Swoole implements high-performance RESTful API server
With the continuous development of the Internet, more and more companies and organizations are beginning to use RESTful APIs to provide data and business services. RESTful API is a concise and clear API design style that communicates through the HTTP protocol, making the interaction between the client and the server very clear and easy to understand. Swoole is a high-performance network communication engine developed based on the PHP language, through which a RESTful API server based on the HTTP protocol can be implemented.
In this article, we will introduce how to use Swoole to implement a high-performance RESTful API server. The article will include the following content:
- What is a RESTful API?
- Basic introduction to Swoole;
- Technical principles of Swoole to implement RESTful API server;
- Swoole steps to implement RESTful API server;
- Can be implemented using Swoole Practical examples of RESTful API servers.
1. What is RESTful API?
RESTful API is an API design style that communicates through the HTTP protocol. It includes the following characteristics:
- Representational State Transfer (REST) : RESTful API implements the addition, deletion, modification and query operations of data resources through HTTP verbs, and returns the request results through HTTP status codes.
- URI address: URI is the resource path of RESTful API, which identifies the API resource to be accessed by the server.
- HTTP request and response: RESTful API uses HTTP protocol for data transmission. The client initiates a request to the server, and the server returns the corresponding response result.
2. Basic introduction to Swoole
Swoole is a high-performance network communication engine developed based on PHP language. Compared with the traditional PHP language, Swoole has the following advantages:
- Based on an asynchronous and non-blocking network programming model;
- Supports multi-process, coroutine and asynchronous IO and other features ;
- Developed based on PHP language, easy to expand and debug.
Therefore, Swoole has a wide range of applications in the fields of network programming, high-concurrency servers and distributed systems.
3. Technical principles of Swoole to implement RESTful API server
The core technology of Swoole to implement RESTful API server is network communication based on HTTP protocol. Swoole implements the following functions through the HTTP protocol:
- Receive the client's HTTP request;
- Parse the URI, request method, request parameters and other information in the HTTP request;
- Process the client's HTTP request and return the HTTP response result.
In addition, Swoole also supports coroutine technology, which can achieve concurrent processing without creating threads, thereby achieving a high-performance RESTful API server.
4. Steps for Swoole to implement RESTful API server
- Install Swoole extension
To install the Swoole extension in a PHP environment, you can use the following command:
pecl install swoole
- Writing a RESTful API server
According to the design principles of RESTful API, the addition, deletion, modification and query operations of the API are implemented. The sample code is as follows:
$request_uri = $_SERVER['REQUEST_URI']; $request_method = $_SERVER['REQUEST_METHOD']; if ($request_method === 'GET') { // 处理GET请求 if ($request_uri === '/api/user') { // 获取用户信息 // TODO } else { // 获取其他资源信息 // TODO } } elseif ($request_method === 'POST') { // 处理POST请求 if ($request_uri === '/api/user') { // 创建用户信息 // TODO } else { // 创建其他资源信息 // TODO } } elseif ($request_method === 'PUT') { // 处理PUT请求 if (preg_match('/^/api/user/d+$/', $request_uri)) { // 更新用户信息 // TODO } else { // 更新其他资源信息 // TODO } } elseif ($request_method === 'DELETE') { // 处理DELETE请求 if (preg_match('/^/api/user/d+$/', $request_uri)) { // 删除用户信息 // TODO } else { // 删除其他资源信息 // TODO } }
Above In the code, we use the basic syntax of PHP to implement a RESTful API server, and handle different API operations by judging the request method and request address.
- Use Swoole to start the RESTful API server
To start the RESTful API server, you can use the following Swoole code:
$server = new SwooleHttpServer('127.0.0.1', 9501); $server->on('Request', function ($request, $response) { $request_uri = $request->server['request_uri']; $request_method = $request->server['request_method']; if ($request_method === 'GET') { // 处理GET请求 if ($request_uri === '/api/user') { // 获取用户信息 // TODO } else { // 获取其他资源信息 // TODO } } elseif ($request_method === 'POST') { // 处理POST请求 if ($request_uri === '/api/user') { // 创建用户信息 // TODO } else { // 创建其他资源信息 // TODO } } elseif ($request_method === 'PUT') { // 处理PUT请求 if (preg_match('/^/api/user/d+$/', $request_uri)) { // 更新用户信息 // TODO } else { // 更新其他资源信息 // TODO } } elseif ($request_method === 'DELETE') { // 处理DELETE请求 if (preg_match('/^/api/user/d+$/', $request_uri)) { // 删除用户信息 // TODO } else { // 删除其他资源信息 // TODO } } $response->end('Hello World'); }); $server->start();
The above code implements a simple RESTful API server, where on('Request', function ($request, $response) {}) listens to HTTP requests and handles business logic in the callback function.
5. Actual cases where Swoole can be used to implement a RESTful API server
The following is a simple example of using Swoole to implement a RESTful API server:
$server = new SwooleHttpServer('0.0.0.0', 9501); $server->on('Request', function ($request, $response) { $method = $request->server['request_method']; $path = $request->server['path_info']; $params = $request->get ?? []; switch ($method) { case 'GET': if ($path == '/api/user') { // 获取用户信息 $response->header("Content-Type", "application/json;charset=utf-8"); $response->end(json_encode($params)); // 假设用户信息存放在$params中 } else { // 获取其他资源信息 $response->status(404); $response->end('Not Found'); } break; case 'POST': if ($path == '/api/user') { // 创建用户信息 $response->status(201); $response->end('Create success'); } else { // 创建其他资源信息 $response->status(400); $response->end('Bad Request'); } break; case 'PUT': if (preg_match('/^/api/user/(d+)$/', $path, $matches)) { // 更新用户信息 $id = $matches[1]; $response->status(200); $response->end("User $id updated"); } else { // 更新其他资源信息 $response->status(400); $response->end('Bad Request'); } break; case 'DELETE': if (preg_match('/^/api/user/(d+)$/', $path, $matches)) { // 删除用户信息 $id = $matches[1]; $response->status(204); $response->end(); } else { // 删除其他资源信息 $response->status(400); $response->end('Bad Request'); } break; default: $response->status(405); $response->header("Allow", "GET,POST,PUT,DELETE"); $response->end('Method Not Allowed'); break; } }); $server->start();
In the above code, We use Swoole's HTTP server and process different API operations by judging different URIs and request methods when listening to HTTP requests. In this way, we can use Swoole to implement a high-performance RESTful API server.
Conclusion
Through the introduction of this article, readers can understand the basic introduction of Swoole, the design principles of RESTful API, and the technical principles and steps of using Swoole to implement RESTful API server. Swoole is very suitable for high-performance network communication needs, so it has a wide range of applications in practical engineering fields. If readers do not have a deep enough understanding of Swoole, they can start with the official documentation and master the usage skills and optimization methods of Swoole through continuous learning and practice, so as to achieve more efficient network communication services.
The above is the detailed content of Swoole implements high-performance RESTful API server. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


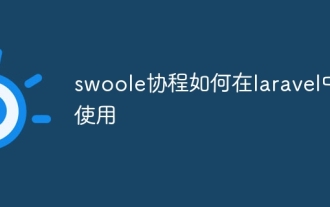
Using Swoole coroutines in Laravel can process a large number of requests concurrently. The advantages include: Concurrent processing: allows multiple requests to be processed at the same time. High performance: Based on the Linux epoll event mechanism, it processes requests efficiently. Low resource consumption: requires fewer server resources. Easy to integrate: Seamless integration with Laravel framework, simple to use.
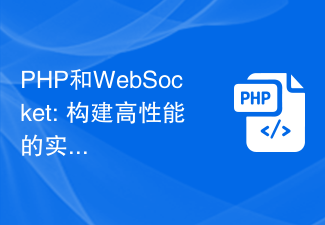
PHP and WebSocket: Building high-performance real-time applications As the Internet develops and user needs increase, real-time applications are becoming more and more common. The traditional HTTP protocol has some limitations when processing real-time data, such as the need for frequent polling or long polling to obtain the latest data. To solve this problem, WebSocket came into being. WebSocket is an advanced communication protocol that provides two-way communication capabilities, allowing real-time sending and receiving between the browser and the server.

Swoole Process allows users to switch. The specific steps are: create a process; set the process user; start the process.
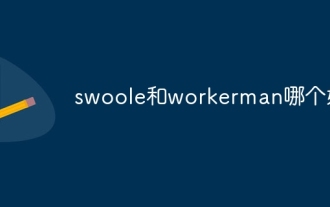
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.
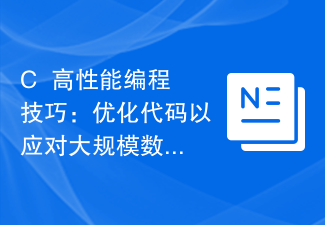
C++ is a high-performance programming language that provides developers with flexibility and scalability. Especially in large-scale data processing scenarios, the efficiency and fast computing speed of C++ are very important. This article will introduce some techniques for optimizing C++ code to cope with large-scale data processing needs. Using STL containers instead of traditional arrays In C++ programming, arrays are one of the commonly used data structures. However, in large-scale data processing, using STL containers, such as vector, deque, list, set, etc., can be more
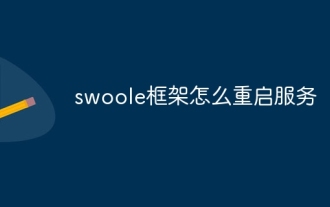
To restart the Swoole service, follow these steps: Check the service status and get the PID. Use "kill -15 PID" to stop the service. Restart the service using the same command that was used to start the service.
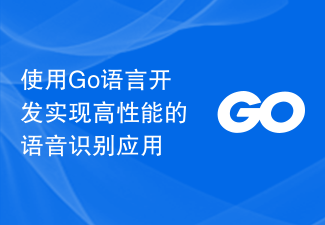
With the continuous development of science and technology, speech recognition technology has also made great progress and application. Speech recognition applications are widely used in voice assistants, smart speakers, virtual reality and other fields, providing people with a more convenient and intelligent way of interaction. How to implement high-performance speech recognition applications has become a question worth exploring. In recent years, Go language, as a high-performance programming language, has attracted much attention in the development of speech recognition applications. The Go language has the characteristics of high concurrency, concise writing, and fast execution speed. It is very suitable for building high-performance
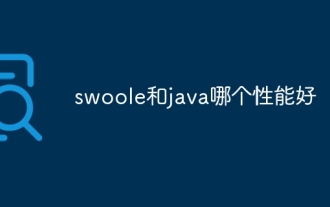
Performance comparison: Throughput: Swoole has higher throughput thanks to its coroutine mechanism. Latency: Swoole's coroutine context switching has lower overhead and smaller latency. Memory consumption: Swoole's coroutines occupy less memory. Ease of use: Swoole provides an easier-to-use concurrent programming API.
