VUE3 Getting Started Example: Making a Simple Image Cropper
Vue.js is a popular JavaScript front-end framework. The latest version - Vue3 has been launched. The new version of Vue has improved performance, size and development experience, and is welcomed by more and more developers. . This article will introduce how to use Vue3 to make a simple image cropper.
First, we need to create a Vue project and install the required plugins. You can use Vue CLI to create the project, or you can build it manually. Here we take the use of Vue CLI as an example:
# 安装Vue CLI npm install -g @vue/cli # 创建Vue项目 vue create image-cropper # 进入项目文件夹 cd image-cropper # 安装所需插件 npm install vue-cropperjs --save npm install cropperjs --save
Vue-Cropperjs is a plug-in for cropping images, and Cropperjs is the core library of Vue-Cropperjs and needs to be installed together.
Next, we need to introduce the Vue-Cropperjs plug-in into the Vue project. Modify the src/main.js
file as follows:
import Vue from 'vue' import App from './App.vue' import VueCropper from 'vue-cropperjs' import 'cropperjs/dist/cropper.css' Vue.use(VueCropper) Vue.config.productionTip = false new Vue({ render: h => h(App), }).$mount('#app')
In the above code, we introduced the Vue-Cropperjs plug-in and called the Vue.use() method in Vue to register. It should be noted that here we also introduce the style file of Cropperjs to ensure the normal operation of the image cropper.
Next, we need to create an image cropper component in Vue. Create a new CropImage.vue
file in the src/views
directory and add the following code:
<template> <div> <div ref="wrapper"> <img ref="img" :src="src" style="max-width: 100%;" /> </div> <div> <input type="file" @change="onUpload" /> </div> <div> <vue-cropper ref="cropper" :src="src" :auto-crop-area="0.5" :guides="false" :view-mode="1" :drag-mode="dragMode" :crop-box-movable="false" :crop-box-resizable="false" :crop-box-border-radius="50"></vue-cropper> </div> <div> <button @click="onCrop">裁剪</button> </div> </div> </template> <script> export default { name: 'CropImage', data() { return { src: '', cropper: null, dragMode: 'move' } }, methods: { onUpload(e) { const file = e.target.files[0] if (file.type.match(/image.*/)) { const reader = new FileReader() reader.onload = (event) => { this.src = event.target.result } reader.readAsDataURL(file) } }, onCrop() { const canvas = this.$refs.cropper.getCroppedCanvas({ width: 100, height: 100 }) const dataUrl = canvas.toDataURL() console.log(dataUrl) } } } </script>
In the above code, we created a file named CropImage
Vue component, which contains three main elements:
- Image container
- Image upload button
- Image cropper
With the img
tag and a div
tag, we create an initial image container. Users can click the "Upload" button to select an image for cropping. When the user selects the image, we use FileReader to convert the image to base64 encoding and assign it to the src
attribute to preview the image.
The image cropper uses the vue-cropper
tag provided in the Vue-Cropperjs plug-in, which supports multiple attributes to control the performance of the cropper, such as: auto-crop- area
controls the area ratio of automatic cropping, guides
controls whether to display the cropping frame auxiliary lines, view-mode
controls the mode of the cropper, etc. In addition, we also set the movement mode of the crop box to "Move" to ensure that users can better operate the crop box.
The crop button is bound to the onCrop
method, which outputs the base64 encoding of the cropped image to the console. Developers can rewrite this method according to actual needs.
Finally we need to introduce the CropImage.vue
component into the App.vue
file. Modify the src/App.vue
file as follows:
<template> <div id="app"> <CropImage /> </div> </template> <script> import CropImage from './views/CropImage.vue' export default { name: 'App', components: { CropImage } } </script> <style> #app { max-width: 640px; margin: 0 auto; padding: 20px; } </style>
In the above code, we introduce the CropImage
component into the App.vue
file, And pass parameters through props
in the component tag to initialize the image cropper.
So far, we have completed the production of a simple image cropper, which can run normally and perform cropping. Of course, this is just an introductory example. Beginners can further understand the use and development skills of Vue3 by modifying and extending the code.
The above is the detailed content of VUE3 Getting Started Example: Making a Simple Image Cropper. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


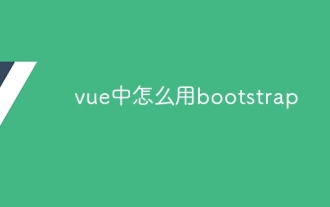
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
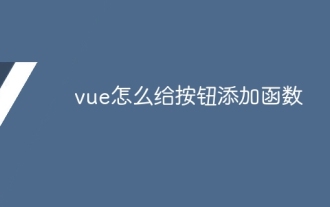
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
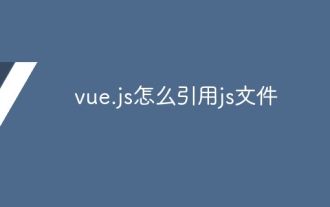
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
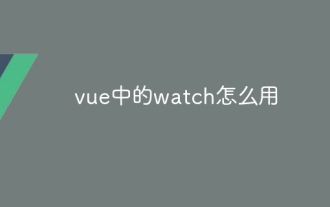
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
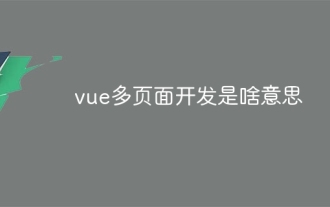
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
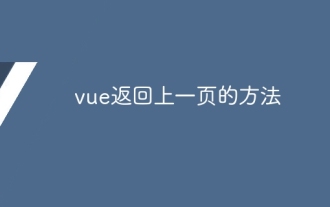
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
