Swoole practice: building a high-performance microservice framework
With the continuous development of Internet technology, microservice architecture has become a current trend. Microservices is a design concept that achieves modular development by splitting a large single application into multiple smaller applications. This model allows teams to build and deploy complex systems faster, with better scalability and maintainability.
Here, we will introduce a method to use Swoole to implement a high-performance microservice architecture. Swoole is an open source, high-performance network framework that can help us implement functions such as asynchronous IO, coroutines, and TCP/UDP network programming. It has good performance and stability, making it an ideal choice for building high-performance microservice architectures.
To demonstrate the application of Swoole in building microservices, we will create a simple microservice application, including an API gateway and multiple service nodes. All service nodes will use Swoole to implement asynchronous communication and high-performance processing.
First, we need to design an API gateway to forward client requests to different service nodes. Swoole-based HTTP servers provide a simple way to implement this functionality. We can write a simple HTTP server that handles client requests and routes them to different services.
In this example, we will use the following route:
- /user/:id - Get the details of a specific user
- /product/:id - Obtain detailed information of a specific product
In routing, :id is a parameter used to locate a specific user or product. We will implement these APIs in our service node.
Next, we need to create multiple service nodes that will handle API requests and respond to clients. Due to Swoole's asynchronous IO and coroutine support, we can use it to implement a fast-response server.
We will create two service nodes: user service and product service. To simplify this example, we will use SQLite as our database.
The user service will provide the following API:
- /user/:id - Get the details of a specific user
- /user/:id/orders - Get All orders of a specific user
The product service will provide the following API:
- /product/:id - Get detailed information of a specific product
We will demonstrate how to use Swoole to create a basic service node. First, we need to create a basic Swoole server and listen on the specified port.
$server = new SwooleServer('0.0.0.0', 8001, SWOOLE_PROCESS, SWOOLE_SOCK_TCP); $server->on('Receive', function (SwooleServer $server, $fd, $reactor_id, $data) { }); $server->start();
When a request arrives, we will handle it in the on('receive') callback. We will parse the HTTP request and extract useful information such as the URL and HTTP method.
$server->on('Receive', function (SwooleServer $server, $fd, $reactor_id, $data) { // 解析HTTP请求 $header_end_pos = strpos($data, " "); $header_data = substr($data, 0, $header_end_pos); $body_data = substr($data, $header_end_pos + 4); $http_parser = new SwooleHttpParser(); $request = $http_parser->execute($header_data, $body_data); // 提取URL和HTTP方法 $path_info = isset($request['server']['path_info']) ? $request['server']['path_info'] : '/'; $http_method = isset($request['server']['request_method']) ? $request['server']['request_method'] : 'GET'; });
Next, we will process these requests and return responses. We need to use Swoole's coroutine feature to implement asynchronous IO within the same request processing cycle. In our example, we will query the SQLite database to obtain user and product information. We can use coroutine client extensions to perform asynchronous queries and writes. Finally, we will return an HTTP response with the correct HTTP status code and response content.
$server->on('Receive', function (SwooleServer $server, $fd, $reactor_id, $data) { // 解析HTTP请求 ... // 处理请求 $response = ['status' => 404, 'content' => 'Not Found']; if (preg_match('/^/user/(d+)/', $path_info, $matches)) { // 查询用户信息 $user_id = $matches[1]; $db = new SwooleCoroutineMySQL(); $db->connect([ 'host' => '127.0.0.1', 'port' => 3306, 'user' => 'root', 'password' => 'password', 'database' => 'test' ]); $result = $db->query("SELECT * FROM users WHERE id = '{$user_id}'"); // 生成响应 if ($result) { $response = ['status' => 200, 'content' => json_encode($result->fetch(), JSON_UNESCAPED_UNICODE)]; } else { $response = ['status' => 404, 'content' => 'Not Found']; } $db->close(); } else if (preg_match('/^/user/(d+)/orders/', $path_info, $matches)) { // 查询用户订单 ... // 生成响应 ... } else if (preg_match('/^/product/(d+)/', $path_info, $matches)) { // 查询商品信息 ... // 生成响应 ... } // 发送响应 $http_response = new SwooleHttpResponse(); $http_response->status($response['status']); $http_response->header('Content-Type', 'application/json'); $http_response->end($response['content']); });
This is a simple example of how to use Swoole to build a high-performance microservice architecture. Swoole provides many features and tools that can help us achieve fast response, high performance and scalable microservice applications.
When using Swoole, we need to pay attention to the following points:
- Always keep the code concise and easy to understand.
- Make full use of Swoole's asynchronous IO and coroutine features to achieve high-performance processing and response.
- Unite multiple service nodes and use API gateway to uniformly manage and route requests.
In this way, we can easily build a high-performance microservice architecture to provide better scalability, maintainability and reliability for our applications.
The above is the detailed content of Swoole practice: building a high-performance microservice framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
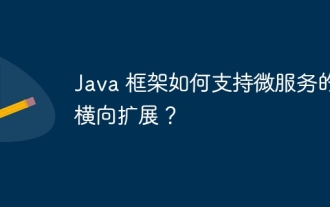
The Java framework supports horizontal expansion of microservices. Specific methods include: Spring Cloud provides Ribbon and Feign for server-side and client-side load balancing. NetflixOSS provides Eureka and Zuul to implement service discovery, load balancing and failover. Kubernetes simplifies horizontal scaling with autoscaling, health checks, and automatic restarts.
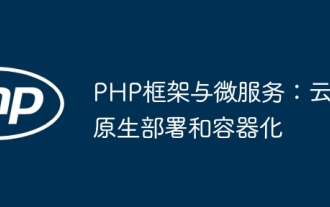
Benefits of combining PHP framework with microservices: Scalability: Easily extend the application, add new features or handle more load. Flexibility: Microservices are deployed and maintained independently, making it easier to make changes and updates. High availability: The failure of one microservice does not affect other parts, ensuring higher availability. Practical case: Deploying microservices using Laravel and Kubernetes Steps: Create a Laravel project. Define microservice controllers. Create Dockerfile. Create a Kubernetes manifest. Deploy microservices. Test microservices.
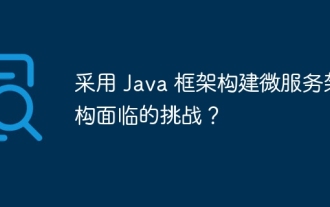
Building a microservice architecture using a Java framework involves the following challenges: Inter-service communication: Choose an appropriate communication mechanism such as REST API, HTTP, gRPC or message queue. Distributed data management: Maintain data consistency and avoid distributed transactions. Service discovery and registration: Integrate mechanisms such as SpringCloudEureka or HashiCorpConsul. Configuration management: Use SpringCloudConfigServer or HashiCorpVault to centrally manage configurations. Monitoring and observability: Integrate Prometheus and Grafana for indicator monitoring, and use SpringBootActuator to provide operational indicators.
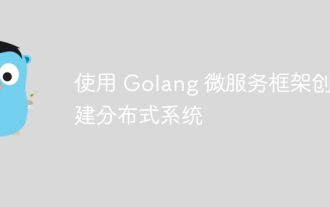
Create a distributed system using the Golang microservices framework: Install Golang, choose a microservices framework (such as Gin), create a Gin microservice, add endpoints to deploy the microservice, build and run the application, create an order and inventory microservice, use the endpoint to process orders and inventory Use messaging systems such as Kafka to connect microservices Use the sarama library to produce and consume order information
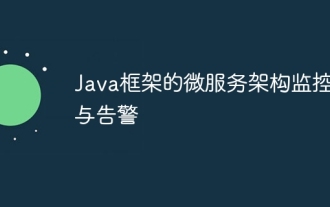
Microservice architecture monitoring and alarming in the Java framework In the microservice architecture, monitoring and alarming are crucial to ensuring system health and reliable operation. This article will introduce how to use Java framework to implement monitoring and alarming of microservice architecture. Practical case: Use SpringBoot+Prometheus+Alertmanager1. Integrate Prometheus@ConfigurationpublicclassPrometheusConfig{@BeanpublicSpringBootMetricsCollectorspringBootMetric
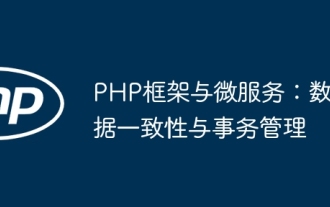
In PHP microservice architecture, data consistency and transaction management are crucial. The PHP framework provides mechanisms to implement these requirements: use transaction classes, such as DB::transaction in Laravel, to define transaction boundaries. Use an ORM framework, such as Doctrine, to provide atomic operations such as the lock() method to prevent concurrency errors. For distributed transactions, consider using a distributed transaction manager such as Saga or 2PC. For example, transactions are used in online store scenarios to ensure data consistency when adding to a shopping cart. Through these mechanisms, the PHP framework effectively manages transactions and data consistency, improving application robustness.
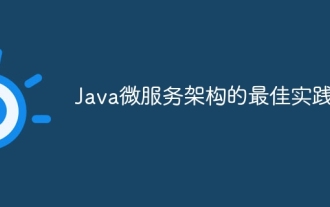
Best Java microservices architecture practices: Use microservices frameworks: Provide structures and tools, such as SpringBoot, Quarkus, Micronaut. Adopt RESTfulAPI: Provide a consistent and standardized interface for cross-service communication. Implement a circuit breaker mechanism: gracefully handle service failures and prevent cascading errors. Use distributed tracing: Monitor requests and dependencies across services for easy debugging and troubleshooting. Automated testing: ensure system robustness and reliability, such as using JUnit. Containerization and orchestration: Use tools like Docker and Kubernetes to simplify deployment and management.
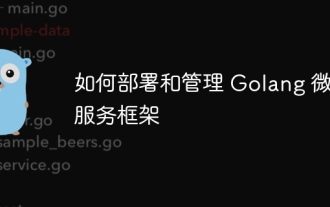
How to deploy and manage the Golang microservices framework to build microservices: Create a Go project and use mockgen to generate a basic service template. Deploy microservices: Deploy using specific commands depending on the platform (e.g. Kubernetes or Docker). Manage microservices: monitoring (Prometheus, Grafana), logging (Jaeger, Zipkin), failover (Istio, Envoy).
