Using AWS CloudWatch in Go: A Complete Guide
AWS CloudWatch is a monitoring, log management, and metric collection service that helps you understand the performance and health of your applications, systems, and services. As a full-featured service provided by AWS, AWS CloudWatch can help users monitor and manage AWS resources, as well as the monitorability of applications and services.
Using AWS CloudWatch in Go, you can easily monitor your applications and resolve performance issues as soon as they are discovered. This article will introduce a complete guide to using AWS CloudWatch in Go language.
- Configuring AWS SDK
Before starting to use AWS CloudWatch, we need to set up the AWS SDK in the Go language. The AWS SDK provides the functionality needed to communicate and authenticate with services. You can install the AWS SDK in Go using the following command:
go get -u github.com/aws/aws-sdk-go/aws
- Verify AWS Account
Before enabling AWS CloudWatch, we need to authenticate the AWS account using AWS credentials. You can set AWS credentials in Go using the following command:
sess, err := session.NewSession(&aws.Config{
Region: aws.String("us-west-2"),
Credentials: credentials.NewStaticCredentials("ACCESS_KEY_ID", "SECRET_ACCESS_KEY", "TOKEN"),
})
In the above example, ACCESS_KEY_ID and SECRET_ACCESS_KEY are the access keys for your AWS account and key. The TOKEN parameter is a temporary security credential generated by the AWS server.
- Create CloudWatch Client
Now we can create a CloudWatch client and start using AWS CloudWatch functionality. You can create a CloudWatch client in Go using the following command:
svc := cloudwatch.New(sess)
In the above example, we use the New function from the existing AWS SDK A new CloudWatch client is created in the session.
- Send Metric Data
Now we are ready to start using AWS CloudWatch functionality. We can use the PutMetricData function to send metric data to AWS CloudWatch. You can send metric data in Go using the following command:
input := &cloudwatch.PutMetricDataInput{
MetricData: []*cloudwatch.MetricDatum{
&cloudwatch.MetricDatum{ MetricName: aws.String("PageViews"), Dimensions: []*cloudwatch.Dimension{ &cloudwatch.Dimension{ Name: aws.String("Page"), Value: aws.String("SiteA"), }, }, Unit: aws.String("Count"), Value: aws.Float64(1.0), },
},
Namespace: aws.String("Site/PageViews"),
}
_, err := svc.PutMetricData(input)
In the above example, we are in the PutMetricDataInput structure The indicator name, indicator dimensions and measurement units are defined. After that, we call the PutMetricData function and pass the input as parameter.
- Create a metric data filter
AWS CloudWatch also provides metric data filters to help you filter and retrieve metric data. You can create a metric data filter in Go using the following command:
input := &cloudwatch.GetMetricDataInput{
MetricDataQueries: []*cloudwatch.MetricDataQuery{
&cloudwatch.MetricDataQuery{ Id: aws.String("m1"), MetricStat: &cloudwatch.MetricStat{}, ReturnData: aws.Bool(true), },
},
StartTime: aws.Time(time.Now().Add(-time.Hour)),
EndTime: aws.Time(time.Now()),
}
_ , err := svc.GetMetricData(input)
In the above example, we use the GetMetricData function and the GetMetricDataInput structure to retrieve the metric data. We can define the query ID and query results, and we can also define the query time range.
- Create CloudWatch Alarms
AWS CloudWatch also supports event alarms, which are triggered when AWS resources reach predetermined thresholds. You can create a CloudWatch alarm in Go using the following command:
input := &cloudwatch.PutMetricAlarmInput{
AlarmName: aws.String("High Load Average"),
ComparisonOperator: aws.String ("GreaterThanOrEqualToThreshold"),
EvaluationPeriods: aws.Int64(3),
MetricName: aws.String("LoadAverage"),
Namespace: aws.String("AWS/EC2"),
Period: aws.Int64(60),
Threshold: aws.Float64(1.0),
AlarmActions: []*string{
aws.String("arn:aws:sns:us-west-2:5466498xxxx:OpsAlert"),
},
}
_, err := svc.PutMetricAlarm(input)
In the above example, we defined the alarm name, comparison operator, evaluation period, metric name, metric namespace, statistical period and alarm threshold. We also define the alert action and set it as the ARN of the SNS topic.
Summary
AWS CloudWatch is a powerful monitoring, log management, and metric collection service. Using the AWS SDK in Go, you can easily integrate with AWS CloudWatch and start monitoring the performance and health of your applications and services. By using AWS CloudWatch, you can immediately identify performance issues and take appropriate actions to resolve them, ensuring the stability and availability of your applications and services.
The above is the detailed content of Using AWS CloudWatch in Go: A Complete Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


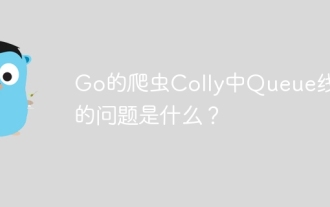
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
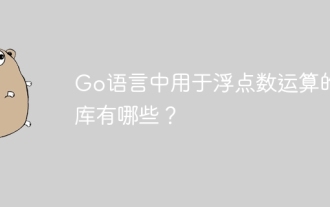
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
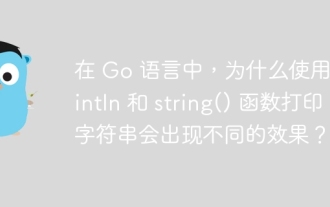
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
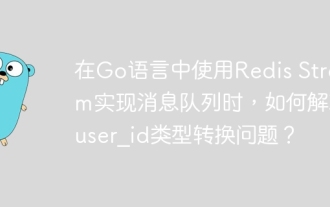
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
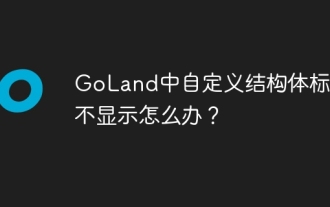
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
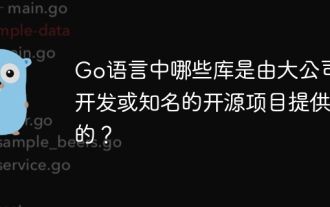
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
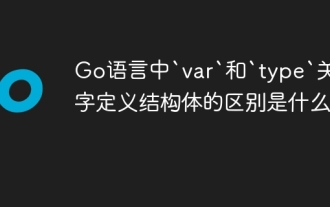
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
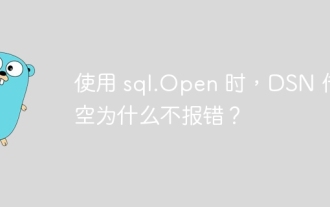
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
