What are the common array operations in PHP programming?
In PHP programming, arrays are a very important form of data structure. They allow us to organize and access data efficiently. Arrays in PHP include indexed arrays, associative arrays, and multidimensional arrays, which can be used in various scenarios, such as processing form data in web development and retrieving data from databases. In this article, we will explore common array operations in PHP programming.
- Creating an array
Creating an array is the most basic operation using PHP. In PHP, there are two ways to create an array. The first is to use the array() function:
$array = array("apple", "banana", "orange");
The second is to use square brackets []:
$array = ["apple", "banana", "orange"];
Of course, both methods can create an identical indexed array.
- Add element
Adding an element to an array is a common operation. An element can be added to the end of the array using the array_push() function:
$array = ["apple", "banana", "orange"]; array_push($array, "mango");
The above code adds the $mango element to the end of the array.
- Accessing array elements
Accessing array elements is one of the common operations in PHP. Based on the subscript, we can easily get the elements in the array. In PHP, the subscript of an array can be a number or a string, as follows:
$array = ["apple", "banana", "orange"]; echo $array[0]; echo $array[1]; echo $array[2];
The above code will output the first, second and third elements in the array.
- Delete elements
Deleting elements in an array is a necessary operation, but sometimes it can produce unexpected results. To delete an element from an array, you can use the unset() function:
$array = ["apple", "banana", "orange"]; unset($array[1]);
The above code will delete the second element in the array - 'banana'. Note that this operation not only deletes the element, but also deletes the subscript in the array.
- Length and number of arrays
Sometimes, we need to know how many elements there are in the array in order to take appropriate action. You can use the count() function to get the length of the array and the number of elements:
$array = ["apple", "banana", "orange"]; echo count($array);
The above code will output the number of elements in the array.
- Traversing an array
Traversing an array is a basic operation for processing arrays in PHP. PHP provides several ways to traverse index arrays and associative arrays:
// 遍历索引数组 $array = ["apple", "banana", "orange"]; foreach ($array as $value) { echo $value; } // 遍历关联数组 $age = ["Peter"=>"35", "Ben"=>"37", "Joe"=>"43"]; foreach($age as $key => $value) { echo "Key=" . $key . ", Value=" . $value; }
The above code will traverse index arrays and associative arrays respectively.
- Array sorting
In PHP, we can sort an array in a specific order. The sort() function is used to sort the array elements in ascending order, and the rsort() function is used to sort the array elements in descending order:
// 升序排列数组 $array = ["apple", "banana", "orange"]; sort($array); // 降序排列数组 $array = ["apple", "banana", "orange"]; rsort($array);
The above code will sort the array elements in ascending order and descending order respectively.
- Check whether an array contains an element
Sometimes we need to check whether an array contains an element. Using the in_array() function, you can check whether a value exists in the array:
$array = ["apple", "banana", "orange"]; if (in_array("apple", $array)) { echo "存在"; } else { echo "不存在"; }
The above code will check whether the "apple" element exists in the array.
Summary
They are the most basic array operations in PHP programming. Understanding the concepts of these array operations is very important and can help us more efficiently handle data in web development and handle other programming tasks. There are many other PHP array operations, only the most basic ones are listed here. After understanding these basic operations, you can now write more efficient PHP code to process data.
The above is the detailed content of What are the common array operations in PHP programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


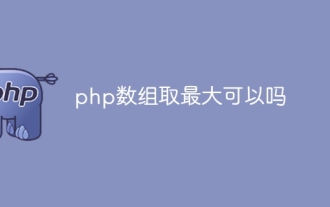
There are three ways to get the maximum value of a PHP array: 1. The "max()" function is used to get the maximum value in the array; 2. The "rsort()" function is used to sort the array in descending order and return the sort. the array after. You can get the maximum value by accessing the element with index 0; 3. Use loop traversal to compare the value of each element to find the maximum value.
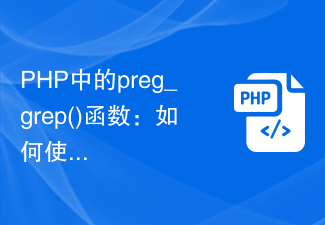
The preg_grep() function in PHP: How to use regular expressions to filter elements in an array Overview: In PHP development, we often need to filter and process arrays. Sometimes, we may want to remove only elements from an array that meet certain conditions. At this time, you can use the preg_grep() function in PHP to achieve this. This function can filter elements in an array by using regular expressions, which is very convenient and practical. This article will introduce the use of preg_grep() function in detail and provide
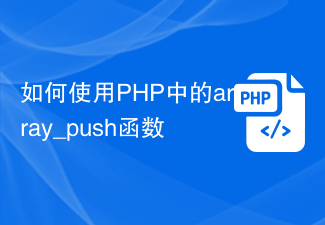
In PHP development, array is a very common data structure. In actual development, we often need to add new elements to the array. For this purpose, PHP provides a very convenient function array_push. The array_push function is used to add one or more elements to the end of an array. The syntax of this function is as follows: array_push(array, value1, value2,...) where array is the array to which elements need to be added, value1, valu
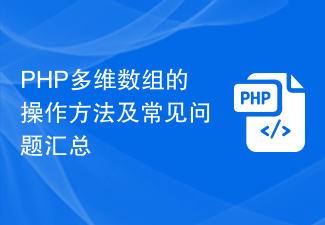
PHP is a programming language widely used in web development. It has a rich set of tools and function libraries, among which multidimensional arrays are one of its important data structures. Multidimensional arrays allow us to organize data into tables or matrices for easy data processing and manipulation. This article will introduce the basic operation methods and common problems of multi-dimensional arrays in PHP, and provide practical examples to illustrate. 1. Basic operation methods 1. Define multi-dimensional arrays We can define a multi-dimensional array through a variety of methods. Here are some common methods: $myArray
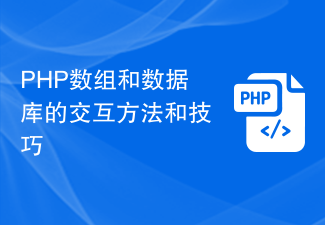
Interaction methods and techniques for PHP arrays and databases Introduction: In PHP development, operating arrays is a basic skill, and interaction with databases is a common requirement. This article will introduce how to use PHP to manipulate arrays and interact with databases, and provide some practical code examples. 1. Basic operations of PHP arrays In PHP, arrays are a very useful data type that can be used to store and operate a group of related data. Here are some common examples of array operations: Create an array: $arr=array
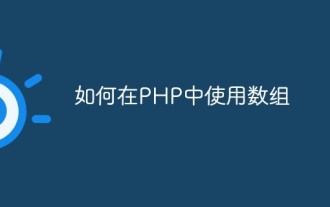
PHP is a powerful programming language that provides a powerful data structure through arrays that can help us manage and process complex data. At the same time, arrays are also one of the most commonly used data types in PHP, which allow us to put multiple data values into a single variable. In this article, we will discuss how to use arrays in PHP. Creating an Array In PHP, we can use the array() function to create an array. For example, the following code creates an array of three strings: $myArra
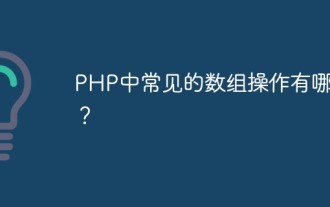
PHP is a widely used server-side programming language and a very important part of Internet application development. In PHP, array is a very common data type that can be used to store and manipulate a group of related data. In this article, we will introduce common array operations in PHP, hoping to be helpful to PHP developers. Creating an array In PHP, creating an array is very simple. You can use the array() function or the [] symbol to create it. For example, the following code will create an array with three elements:
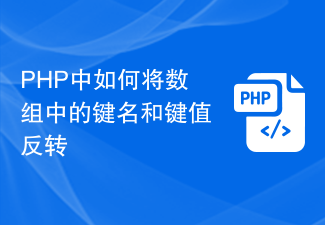
How to reverse the key names and key values in an array in PHP In PHP, we often need to deal with arrays. Sometimes, we need to reverse the key names and key values in the array, that is, use the key names as the values of the new array, and use the original key values as the key names of the new array. This article will introduce how to implement this operation in PHP and provide corresponding code examples. Method 1: Use the array_flip function PHP provides a built-in function array_flip, which can be used to exchange key names and key values in the array. under
