


Implementing efficient asynchronous programming patterns using Go language
Use Go language to implement efficient asynchronous programming model
With the rapid development of Internet technology, the performance and scalability requirements of modern applications are getting higher and higher. To address this challenge, asynchronous programming has become standard in modern programming languages. The Go language is a language naturally suitable for asynchronous programming. Its simple syntax and efficient concurrency mechanism have become the choice of many developers.
The advantage of the asynchronous programming model is that it can improve the response speed and throughput of the system. But at the same time, asynchronous programming also increases the complexity of the code. In Go language, we can achieve efficient asynchronous programming by using goroutine and channel.
- goroutine
Goroutine is a lightweight execution unit in the Go language. Compared with threads in other languages, goroutines are more lightweight and can be created and destroyed faster. In the Go language, millions of goroutines can be easily created without much overhead.
The way to create a goroutine is very simple, just add the go keyword before the function call. For example:
func main(){ go func(){ //code }() }
- channel
channel is a mechanism in the Go language for communication between goroutines. Channel can realize synchronous and asynchronous communication, and using channel can avoid the problem of shared memory, so it can effectively avoid data competition.
The creation method of channel is also very simple, just use the make function.
c := make(chan int)
The sending and receiving operations of channel use the <- symbol, for example:
c <- value // 发送value到channel v := <-c // 从channel中接收值并赋值给v
Using channel can achieve synchronization and communication between multiple goroutines. For example, in the following example, we can use a channel to synchronize the execution of two goroutines:
func main() { c := make(chan int) go func() { c <- 1 // send 1 to channel c }() v := <-c // receive from channel c fmt.Println(v) // output: 1 }
- select
In the Go language, select is used Perform non-blocking operations on multiple channels. The syntax of select is similar to switch, but the expression after the case must be a channel operation, for example:
select { case v := <-ch1: fmt.Println("received from ch1: ", v) case v := <-ch2: fmt.Println("received from ch2: ", v) }
If multiple cases can be executed, select will randomly select one of them. If there is no case in the select that can be executed, it will block until at least one case can be executed.
When using select, we can use the default statement to handle some special situations. For example:
select { case v := <-ch1: fmt.Println("received from ch1: ", v) case v := <-ch2: fmt.Println("received from ch2: ", v) default: fmt.Println("no message received") }
- Sample code
The following is an example of using goroutine and channel to implement asynchronous programming. In this example, we will start multiple goroutines to calculate the Fibonacci sequence. After the calculation is completed, use the channel to transfer the calculation results to the main goroutine. Finally, the main goroutine will output the calculation results to the console.
package main import ( "fmt" ) func fibonacci(n int, c chan int) { if n < 2 { c <- n return } c1 := make(chan int) c2 := make(chan int) go fibonacci(n-1, c1) go fibonacci(n-2, c2) x, y := <-c1, <-c2 c <- x + y } func main() { n := 10 c := make(chan int) go fibonacci(n, c) res := <-c fmt.Printf("fibonacci(%d)=%d ", n, res) }
In the above example, we used a recursive method to calculate the Fibonacci sequence, and used two channels to synchronize the calculation. In the main goroutine, we only need to wait for the calculation results, and the whole process is very simple and efficient.
Summary
The Go language provides an efficient asynchronous programming model, allowing developers to easily implement high-performance and scalable applications. By using goroutine, channel and select, we can easily implement asynchronous programming. When using Go language for development, it is recommended to use asynchronous programming mode to give full play to the advantages of Go language and make the program more efficient and robust.
The above is the detailed content of Implementing efficient asynchronous programming patterns using Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


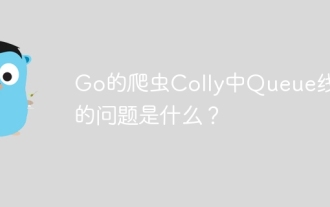
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
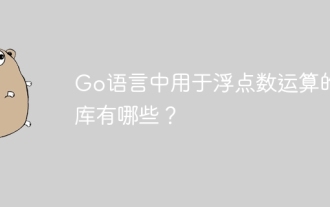
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
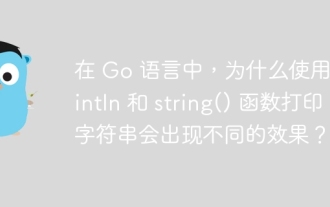
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
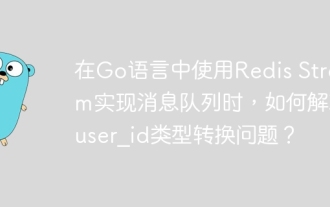
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
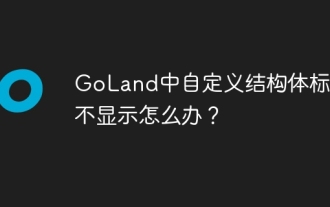
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
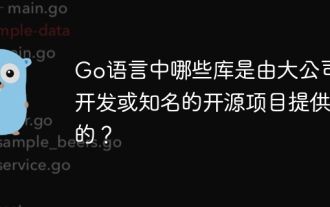
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
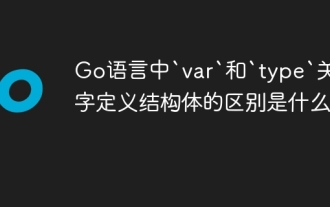
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
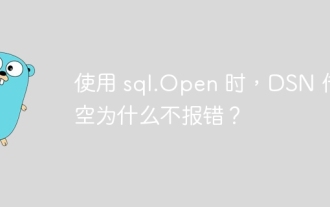
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
