Implementing global variable safety in JavaScript
With the popularity of JavaScript, more and more websites and applications rely on JavaScript. However, the use of global variables in JavaScript can have security issues. In this article, I will introduce how to implement global variable safety in JavaScript.
- Avoid using global variables
The best way is to avoid using global variables. In JavaScript, all variables are global by default unless they are declared within a function. Therefore, local variables should be used instead of global variables whenever possible. This will make the code easier to maintain and debug, and reduce possible security vulnerabilities.
- Encapsulating global variables
If you must use global variables, the best thing to do is to encapsulate them, which ensures that access to global variables is restricted. For example, you can encapsulate global variables in a function:
function myFunction() { var globalVariable = "I am a global variable."; // your code here }
Doing so ensures that global variables can only be used in the myFunction
function, thereby increasing code security.
- Use ES6's let and const
In ES6, two new keywords, let and const, are introduced, both of which are block-level scope variables. Use them to make variables visible only within the scope of a specified code block, thereby reducing variable pollution and eliminating possible security holes.
function myFunction() { let localVariable = "I am a local variable."; // your code here }
In this example, localVariable
is only visible within the myFunction
function, not the global variable.
- Using closures
Another mechanism for protecting global variables is to use closures. A closure encapsulates a function and its execution environment in a package, thereby protecting internal variables from contamination and attacks by the external environment.
function myFunction() { var globalVariable = "I am a global variable."; return function() { // your code here } } var myClosure = myFunction();
In this example, the myFunction
function returns a closure that can access the globalVariable
variable. Because the variable is encapsulated in a closure, it can only be accessed through the closure, thus ensuring the safety of the variable.
In summary, although there may be security issues when using global variables in JavaScript, there are many ways to ensure the security of global variables. Understanding these methods and using them in your coding can make your code more robust and reliable.
The above is the detailed content of Implementing global variable safety in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


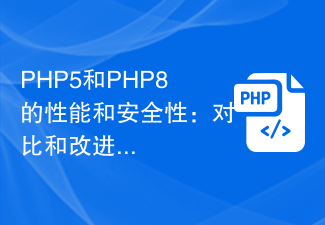
PHP is a widely used server-side scripting language used for developing web applications. It has developed into several versions, and this article will mainly discuss the comparison between PHP5 and PHP8, with a special focus on its improvements in performance and security. First let's take a look at some features of PHP5. PHP5 was released in 2004 and introduced many new functions and features, such as object-oriented programming (OOP), exception handling, namespaces, etc. These features make PHP5 more powerful and flexible, allowing developers to
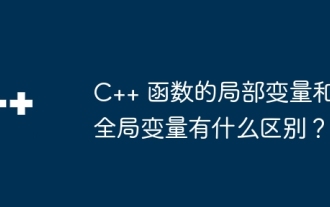
The difference between C++ local variables and global variables: Visibility: Local variables are limited to the defining function, while global variables are visible throughout the program. Memory allocation: local variables are allocated on the stack, while global variables are allocated in the global data area. Scope: Local variables are within a function, while global variables are throughout the program. Initialization: Local variables are initialized when a function is called, while global variables are initialized when the program starts. Recreation: Local variables are recreated on every function call, while global variables are created only when the program starts.
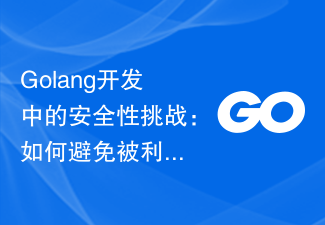
Security challenges in Golang development: How to avoid being exploited for virus creation? With the wide application of Golang in the field of programming, more and more developers choose to use Golang to develop various types of applications. However, like other programming languages, there are security challenges in Golang development. In particular, Golang's power and flexibility also make it a potential virus creation tool. This article will delve into security issues in Golang development and provide some methods to avoid G
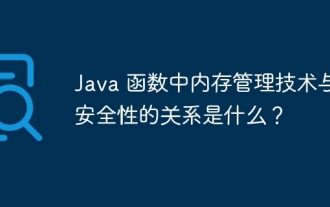
Memory management in Java involves automatic memory management, using garbage collection and reference counting to allocate, use and reclaim memory. Effective memory management is crucial for security because it prevents buffer overflows, wild pointers, and memory leaks, thereby improving the safety of your program. For example, by properly releasing objects that are no longer needed, you can avoid memory leaks, thereby improving program performance and preventing crashes.
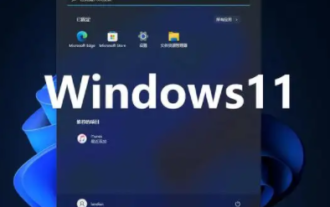
Win11 comes with anti-virus software. Generally speaking, the anti-virus effect is very good and does not need to be installed. However, the only disadvantage is that the virus is uninstalled first instead of reminding you in advance whether you need it. If you accept it, you don’t need to download it. Other anti-virus software. Does win11 need to install anti-virus software? Answer: No. Generally speaking, win11 comes with anti-virus software and does not require additional installation. If you don’t like the way the anti-virus software that comes with the win11 system is handled, you can reinstall it. How to turn off the anti-virus software that comes with win11: 1. First, we enter settings and click "Privacy and Security". 2. Then click "Window Security Center". 3. Then select “Virus and threat protection”. 4. Finally, you can turn it off
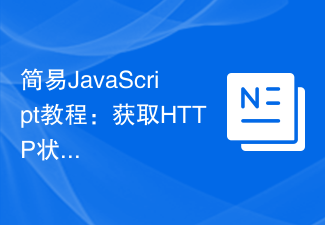
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
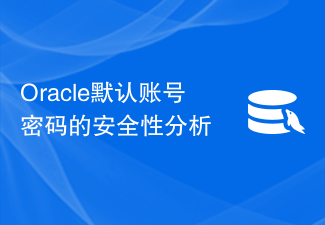
Oracle database is a popular relational database management system. Many enterprises and organizations choose to use Oracle to store and manage their important data. In the Oracle database, there are some default accounts and passwords preset by the system, such as sys, system, etc. In daily database management and operation and maintenance work, administrators need to pay attention to the security of these default account passwords, because these accounts have higher permissions and may cause serious security problems once they are maliciously exploited. This article will cover Oracle default
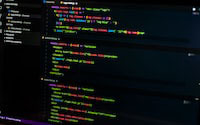
What is EJB? EJB is a Java Platform, Enterprise Edition (JavaEE) specification that defines a set of components for building server-side enterprise-class Java applications. EJB components encapsulate business logic and provide a set of services for handling transactions, concurrency, security, and other enterprise-level concerns. EJB Architecture EJB architecture includes the following major components: Enterprise Bean: This is the basic building block of EJB components, which encapsulates business logic and related data. EnterpriseBeans can be stateless (also called session beans) or stateful (also called entity beans). Session context: The session context provides information about the current client interaction, such as session ID and client
