How to use XML for API response in PHP
Jun 17, 2023 pm 02:11 PMWith the development of Internet technology, API interfaces are becoming more and more widely used, and XML is also widely used as a format for data transmission. Using XML for API responses in PHP is a common implementation method. In this article, we'll cover how to use XML for API responses in PHP.
- Determine the API return format
Before we start writing PHP programs, we need to determine the API return format. Usually, the data format returned by an API needs to contain the following content:
- Returned status code
- Returned message, usually in a user-friendly text format
- Returned Data, such as JSON or XML format
- Create an XML document
In PHP, we can use the DOMDocument class to create an XML document. The following is a simple XML document:
<?xml version="1.0"?> <response> <status>200</status> <message>API 请求成功</message> </response>
The above XML document contains two elements: status code and message. In PHP, we can create a DOMDocument object using the following code:
$xml = new DOMDocument('1.0', 'UTF-8');
- Creating XML elements
Next, we need to add elements to the XML document. In our case, we need to add status and message elements. The following is an example of PHP code:
// 创建根元素 $response = $xml->createElement('response'); // 添加 status 元素 $status = $xml->createElement('status', '200'); $response->appendChild($status); // 添加 message 元素 $message = $xml->createElement('message', 'API 请求成功'); $response->appendChild($message); $xml->appendChild($response);
The above code creates a root element named response and adds child elements to it that contain the status code and message.
- Output XML response
The final step is to send the XML response back to the client. In PHP, we can use the following code to output an XML response:
header('Content-type: text/xml'); echo $xml->saveXML();
The above code will output the XML document to the client.
- Complete PHP program
The following is a complete PHP program example:
<?php // 创建 DOMDocument 对象 $xml = new DOMDocument('1.0', 'UTF-8'); // 创建根元素 $response = $xml->createElement('response'); // 添加 status 元素 $status = $xml->createElement('status', '200'); $response->appendChild($status); // 添加 message 元素 $message = $xml->createElement('message', 'API 请求成功'); $response->appendChild($message); // 把根元素添加到 XML 文档中 $xml->appendChild($response); // 输出 XML 响应 header('Content-type: text/xml'); echo $xml->saveXML();
The above code will output the following XML format response:
<?xml version="1.0"?> <response> <status>200</status> <message>API 请求成功</message> </response>
Summary
Using XML in PHP for API responses is relatively simple for those familiar with XML and PHP programming. It should be noted that before outputting the response, you need to ensure that the XML document has been created and contains all elements. I hope this article helps you understand how to use XML for API responses in PHP.
The above is the detailed content of How to use XML for API response in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
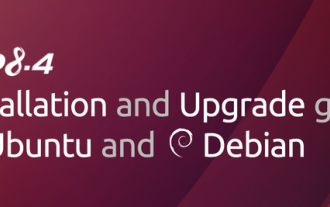
PHP 8.4 Installation and Upgrade guide for Ubuntu and Debian
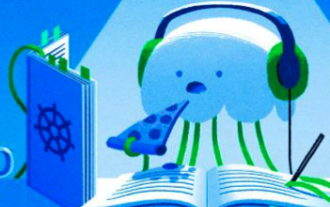
How To Set Up Visual Studio Code (VS Code) for PHP Development
