Using AWS SDK in Go: A Complete Guide
AWS (Amazon Web Services) is a leading global cloud computing provider, providing various cloud computing services to enterprises and individuals. With the development of cloud computing technology, more and more developers are using AWS to develop, test and deploy their applications.
Go language is a very popular programming language, especially suitable for building high-performance and scalable cloud-native applications. AWS provides an SDK (Software Development Kit) for the Go language, which allows developers to easily use various AWS services in Go.
In this article, we will provide you with a complete guide on using AWS SDK in Go language. We will cover how to install and configure the AWS SDK in Go and how to use the SDK to consume AWS services.
- Installing AWS SDK
Installing AWS SDK in Go language is very simple. You only need to execute the following command in the terminal:
go get -u github.com/aws/aws-sdk-go
This command will automatically download and install the AWS SDK. Make sure you have the Go language installed before executing this command.
- Configure AWS SDK
Before using the AWS SDK, you need to create an IAM user on the AWS console and generate an access key. This will allow you to use AWS services.
After you obtain the access key, you need to configure the AWS SDK in your Go language code to use the access key. You can complete the configuration by:
import ( "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/aws/credentials" "github.com/aws/aws-sdk-go/aws/session" ) // 创建一个新的Session sess := session.New(&aws.Config{ Region: aws.String("YOUR_REGION"), // 替换为您的区域 Credentials: credentials.NewStaticCredentials("YOUR_ACCESS_KEY_ID", "YOUR_SECRET_ACCESS_KEY", ""), }) // 创建一个新的AWS服务客户端 svc := ec2.New(sess) // 替换为您的服务
In the sample code above, you need to replace YOUR_REGION, YOUR_ACCESS_KEY_ID, and YOUR_SECRET_ACCESS_KEY with your AWS Region, Access Key ID, and Access Key.
- Using AWS SDK
Now you are ready to use AWS SDK in Go language. Below are some of the most commonly used services in the AWS SDK and examples of their usage.
3.1 Amazon S3
Amazon S3 (Simple Storage Service) is a scalable object storage service that can store and retrieve any type and amount of data. The following is a sample code for uploading files using Amazon S3 in Go language:
import ( "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/service/s3" ) // 创建一个新的S3服务客户端 svc := s3.New(sess) // 使用上面的Session // 上传文件到S3 _, err := svc.PutObject(&s3.PutObjectInput{ Bucket: aws.String("YOUR_BUCKET_NAME"), // 替换为您的桶名 Key: aws.String("YOUR_OBJECT_KEY"), // 替换为您的对象 Body: bytes.NewReader(fileBytes), // 文件的字节码 })
3.2 Amazon SQS
Amazon SQS (Simple Queue Service) is a scalable message queue service that can Distributed, loosely coupled applications. The following is a sample code for sending messages using Amazon SQS in Go language:
import ( "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/service/sqs" ) // 创建一个新的SQS服务客户端 svc := sqs.New(sess) // 使用上面的Session // 发送消息到SQS _, err := svc.SendMessage(&sqs.SendMessageInput{ QueueUrl: aws.String("YOUR_QUEUE_URL"), // 替换为您的队列URL MessageBody: aws.String("YOUR_MESSAGE"), // 替换为您的消息 })
3.3 Amazon DynamoDB
Amazon DynamoDB is a fully managed NoSQL database service that can provide Scalability and performance. The following is the sample code to get items using Amazon DynamoDB in Go language:
import ( "github.com/aws/aws-sdk-go/aws" "github.com/aws/aws-sdk-go/service/dynamodb" ) // 创建一个新的DynamoDB服务客户端 svc := dynamodb.New(sess) // 使用上面的Session // 获取DynamoDB项目 result, err := svc.GetItem(&dynamodb.GetItemInput{ TableName: aws.String("YOUR_TABLE_NAME"), // 替换为您的表名 Key: map[string]*dynamodb.AttributeValue{ "YOUR_PRIMARY_KEY": { S: aws.String("YOUR_PRIMARY_KEY_VALUE"), // 替换为您的主键值 }, }, })
- Conclusion
In this article, we discussed how to use AWS SDK. We cover the installation and configuration process of the SDK, as well as discussing how to use some common AWS services, such as Amazon S3, Amazon SQS, and Amazon DynamoDB.
AWS provides a very powerful cloud computing platform, and the Go language is a powerful and simple programming language. Using the AWS SDK, you can easily use various AWS services in the Go language, improving efficiency and implementing better applications.
The above is the detailed content of Using AWS SDK in Go: A Complete Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


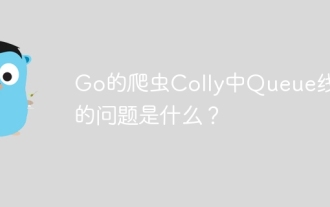
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
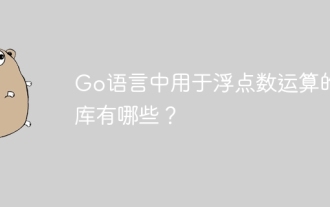
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
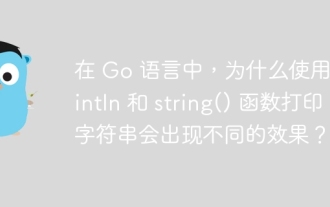
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
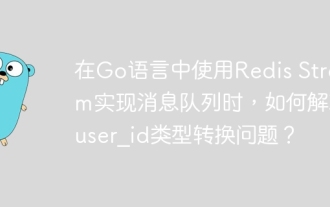
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
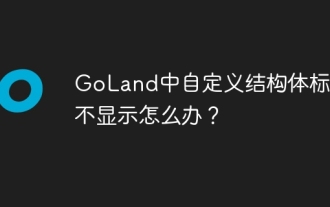
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
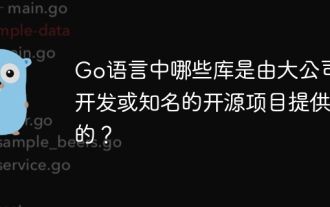
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
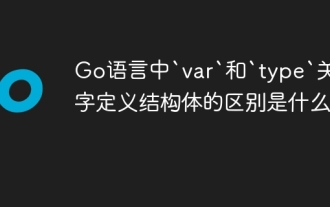
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
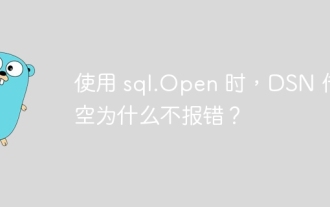
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
