Java backend development: API calls using Retrofit
Java back-end development: Using Retrofit for API calls
With the rapid development of Internet technology, API has become a standard protocol for communication between applications and services, and is widely used in various scenarios. , such as mobile application and website development. In the field of Java back-end development, Retrofit is currently a very popular framework for implementing API calls. This article will introduce what Retrofit is and how to use Retrofit to make API calls.
1. What is Retrofit
Retrofit is a framework based on Java that implements server-side API calls. It uses annotations to describe HTTP requests, parameters and response bodies, and uses Java interfaces to Implement server-side API calls. It uses OkHttp as the underlying network request library, supports synchronous and asynchronous network request methods, and provides a large number of auxiliary functions, such as request retry, request caching, file upload, etc. Retrofit also supports a variety of data converters, such as Gson, Jackson, Moshi, etc., which can easily convert request and response bodies into Java objects.
2. How to use Retrofit to make API calls
1. Import dependencies
To use Retrofit to make API calls, you first need to add relevant dependencies to the project. In the Maven project, you can add the following dependencies in the pom.xml file:
<dependency> <groupId>com.squareup.retrofit2</groupId> <artifactId>retrofit</artifactId> <version>2.9.0</version> </dependency> <dependency> <groupId>com.squareup.retrofit2</groupId> <artifactId>converter-gson</artifactId> <version>2.9.0</version> </dependency>
Among them, retrofit is a dependency of the Retrofit framework itself, and converter-gson is a dependency of Retrofit's Gson data converter.
2. Create API interface
When using Retrofit to make API calls, you need to create the corresponding API interface first. The interface describes the URL, request method, request parameters, return data type and other information of the server API. For example, the following code defines a basic interface for sending a GET request to the server and returning a string:
public interface ApiService { @GET("/api/hello") Call<String> getHello(); }
In this interface, the @GET annotation provided by Retrofit is used to describe HTTP Request type and URL address, use Call
3. Create a Retrofit object
After the interface is defined, you need to use Retrofit to create the corresponding service instance. When creating a Retrofit object, you can specify the request URL, data converter, network request library and other related properties. For example, the following code creates a Retrofit instance and specifies the request URL, Gson data converter and OkHttp network request library:
Retrofit retrofit = new Retrofit.Builder() .baseUrl("http://localhost:8080") .addConverterFactory(GsonConverterFactory.create()) .client(new OkHttpClient.Builder().build()) .build();
Among them, baseUrl specifies the base URL address of the server, and addConverterFactory specifies the data conversion The converter is GsonConverter, and the client specifies the use of OkHttp as the underlying network request library. The default configuration of OkHttpClient is used here, but you can also configure related parameters yourself, such as connection timeout, read and write timeout, etc.
4. Create an API instance
Retrofit creates the implementation class of the API interface through dynamic proxy, making API calls very simple. For example, the following code creates an API instance and calls the getHello method:
ApiService apiService = retrofit.create(ApiService.class); Call<String> call = apiService.getHello(); Response<String> response = call.execute(); System.out.println(response.body());
In this code, an ApiService implementation class is dynamically generated through the retrofit.create method, and the getHello method is used to obtain the Call Object, and finally call the execute method of Call to execute the request synchronously. The execute method will return a Response object, which contains all the information returned by the server, in which the body attribute is the data returned by the server.
If you want to execute the request asynchronously, you can use Call's enqueue method. For example:
ApiService apiService = retrofit.create(ApiService.class); Call<String> call = apiService.getHello(); call.enqueue(new Callback<String>() { @Override public void onResponse(Call<String> call, Response<String> response) { System.out.println(response.body()); } @Override public void onFailure(Call<String> call, Throwable t) { t.printStackTrace(); } });
In this code, Call's enqueue method is used to asynchronously execute the request, and a Callback interface is implemented to process the request results. The onResponse method will be called when the request is successful, and the onFailure method will be called when the request fails.
3. Summary
This article introduces the basic usage of Retrofit, including the process of creating API interfaces, creating Retrofit instances, creating API instances and performing network requests. Retrofit simplifies API calls by using annotations, making it very convenient for the front-end and back-end to interact with data. You need to pay attention to thread safety issues when using Retrofit, because Retrofit is not thread-safe and requires proper synchronization in a multi-threaded environment.
The above is the detailed content of Java backend development: API calls using Retrofit. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


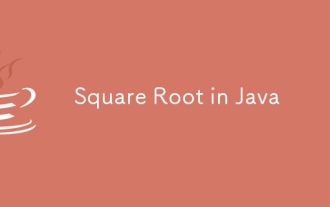
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
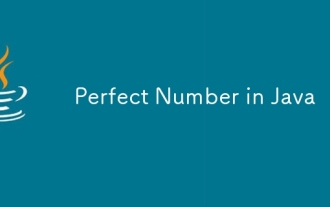
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
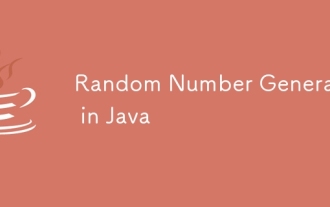
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
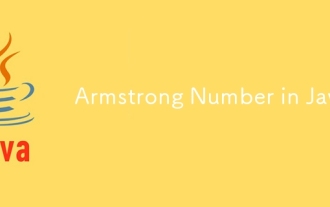
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
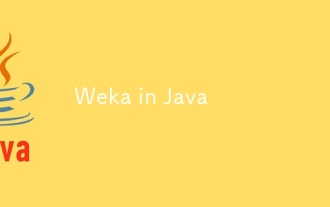
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
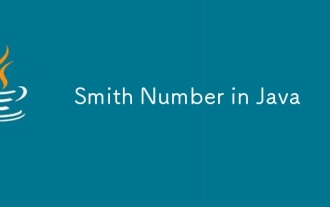
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
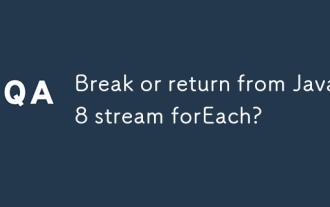
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
