Swoole in action: quickly create a chat room based on WebSocket
In the Internet era, chat rooms have become an important place for people to communicate and socialize. The emergence of WebSocket technology has made real-time communication smoother and more stable. Today, we introduce how to use the Swoole framework to quickly build a chat room based on WebSocket.
Swoole is a high-performance PHP coroutine network communication framework, written in C language, integrating asynchronous IO, coroutine, network communication and other functions, allowing PHP code to be processed as efficiently as Node.js Event-driven asynchronous concurrent programming. It can be said that Swoole is an important tool for developing high-concurrency network applications.
Below, we will introduce step by step how to use Swoole to implement a chat room based on WebSocket and support multi-person online chat.
- Environment preparation
Before you start, you need to make sure you have installed the Swoole extension and enabled WebSocket support.
The installation method is as follows:
pecl install swoole
Or compile and install:
wget https://pecl.php.net/get/swoole-{version}.tgz tar xzvf swoole-{version}.tgz cd swoole-{version} phpize ./configure --enable-async-redis --enable-coroutine --enable-openssl --enable-http2 --enable-sockets make && make install
If Docker is used, you can add the following statements in the Dockerfile:
RUN pecl install swoole && docker-php-ext-enable swoole && docker-php-ext-install pcntl
- Client Page
First, we need to write a page for sending messages to the chat room. The code is as follows:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>WebSocket ChatRoom Demo</title> <style> * { margin: 0; padding: 0; } .container { margin: 30px auto; width: 800px; height: 600px; border: 1px solid #aaa; border-radius: 5px; overflow: hidden; } .message-box { width: 800px; height: 500px; border-bottom: 1px solid #aaa; overflow-y: scroll; } .input-box { width: 800px; height: 100px; border-top: 1px solid #aaa; } .input-text { width: 600px; height: 80px; margin: 10px; padding: 10px; font-size: 20px; border-radius: 5px; border: 1px solid #aaa; outline: none; } .send-btn { width: 100px; height: 100%; margin: 0 10px; background-color: #4CAF50; border: none; color: white; font-size: 18px; border-radius: 5px; cursor: pointer; } </style> </head> <body> <div class="container"> <div class="message-box"></div> <div class="input-box"> <textarea class="input-text"></textarea> <button class="send-btn">Send</button> </div> </div> <script> // 初始化WebSocket let socket = new WebSocket('ws://localhost:9502'); // 监听连接成功事件 socket.onopen = function (event) { console.log('WebSocket connection established.'); } // 监听服务端发送的消息 socket.onmessage = function (event) { let message_box = document.querySelector('.message-box'); message_box.innerHTML += `<p>${event.data}</p>`; message_box.scrollTop = message_box.scrollHeight; } // 监听连接关闭事件 socket.onclose = function (event) { console.log('WebSocket connection closed.'); } // 发送消息 let send_btn = document.querySelector('.send-btn'); let input_text = document.querySelector('.input-text'); send_btn.addEventListener('click', function (event) { if (input_text.value.trim() == '') return; socket.send(input_text.value); input_text.value = ''; }); </script> </body> </html>
In this code, we divide the chat room page into two parts: the message display box and the message input box. At the same time, the related logic of WebSocket connection and message sending is defined.
It should be noted that when deploying in a local environment, the address of WebSocket
needs to be modified to the local IP address instead of localhost
. If you want to use an online environment, you need to change the WebSocket
address to the server's public IP.
- Server-side code
Next, we write the server-side code. Through the class library provided by Swoole, we can easily create a WebSocket server. The code is as follows:
<?php // 创建WebSocket服务器 $server = new SwooleWebsocketServer("0.0.0.0", 9502); // 监听WebSocket连接打开事件 $server->on('open', function (SwooleWebsocketServer $server, $request) { echo "connection open: {$request->fd} "; }); // 监听WebSocket消息事件 $server->on('message', function (SwooleWebsocketServer $server, $frame) { echo "received message: {$frame->data} "; // 广播消息 foreach ($server->connections as $fd) { $server->push($fd, $frame->data); } }); // 监听WebSocket连接关闭事件 $server->on('close', function (SwooleWebsocketServer $server, $fd) { echo "connection close: {$fd} "; }); // 启动WebSocket服务器 $server->start();
First, we create a WebSocket server and bind it to the address 0.0.0.0:9502
to wait for client connections. The three events of WebSocket connection opening, message, and connection closing are monitored through the on
method, and the processing logic for these three events has been implemented.
In the open
event, we use the client fd recorded by Swoole to output it to the console.
In the message
event, we obtain the information from the client, use echo
to output it to the console, and pass foreach
Traverse the clients that have established connections and broadcast the message to all clients.
In the close
event, we again use the client fd recorded by Swoole and output it to the console.
Finally, we use the start
method to start the WebSocket server.
- Conclusion
So far, we have implemented a multi-person online chat room based on WebSocket. In the client page, you can send any message, and the message will be broadcast to all online clients for display.
Through the Swoole framework, we can easily create an efficient WebSocket server, which provides a convenient means to achieve high performance, low latency, and reliable real-time communication.
The above is the detailed content of Swoole in action: quickly create a chat room based on WebSocket. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
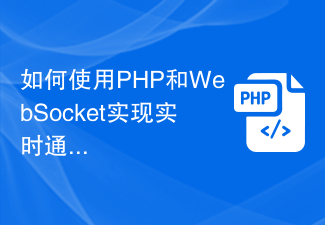
With the continuous development of Internet technology, real-time communication has become an indispensable part of daily life. Efficient, low-latency real-time communication can be achieved using WebSockets technology, and PHP, as one of the most widely used development languages in the Internet field, also provides corresponding WebSocket support. This article will introduce how to use PHP and WebSocket to achieve real-time communication, and provide specific code examples. 1. What is WebSocket? WebSocket is a single
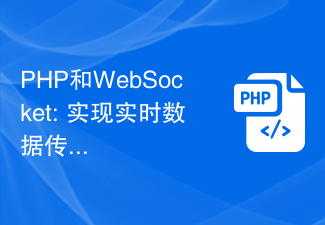
PHP and WebSocket: Best Practice Methods for Real-Time Data Transfer Introduction: In web application development, real-time data transfer is a very important technical requirement. The traditional HTTP protocol is a request-response model protocol and cannot effectively achieve real-time data transmission. In order to meet the needs of real-time data transmission, the WebSocket protocol came into being. WebSocket is a full-duplex communication protocol that provides a way to communicate full-duplex over a single TCP connection. Compared to H
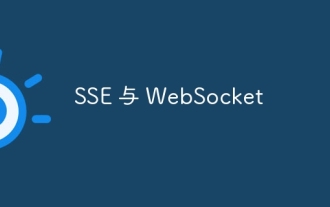
In this article, we will compare Server Sent Events (SSE) and WebSockets, both of which are reliable methods for delivering data. We will analyze them in eight aspects, including communication direction, underlying protocol, security, ease of use, performance, message structure, ease of use, and testing tools. A comparison of these aspects is summarized as follows: Category Server Sent Event (SSE) WebSocket Communication Direction Unidirectional Bidirectional Underlying Protocol HTTP WebSocket Protocol Security Same as HTTP Existing security vulnerabilities Ease of use Setup Simple setup Complex performance Fast message sending speed Affected by message processing and connection management Message structure Plain text or binary Ease of use Widely available Helpful for WebSocket integration
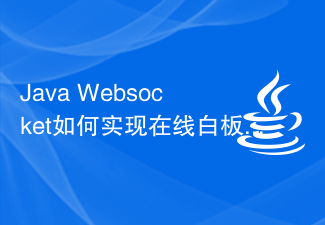
How does JavaWebsocket implement online whiteboard function? In the modern Internet era, people are paying more and more attention to the experience of real-time collaboration and interaction. Online whiteboard is a function implemented based on Websocket. It enables multiple users to collaborate in real-time to edit the same drawing board and complete operations such as drawing and annotation. It provides a convenient solution for online education, remote meetings, team collaboration and other scenarios. 1. Technical background WebSocket is a new protocol provided by HTML5. It implements
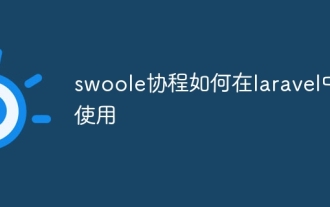
Using Swoole coroutines in Laravel can process a large number of requests concurrently. The advantages include: Concurrent processing: allows multiple requests to be processed at the same time. High performance: Based on the Linux epoll event mechanism, it processes requests efficiently. Low resource consumption: requires fewer server resources. Easy to integrate: Seamless integration with Laravel framework, simple to use.
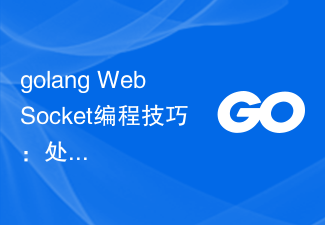
Golang is a powerful programming language, and its use in WebSocket programming is increasingly valued by developers. WebSocket is a TCP-based protocol that allows two-way communication between client and server. In this article, we will introduce how to use Golang to write an efficient WebSocket server that handles multiple concurrent connections at the same time. Before introducing the techniques, let's first learn what WebSocket is. Introduction to WebSocketWeb
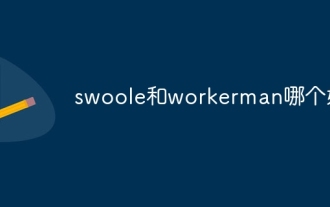
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.
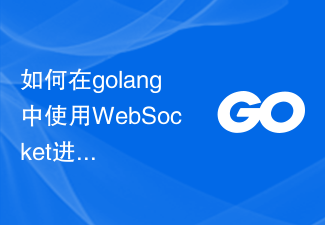
How to use WebSocket for file transfer in golang WebSocket is a network protocol that supports two-way communication and can establish a persistent connection between the browser and the server. In golang, we can use the third-party library gorilla/websocket to implement WebSocket functionality. This article will introduce how to use golang and gorilla/websocket libraries for file transfer. First, we need to install gorilla
