Flask error handling skills
Flask is a popular Python web framework, and its flexibility and extensibility make it the preferred framework for many people. When developing web applications, you may encounter many problems, such as request errors, server errors, unhandled exceptions, etc. In this post, we’ll explore how to use Flask’s error handling techniques to handle these issues.
- Application level error handling
In a Flask application, we can use the decorator @app.errorhandler()
to handle the application level error. @app.errorhandler()
Accepts a parameter indicating the type of error to be handled. For example, we can add the following code to the application to handle 500 errors:
@app.errorhandler(500) def handle_500_error(error): return "Sorry, there was a server error.", 500
When a 500 error occurs in the application, Flask will call the handle_500_error()
function to handle the error, and returns an HTTP response.
- Blueprints level error handling
In Flask, Blueprint is an architecture that organizes view functions, templates and static files together. If we need to handle errors in a certain Blueprint, we can use the same trick, that is, use the errorhandler()
decorator.
from flask import Blueprint, jsonify bp = Blueprint('api', __name__, url_prefix='/api') @bp.errorhandler(404) def handle_404_error(error): return jsonify({'error': 'Not found'}), 404
In the above example, when some requests apply to Blueprint api
, but the requested resource does not exist or is unavailable, Flask will call handle_404_error()
Returns a 404 HTTP response.
- Use abort() to handle errors
When we want to handle errors in the view function, we can use the abort()
function to help We immediately abort the action, throw a specific error and return the specified error message.
from flask import abort @app.route('/user/<id>') def get_user(id): user = User.query.get(id) if not user: abort(404, description="User does not exist") return render_template('user.html', user=user)
In the above example, we check whether the user with the specified id exists. If not, the abort(404)
function will throw a 404 error, abort the action, and return a 404 error page to the user. Custom error messages can be passed using the description
parameter.
- Custom error page
Finally, we can handle errors that occur during the request by customizing the error page. Flask provides a simple method to specify an error page:
@app.errorhandler(404) def not_found_error(error): return render_template('404.html'), 404
In the above example, we defined a 404
error handling function to present the user with a specified 404.html
page. In this page, you can add custom information, such as prompting the user that the page they are looking for does not exist, recommending some similar pages or websites, and providing links back to other pages, etc.
In Flask, error handling is a very important topic. By using the above tips, you can better handle request errors and provide a better user experience. So, please don’t ignore error handling!
The above is the detailed content of Flask error handling skills. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


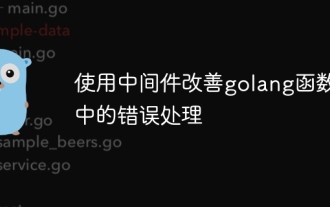
Use middleware to improve error handling in Go functions: Introducing the concept of middleware, which can intercept function calls and execute specific logic. Create error handling middleware that wraps error handling logic in a custom function. Use middleware to wrap handler functions so that error handling logic is performed before the function is called. Returns the appropriate error code based on the error type, улучшениеобработкиошибоквфункциях Goспомощьюпромежуточногопрограммногообеспечения.Оно позволяетнамсосредоточитьсянаобработкеошибо
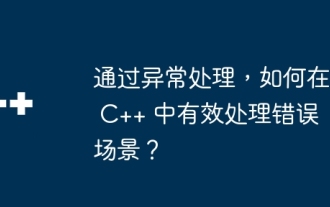
In C++, exception handling handles errors gracefully through try-catch blocks. Common exception types include runtime errors, logic errors, and out-of-bounds errors. Take file opening error handling as an example. When the program fails to open a file, it will throw an exception and print the error message and return the error code through the catch block, thereby handling the error without terminating the program. Exception handling provides advantages such as centralization of error handling, error propagation, and code robustness.
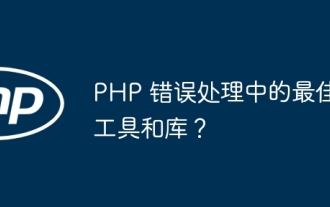
The best error handling tools and libraries in PHP include: Built-in methods: set_error_handler() and error_get_last() Third-party toolkits: Whoops (debugging and error formatting) Third-party services: Sentry (error reporting and monitoring) Third-party libraries: PHP-error-handler (custom error logging and stack traces) and Monolog (error logging handler)
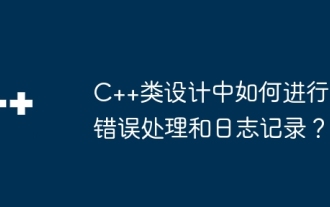
Error handling and logging in C++ class design include: Exception handling: catching and handling exceptions, using custom exception classes to provide specific error information. Error code: Use an integer or enumeration to represent the error condition and return it in the return value. Assertion: Verify pre- and post-conditions, and throw an exception if they are not met. C++ library logging: basic logging using std::cerr and std::clog. External logging libraries: Integrate third-party libraries for advanced features such as level filtering and log file rotation. Custom log class: Create your own log class, abstract the underlying mechanism, and provide a common interface to record different levels of information.
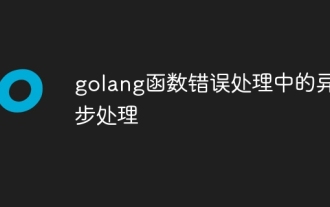
In Go functions, asynchronous error handling uses error channels to asynchronously pass errors from goroutines. The specific steps are as follows: Create an error channel. Start a goroutine to perform operations and send errors asynchronously. Use a select statement to receive errors from the channel. Handle errors asynchronously, such as printing or logging error messages. This approach improves the performance and scalability of concurrent code because error handling does not block the calling thread and execution can be canceled.
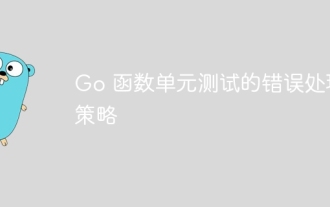
In Go function unit testing, there are two main strategies for error handling: 1. Represent the error as a specific value of the error type, which is used to assert the expected value; 2. Use channels to pass errors to the test function, which is suitable for testing concurrent code. In a practical case, the error value strategy is used to ensure that the function returns 0 for negative input.
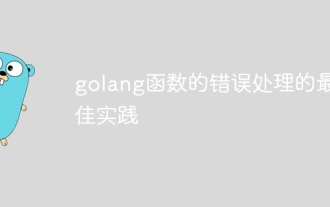
Best practices for error handling in Go include: using the error type, always returning an error, checking for errors, using multi-value returns, using sentinel errors, and using error wrappers. Practical example: In the HTTP request handler, if ReadDataFromDatabase returns an error, return a 500 error response.
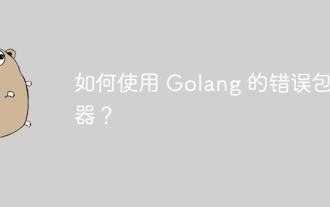
In Golang, error wrappers allow you to create new errors by appending contextual information to the original error. This can be used to unify the types of errors thrown by different libraries or components, simplifying debugging and error handling. The steps are as follows: Use the errors.Wrap function to wrap the original errors into new errors. The new error contains contextual information from the original error. Use fmt.Printf to output wrapped errors, providing more context and actionability. When handling different types of errors, use the errors.Wrap function to unify the error types.
