How to implement a robot assistant program using Java
In the information age, computer technology has become more and more popular, especially in automation applications. The use of robots has been widely used in life and industry. Some of these robots rely on programs for assistance or control operations. Among many programming languages, Java can be said to be a very popular language. I believe everyone will know it when learning programming. Although implementing robots in Java may be a bit complicated, in fact, as long as you follow the tutorial step by step, you can develop a relatively practical robot auxiliary program. Below I will describe in detail how to use Java to implement a robot auxiliary program.
Step One: Java Preparation
Before proceeding with the Java robot auxiliary program, we need to prepare the necessary tools and software. Here we need to download the Java development tools and Java Robot class library.
Step 2: Use the Java Robot class library
The Java Robot class is a class that handles local system input events. Using this class, you can simulate mouse and keyboard events, and at the same time, you can manipulate the color and color of the screen. Pixel values. Therefore, you can use the Robot class in Java to implement the robot's auxiliary program. I will introduce the specific steps below.
- First, we need to import the Robot class library into the Java program code, import java.awt.Robot;
- Then, create a Robot class instance in the program, instantiate, Robot bot = new Robot();
- Now enable the robot to simulate mouse clicks, you can use mouse and keyboard events, such as left mouse button click, bot.mousePress(MouseEvent.BUTTON1_MASK);bot.mouseRelease(MouseEvent. BUTTON1_MASK). In this way, the robot can complete the click event;
- The important point in implementing the robot auxiliary program is scene recognition, that is, identifying the scene of the application. Generally speaking, we can use image processing algorithms (such as OpenCV) or find some software to assist. For example, we use the window snapshot tool provided in the Windows application to take a screenshot and save it locally;
- Robots often need to inject programs, such as simulating the Ctrl C and Ctrl V key combinations to paste and copy the contents of the clipboard . And it requires frequent mouse movement. These operations can be implemented through instantiated objects of the Java Robot class.
Step 3: Implementation of the robot auxiliary program code
Through the above steps, you have been able to use Java to implement the robot auxiliary program. Below I will give an example of the Java robot auxiliary program code:
public class RobotExample {
private Robot robot = null;
public void init() {
try { robot = new Robot(); } catch (Exception e) { e.printStackTrace(); }
}
public void mouseClick(int x, int y) {
robot.mouseMove(x, y); robot.mousePress(InputEvent.BUTTON1_MASK); robot.mouseRelease(InputEvent.BUTTON1_MASK);
}
public void keyPress(int[] keys) {
for(int key : keys) { robot.keyPress(key); robot.keyRelease(key); }
}
public void typeString (String s) {
for(char c : s.toCharArray()) { robot.keyPress(c); robot.keyRelease(c); }
}
public static void main(String[] args) {
RobotExample robotExample = new RobotExample(); robotExample.init(); robotExample.mouseClick(312,225); robotExample.keyPress(new int[] {KeyEvent.VK_CONTROL, KeyEvent.VK_C}); robotExample.typeString("Hello, World!"); robotExample.keyPress(new int[] {KeyEvent.VK_CONTROL, KeyEvent.VK_V});
}
}
After the above code operation, The robot can replace humans in mouse operations, simulate keyboard keystrokes and other behaviors, thereby achieving the effect of a robot-assisted program.
Summary
Java is a powerful programming language that is very flexible in application implementation. The Java Robot class provides the function of processing local system input events, can simulate mouse and keyboard events, and manipulate the color and pixel values of the screen. Therefore, using the Robot class in Java can implement the robot's auxiliary program. However, please note that you need to be careful when using robots for automated testing to avoid adverse effects on others. At the same time, in order to ensure the stability of the program, it is recommended that sufficient testing be required during development to ensure the stability and reliability of the code.
The above is the detailed content of How to implement a robot assistant program using Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
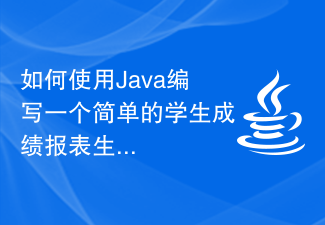
How to write a simple student performance report generator using Java? Student Performance Report Generator is a tool that helps teachers or educators quickly generate student performance reports. This article will introduce how to use Java to write a simple student performance report generator. First, we need to define the student object and student grade object. The student object contains basic information such as the student's name and student number, while the student score object contains information such as the student's subject scores and average grade. The following is the definition of a simple student object: public
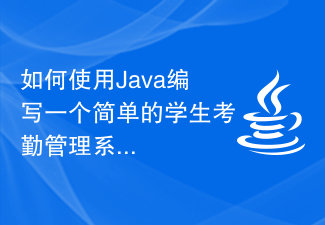
How to write a simple student attendance management system using Java? With the continuous development of technology, school management systems are also constantly updated and upgraded. The student attendance management system is an important part of it. It can help the school track students' attendance and provide data analysis and reports. This article will introduce how to write a simple student attendance management system using Java. 1. Requirements Analysis Before starting to write, we need to determine the functions and requirements of the system. Basic functions include registration and management of student information, recording of student attendance data and
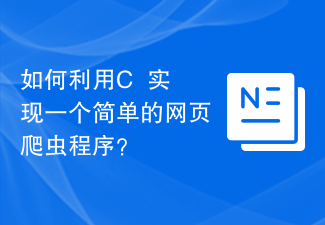
How to use C++ to implement a simple web crawler program? Introduction: The Internet is a treasure trove of information, and a large amount of useful data can be easily obtained from the Internet through web crawlers. This article will introduce how to use C++ to write a simple web crawler program, as well as some common tips and precautions. 1. Preparation to install a C++ compiler: First, you need to install a C++ compiler on your computer, such as gcc or clang. You can enter "g++-v" or "clang" through the command line
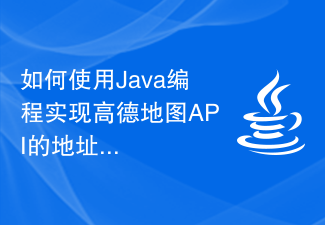
How to use Java programming to implement the address location search of the Amap API Introduction: Amap is a very popular map service and is widely used in various applications. Among them, the search function near the address location provides the ability to search for nearby POI (Point of Interest, points of interest). This article will explain in detail how to use Java programming to implement the address location search function of the Amap API, and use code examples to help readers understand and master related technologies. 1. Apply for Amap development
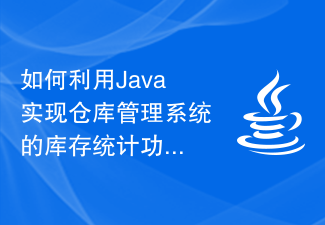
How to use Java to implement the inventory statistics function of the warehouse management system. With the development of e-commerce and the increasing importance of warehousing management, the inventory statistics function has become an indispensable part of the warehouse management system. Warehouse management systems written in the Java language can implement inventory statistics functions through concise and efficient code, helping companies better manage warehouse storage and improve operational efficiency. 1. Background introduction Warehouse management system refers to a management method that uses computer technology to perform data management, information processing and decision-making analysis on an enterprise's warehouse. Inventory statistics are
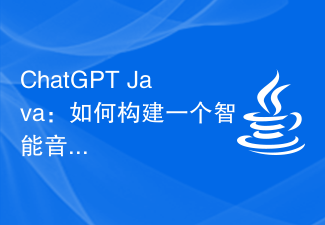
ChatGPTJava: How to build an intelligent music recommendation system, specific code examples are needed. Introduction: With the rapid development of the Internet, music has become an indispensable part of people's daily lives. As music platforms continue to emerge, users often face a common problem: how to find music that suits their tastes? In order to solve this problem, the intelligent music recommendation system came into being. This article will introduce how to use ChatGPTJava to build an intelligent music recommendation system and provide specific code examples. No.
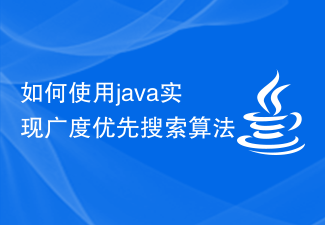
How to use Java to implement breadth-first search algorithm Breadth-First Search algorithm (Breadth-FirstSearch, BFS) is a commonly used search algorithm in graph theory, which can find the shortest path between two nodes in the graph. BFS is widely used in many applications, such as finding the shortest path in a maze, web crawlers, etc. This article will introduce how to use Java language to implement the BFS algorithm, and attach specific code examples. First, we need to define a class for storing graph nodes. This class contains nodes
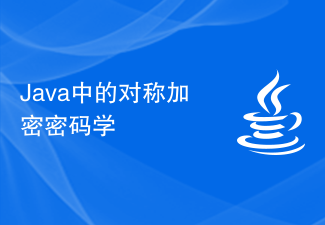
IntroductionSymmetric encryption, also known as key encryption, is an encryption method in which the same key is used for encryption and decryption. This encryption method is fast and efficient and suitable for encrypting large amounts of data. The most commonly used symmetric encryption algorithm is Advanced Encryption Standard (AES). Java provides strong support for symmetric encryption, including classes in the javax.crypto package, such as SecretKey, Cipher, and KeyGenerator. Symmetric encryption in Java The JavaCipher class in the javax.crypto package provides cryptographic functions for encryption and decryption. It forms the core of the Java Cryptozoology Extensions (JCE) framework. In Java, the Cipher class provides symmetric encryption functions, and K
