Laravel development: How to define routes using Laravel Routing?
Laravel Development: How to define routes using Laravel Routing?
When we create a Laravel application, defining routes is a necessary and basic step. Laravel Routing's elegance and convenience make defining routes an easy and enjoyable task.
Route is the application's URL mapping, which tells the application how to respond to requests from the client. Through beautiful routing design, we can achieve many functions, such as managing different pages and application functions, determining different ways to respond to requests, and passing parameters.
This article will introduce how to use Laravel Routing to define routes.
Laravel Routing Basics
In Laravel, route definition is usually implemented through routing files (route files). All routing definitions are completed in the route file, which is located in the routes folder of the project directory.
In Laravel, the common routing types are as follows:
- GET request
- POST request
- PUT request
- DELETE request
For each type of request, we can use the corresponding method to define routing. For example, if we want to define a route for a GET request, we can add the following code to the route file:
Route::get('/', function () { return view('welcome'); });
The Route::get() method in the code indicates that we want to define a route for a GET request, followed by The '/' represents the route URL. The route here points to an anonymous function, which returns a view.
When defining the route of a POST request, we can use the Route::post() method. The specific code is as follows:
Route::post('/user', function () { // 创建新用户 });
In this example, we create a new user through a POST request.
The route definitions for PUT requests and DELETE requests are also similar. We can use Route::put() and Route::delete() to define the routes for PUT requests and DELETE requests respectively.
Laravel Routing parameters
In Laravel, we can define some parameters to achieve more flexible routing. For example, we can define a route that accepts one parameter. The specific code is as follows:
Route::get('/user/{id}', function ($id) { return 'User '.$id; });
This route points to an anonymous function. The function accepts a parameter $id, and the routing URL is /user/{id}. For example, when we access /user/1, the returned information is "User 1", indicating that the route has been successfully matched.
We can also define multiple parameters in the route. The specific code is as follows:
Route::get('/user/{id}/{name}', function ($id, $name) { return 'User '.$id . ' Name: ' . $name; });
This route points to an anonymous function. The function accepts two parameters $id and $name. The routing URL is /user/{id}/{name}. For example, when we access /user/1/john, the returned information is "User 1 Name: john".
Laravel Routing named routing
In Laravel, we can name the route to make it easier to reference and jump. For example, we can name the previously defined route that accepts parameters. The specific code is as follows:
Route::get('/user/{id}', function ($id) { return 'User '.$id; })->name('user.profile');
The ->name() method in this code is used to specify the route name. We name this route user.profile.
We can use the route() function to reference the named route. The specific code is as follows:
$url = route('user.profile', ['id' => 1]);
In this code, we use the route() function to generate a URL for the named route. We can pass the required parameters to the route() function as the second parameter.
When we call the route() function, Laravel will return a complete URL, for example: http://your-app-url/user/1. The 1 here is the parameter we passed to the route() function.
Laravel Routing middleware
In Laravel, we can filter routes through middleware. Middleware is a mechanism that performs some action before or after a request is passed to a Laravel application.
For example, we can add an authentication middleware to route requests to ensure that the user has been authenticated. The specific code is as follows:
Route::get('/dashboard', function () { // })->middleware(['auth']);
The middleware(['auth']) method in this code is used to specify the middleware in the route. In the above example, we specify middleware as auth to ensure that the user has been authenticated before accessing the dashboard route.
We can add multiple middlewares by calling the middleware() method. The specific code is as follows:
Route::get('/dashboard', function () { // })->middleware(['auth', 'admin']);
The middleware() method in this code specifies two middlewares: auth and admin . This means that the user must be authenticated and granted administrator privileges to access the dashboard route.
Conclusion
In Laravel development, it is crucial to understand how to define Routing. With Laravel Routing, we are able to define elegant and flexible URL key-value pairs for our applications and add various filters and middleware to requests.
In this article, we introduced the basics of Laravel Routing, parameters, named routes, and middleware. I hope this content is helpful when you use Laravel Routing to define routes.
The above is the detailed content of Laravel development: How to define routes using Laravel Routing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
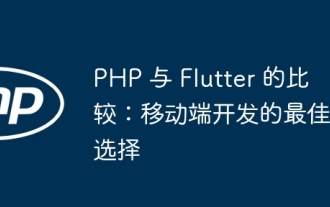
PHP and Flutter are popular technologies for mobile development. Flutter excels in cross-platform capabilities, performance and user interface, and is suitable for applications that require high performance, cross-platform and customized UI. PHP is suitable for server-side applications with lower performance and not cross-platform.
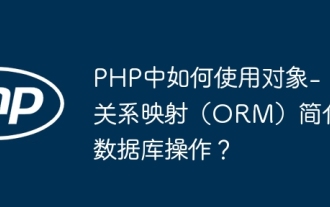
Database operations in PHP are simplified using ORM, which maps objects into relational databases. EloquentORM in Laravel allows you to interact with the database using object-oriented syntax. You can use ORM by defining model classes, using Eloquent methods, or building a blog system in practice.
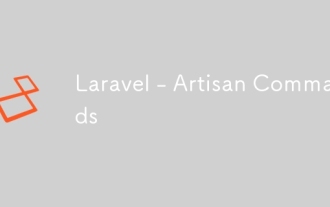
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?

PHP unit testing tool analysis: PHPUnit: suitable for large projects, provides comprehensive functionality and is easy to install, but may be verbose and slow. PHPUnitWrapper: suitable for small projects, easy to use, optimized for Lumen/Laravel, but has limited functionality, does not provide code coverage analysis, and has limited community support.
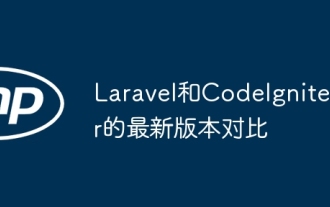
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
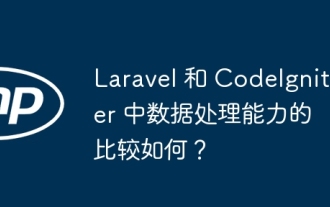
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
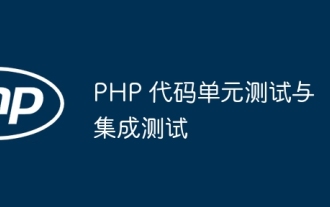
PHP Unit and Integration Testing Guide Unit Testing: Focus on a single unit of code or function and use PHPUnit to create test case classes for verification. Integration testing: Pay attention to how multiple code units work together, and use PHPUnit's setUp() and tearDown() methods to set up and clean up the test environment. Practical case: Use PHPUnit to perform unit and integration testing in Laravel applications, including creating databases, starting servers, and writing test code.
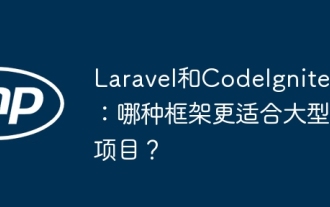
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
