeout (in seconds) for the entire request ); $ch = curl_init($url); curl_setopt_array($ch, $options); $content = curl_exec($ch); curl_close($ch);
Step 3: Decode the response using the JSON library
When you use a REST API, the API may end up in JSON format return data. In PHP programming, you need to use a JSON library to decode the response. The json_decode() function in the JSON library can conveniently decode JSON data into an array. Below is a code sample to decode a JSON response using the json_decode() function:
$content = '{"name": "John", "age": 25}'; $data = json_decode($content, true); echo 'Name: ' . $data['name'] . ', Age: ' . $data['age'];
Step 4: Process the response
Once you have the response data, you need to process this data according to your requirements. Typically, you need to store data in a database or display it on your web page. Here is a code sample that displays the response on the page:
$content = '{ "customer": { "name": "John Smith", "email": "john@example.com", "phone": "123-456-7890" }, "items": [ { "name": "Widget", "price": 9.99 }, { "name": "Gadget", "price": 19.99 } ] }'; $data = json_decode($content, true); echo 'Customer Name: ' . $data['customer']['name'] . '<br>'; echo 'Customer Email: ' . $data['customer']['email'] . '<br>'; echo 'Customer Phone: ' . $data['customer']['phone'] . '<br>'; echo '<table>'; foreach ($data['items'] as $item) { echo '<tr><td>' . $item['name'] . '</td><td>' . $item['price'] . '</td></tr>'; } echo '</table>';
Summary
When writing PHP applications, interaction with other applications is very important. REST API is a communication protocol that helps us interact better with other applications. Using the CURL library, JSON library, and HTTP requests, we can easily interact with the API. By using REST API, we can transfer data efficiently, display different data for different clients, and port or platform the application more easily.
The above is the detailed content of How to use REST API in PHP programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


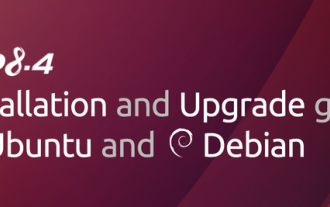
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
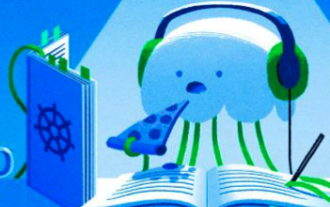
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
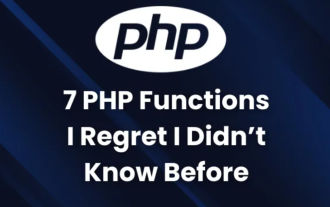
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
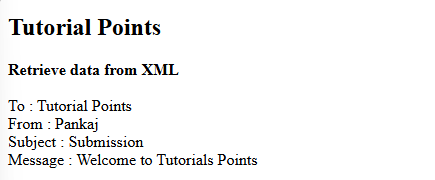
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
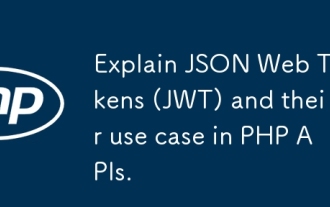
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
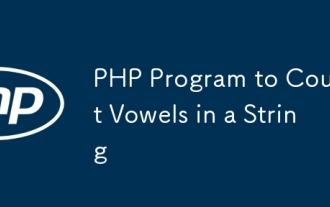
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
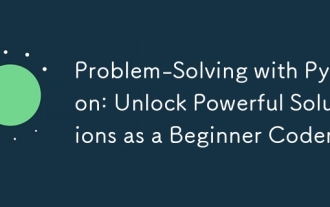
Pythonempowersbeginnersinproblem-solving.Itsuser-friendlysyntax,extensivelibrary,andfeaturessuchasvariables,conditionalstatements,andloopsenableefficientcodedevelopment.Frommanagingdatatocontrollingprogramflowandperformingrepetitivetasks,Pythonprovid
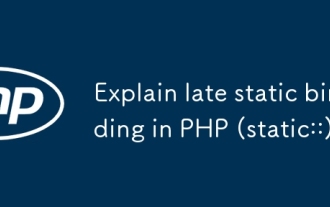
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
