Laravel development: How to use Laravel Cache to implement caching?
Jun 13, 2023 am 10:01 AMLaravel development: How to use Laravel Cache to implement caching?
With the development of web applications, performance issues have become a key issue for modern web applications. Using caching is a common way to solve web application performance problems. Laravel provides a flexible caching solution called caching. Caching is a technique for storing data in temporary storage so that it can be retrieved and displayed faster later. This article will explore how Laravel caching is implemented and used.
Introduction to Laravel Cache
Laravel Cache provides a unified API to use various caching backends (such as Memcached, Redis and file cache). Since Laravel Cache is one of the components of Laravel, caching can be made easier and more convenient. Laravel Cache can be used anywhere in the application to improve the performance of the application.
Laravel Cache configuration
The default backend of Laravel Cache is the file driver. However, we can easily change the cache backend in the config/cache.php file. For example, to use the Memcached cache backend, you can add the following code in the config/cache.php file:
'cache' => [
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
],
In this sample code, we use the Memcached caching backend.
Laravel Cache Usage
Using caching in a Laravel application is very easy. Here is a simple example of how to store data in the cache:
// Storing data
Cache::put('key', 'value', $minutes);
// Get data
$value = Cache::get('key');
// Determine whether the data exists
if (Cache::has('key')) {
1 |
|
}
//Delete data
Cache::forget('key');
In this sample code, Cache::put() method To store data in the cache, the Cache::get() method retrieves the data from the cache, the Cache::has() method checks whether the data exists, and the Cache::forget() method deletes the data from the cache.
Cache Tags
Cache tags are a mechanism for organizing multiple cache items together. It's easy to identify and clear your cache using cache tags. Here's an example of how to use cache tags:
// Store data in cache tags
Cache::tags(['people', 'artists'])->put(' John', $john, $minutes);
Cache::tags(['people', 'authors'])->put('Anne', $anne, $minutes);
// Clean cache tag data
Cache::tags('people')->flush();
In this sample code, we use cache tags to organize data together. Cache tags are specified using the Cache::tags() method, and data can then be stored in the tags using put(). Finally, we can use the flush() method to clear all caches associated with the tag.
Cache validity period
Laravel Cache provides many different methods to set the cache validity period. The most common way is to use the $minutes attribute in the put() method. For example, to store a cache item for 10 minutes, you would use the following code:
// Store cache for 10 minutes
Cache::put('key', 'value', 10);
// Store the permanent cache
Cache::forever('key', 'value');
// Retrieve the value and re-store it
$value = Cache::remember( 'users', $minutes, function () {
1 |
|
});
In this sample code, the Cache::put() method stores the data in the cache, but the cache only retains 10 minutes. The Cache::forever() method stores data in the cache but does not set an expiration time. The Cache::remember() method will look for the "users" item in the cache. If the item does not exist, the callback function is called and placed in the cache. The cached data will be used even if the cache expires.
Conclusion
Laravel Cache is a powerful caching solution that can help improve the performance of web applications. This article introduces the concept and usage of Laravel Cache, and provides sample code to help readers understand how to use Laravel Cache to implement caching. By using Laravel Cache, developers can greatly improve the performance of web applications and provide a better user experience.
The above is the detailed content of Laravel development: How to use Laravel Cache to implement caching?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
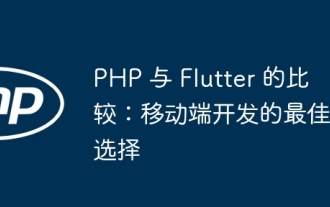
PHP vs. Flutter: The best choice for mobile development

Analysis of the advantages and disadvantages of PHP unit testing tools
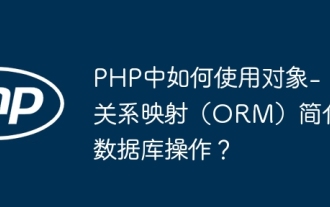
How to use object-relational mapping (ORM) in PHP to simplify database operations?
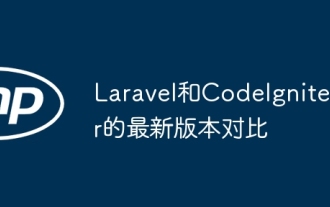
Comparison of the latest versions of Laravel and CodeIgniter
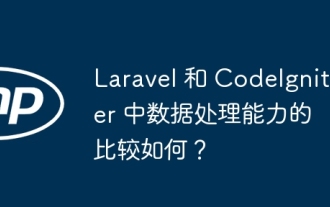
How do the data processing capabilities in Laravel and CodeIgniter compare?
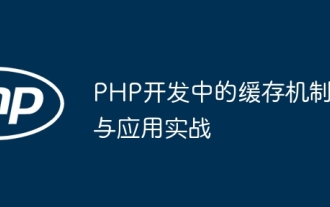
Caching mechanism and application practice in PHP development
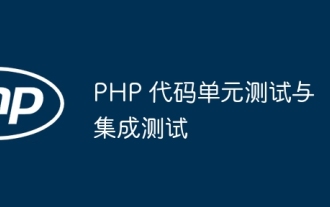
PHP code unit testing and integration testing
